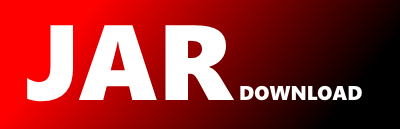
com.aerospike.vector.client.VectorSearchQuery Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of avs-client-java Show documentation
Show all versions of avs-client-java Show documentation
This project includes the Java client for Aerospike Vector Search for high-performance data interactions.
The newest version!
package com.aerospike.vector.client;
import com.aerospike.vector.client.proto.HnswSearchParams;
import com.aerospike.vector.client.proto.IndexId;
import com.aerospike.vector.client.proto.Vector;
import com.aerospike.vector.client.proto.VectorSearchRequest;
import com.google.common.base.Preconditions;
import lombok.Builder;
import lombok.Getter;
import lombok.NonNull;
import lombok.ToString;
/**
* This class encapsulates all necessary parameters required for executing a vector search,
* including namespace, index name, the vector for search, limit on results, search parameters, and
* projection for result filtering.
*/
@Getter
@ToString
@Builder
public class VectorSearchQuery {
public static final int DEFAULT_SEARCH_LIMIT = 5;
/** The namespace of the Aerospike database where the search is to be performed. */
@NonNull private final String namespace;
/** The name of the index within the namespace. */
@NonNull private final String indexName;
/** The vector against which other vectors will be searched. */
@NonNull private final Vector vector;
/** The maximum number of search results to return. */
@Builder.Default private int limit = DEFAULT_SEARCH_LIMIT;
/** Additional search parameters (e.g., algorithm-specific settings). */
private final HnswSearchParams searchParams;
/**
* Projection settings to specify which fields should be included or excluded in the response.
*/
@Builder.Default private Projection projection = Projection.DEFAULT;
/** The HNSW search parameters used to configure the search behavior. */
@Builder.Default private final int timeout = Integer.MAX_VALUE;
/**
* Converts this query object into a VectorSearchRequest.
*
* @return a VectorSearchRequest object that contains all the information of this query.
*/
public VectorSearchRequest toVectorSearchRequest() {
Preconditions.checkArgument(limit > 0, "limit must be >0.");
VectorSearchRequest.Builder requestBuilder =
VectorSearchRequest.newBuilder()
.setIndex(
IndexId.newBuilder()
.setNamespace(namespace)
.setName(indexName)
.build())
.setQueryVector(vector)
.setLimit(limit)
.setProjection(projection.toProjectionSpec());
if (searchParams != null) {
requestBuilder.setHnswSearchParams(searchParams);
}
return requestBuilder.build();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy