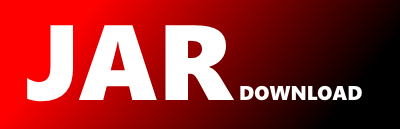
com.aerospike.vector.client.KeySearchQuery Maven / Gradle / Ivy
Show all versions of avs-client-java Show documentation
package com.aerospike.vector.client;
import com.aerospike.vector.client.proto.HnswSearchParams;
import com.aerospike.vector.client.proto.IndexId;
import com.aerospike.vector.client.proto.Vector;
import com.aerospike.vector.client.proto.VectorSearchRequest;
import com.google.common.base.Preconditions;
import lombok.Builder;
import lombok.Getter;
import lombok.NonNull;
import lombok.ToString;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* Represents a search query that uses a specific key in an index. This class should be constructed
* using the {@code KeySearchQuery.builder()}.
*
* The {@code KeySearchQuery} is used to define the parameters for performing a search query in
* an Aerospike vector index using a specific user-defined key.
*
*
Example usage:
*
*
* KeySearchQuery query = KeySearchQuery.builder()
* .namespace("test")
* .indexName("index")
* .limit(10)
* .searchParams(new HnswSearchParams())
* .projection(PROJECT_ALL)
* .timeout(5000)
* .userKey("testKey")
* .build();
*
*
* The class contains several optional parameters such as search parameters, projection, and
* timeout, all of which can be customized using the builder.
*/
@Getter
@ToString
@Builder
public class KeySearchQuery {
private static final Logger log = LoggerFactory.getLogger(KeySearchQuery.class);
/** The namespace in which the index is stored. */
@NonNull private final String namespace;
/** The name of the index to used for searching. */
@NonNull private final String indexName;
/**
* The maximum number of results to return. Defaults to {@link
* VectorSearchQuery#DEFAULT_SEARCH_LIMIT}.
*/
@Builder.Default private int limit = VectorSearchQuery.DEFAULT_SEARCH_LIMIT;
/** The HNSW search parameters used to configure the search behavior. */
private final HnswSearchParams searchParams;
/** The projection of the search results. Defaults to {@link Projection#DEFAULT}. */
@Builder.Default private Projection projection = Projection.DEFAULT;
/**
* The maximum time in milliseconds allowed for the search query. Defaults to {@link
* Integer#MAX_VALUE}.
*/
private final int timeout;
/** The set within the namespace to search (optional). */
private final String set;
/** The user-defined key to be used for the search. */
@NonNull private final Object userKey;
/**
* Converts the current {@code KeySearchQuery} into a {@link VectorSearchRequest}. The
* conversion will use the current state of the query to build a search request, which includes
* the query vector, index details, and optional search parameters.
*
* @param vector The vector used for querying. Must not be {@code null}.
* @return A {@link VectorSearchRequest} that can be used for searching with the given vector.
* @throws IllegalArgumentException if the vector is {@code null}.
*/
public VectorSearchRequest toVectorSearchRequest(Vector vector) {
Preconditions.checkArgument(vector != null, "vector must not be null.");
Preconditions.checkArgument(
namespace != null && !namespace.isEmpty(), "namespace must not be null or empty.");
Preconditions.checkArgument(
indexName != null && !indexName.isEmpty(), "indexName must not be null or empty.");
Preconditions.checkArgument(projection != null, "projection must not be null.");
Preconditions.checkArgument(limit > 0, "limit must be >0.");
VectorSearchRequest.Builder requestBuilder =
VectorSearchRequest.newBuilder()
.setIndex(
IndexId.newBuilder()
.setNamespace(namespace)
.setName(indexName)
.build())
.setQueryVector(vector)
.setLimit(limit)
.setProjection(projection.toProjectionSpec());
if (searchParams != null) {
requestBuilder.setHnswSearchParams(searchParams);
}
return requestBuilder.build();
}
}