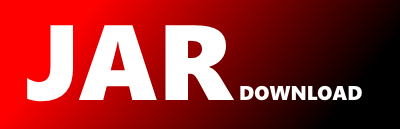
com.aerospike.vector.client.ConnectionConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of avs-client-java Show documentation
Show all versions of avs-client-java Show documentation
This project includes the Java client for Aerospike Vector Search for high-performance data interactions.
The newest version!
package com.aerospike.vector.client;
import com.aerospike.vector.client.auth.Credentials;
import java.util.List;
import lombok.Builder;
import lombok.Getter;
import lombok.ToString;
/**
* Represents the configuration for connecting to an AVS (Aerospike Vector Similarity) server. This
* configuration class encompasses details like connection endpoints, TLS configuration, and
* authentication credentials.
*
* A null {@link ClientTlsConfig} signifies that the connection will not use TLS.
*
*
Example Usage:
*
*
{@code
* ConnectionConfig config = ConnectionConfig.builder()
* .seeds(Arrays.asList(new HostPort("localhost", 3000)))
* .listenerName("myListener")
* .isLoadBalancer(false)
* .clientTlsConfig(null) // or provide a ClientTlsConfig instance
* .credentials(new Credentials("user", "password"))
* .connectTimeout(5000)
* .defaultTimeout(10000)
* .failIfNotConnected(true)
* .build();
* }
*/
@Getter
@ToString
@Builder
public class ConnectionConfig {
/** A list of {@link HostPort} objects specifying the seed nodes for the cluster connection. */
private final List seeds;
/** The name of the listener, if specific configuration is needed. */
private final String listenerName;
/** Indicates whether load balancing is enabled. */
private final boolean isLoadBalancer;
/** The TLS configuration for secure connections, null if not using TLS. */
private final ClientTlsConfig clientTlsConfig;
/** The credentials used for authentication. */
private final Credentials credentials;
/** The timeout in milliseconds for establishing a connection. Defaults to 5000 ms. */
@Builder.Default private int connectTimeout = 5_000;
/**
* The default timeout in milliseconds for operations. Defaults to {@link Integer#MAX_VALUE}.
*/
@Builder.Default private int defaultTimeout = Integer.MAX_VALUE;
/** Flag indicating whether to fail if no connections could be established. */
private final boolean failIfNotConnected;
/**
* Private constructor with validation logic.
*
* @param seeds A list of {@link HostPort} objects specifying the seed nodes for the cluster
* connection.
* @param listenerName The name of the listener, if specific configuration is needed.
* @param isLoadBalancer Indicates whether load balancing is enabled.
* @param clientTlsConfig The TLS configuration for secure connections, null if not using TLS.
* @param credentials The credentials used for authentication.
* @param connectTimeout The timeout in milliseconds for establishing a connection.
* @param defaultTimeout The default timeout in milliseconds for operations.
* @param failIfNotConnected Flag indicating whether to fail if no connections could be
* established.
*/
private ConnectionConfig(
List seeds,
String listenerName,
boolean isLoadBalancer,
ClientTlsConfig clientTlsConfig,
Credentials credentials,
int connectTimeout,
int defaultTimeout,
boolean failIfNotConnected) {
// Parameter validation
if (seeds == null || seeds.isEmpty()) {
throw new IllegalArgumentException("Seed list must not be empty.");
}
if (connectTimeout < 0) {
throw new IllegalArgumentException("Connect timeout must be >= 0.");
}
if (defaultTimeout < 0) {
throw new IllegalArgumentException("Default timeout must be >= 0.");
}
// Assign fields
this.seeds = seeds;
this.listenerName = listenerName;
this.isLoadBalancer = isLoadBalancer;
this.clientTlsConfig = clientTlsConfig;
this.credentials = credentials;
this.connectTimeout = connectTimeout;
this.defaultTimeout = defaultTimeout;
this.failIfNotConnected = failIfNotConnected;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy