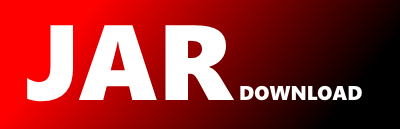
com.aerospike.vector.client.ClientTlsConfig Maven / Gradle / Ivy
Show all versions of avs-client-java Show documentation
package com.aerospike.vector.client;
import io.netty.handler.ssl.SslContext;
import lombok.Getter;
/**
* Configuration class for handling TLS and mTLS within a client application. This class supports
* setting up TLS configurations including custom SSL contexts, specifying allowable TLS protocols,
* and defining the necessary components for mTLS such as root certificates, private keys, and
* certificate chains.
*
* For TLS, only the root certificate is mandatory. For mTLS, the root certificate, private key
* and certificate chain are required.
*/
@Getter
public class ClientTlsConfig {
/**
* The Netty SslContext for creating secure channels. If not specified, then context is created
* using provided tls certs. -- GETTER -- Returns the configured Netty SslContext.
*/
private final SslContext nettySslContext;
/** -- GETTER -- Returns the root certificate path or content. */
private final String rootCertificate;
/** -- GETTER -- Returns the private key path or content, required for mTLS. */
private final String privateKey;
/** -- GETTER -- Returns the certificate chain path or content, required for mTLS. */
private final String certificateChain;
/**
* Constructs a new ClientTlsConfig with specified SSL context, TLS protocols, and certificate
* information.
*
* @param nettySslContext Custom or default Netty SslContext.
* @param protocols Array of strings specifying the allowable TLS protocols.
* @param rootCertificate Path or content of the root certificate.
* @param privateKey Path or content of the private key (required for mTLS).
* @param certificateChain Path or content of the certificate chain (required for mTLS).
*/
public ClientTlsConfig(
SslContext nettySslContext,
String[] protocols,
String rootCertificate,
String privateKey,
String certificateChain) {
this.nettySslContext = nettySslContext;
this.rootCertificate = rootCertificate;
this.privateKey = privateKey;
this.certificateChain = certificateChain;
}
}