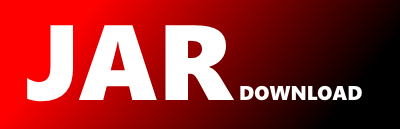
com.aerospike.vector.client.dbclient.IAdminClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of avs-client-java Show documentation
Show all versions of avs-client-java Show documentation
This project includes the Java client for Aerospike Vector Search for high-performance data interactions.
The newest version!
/* (C)2024 */
package com.aerospike.vector.client.dbclient;
import com.aerospike.vector.client.proto.*;
import java.util.List;
import java.util.Map;
import java.util.Set;
import javax.annotation.Nullable;
/**
* The IAdminClient interface provides methods for managing indices within a vector database. It
* extends the Closeable interface, which allows instances of classes implementing this interface to
* be closed in order to release system resources.
*/
public interface IAdminClient {
/**
* Creates a new index for vector search.
*
* @param indexId Unique identifier for the index.
* @param vectorFieldName Name of the bin in the records that contains the vector to index.
* @param dimensions Dimensionality of the indexed vectors.
* @param vectorDistanceMetric Metric used to calculate distance in the vector space.
* @param setFilter Optional filter to only select a subset of the records from a specific set.
* @param indexParams Optional parameters to fine-tune the index configuration.
* @param storage Optional storage parameters for the index, such as persistence settings.
* @param labels Optional user-specific labels to attach to the index for identification or
* categorization.
* @param timeoutInMillis The maximum time in milliseconds that the method will wait for the
* index to be visible.
* @throws RuntimeException if there is a runtime error during index creation.
*/
void indexCreate(
IndexId indexId,
String vectorFieldName,
int dimensions,
VectorDistanceMetric vectorDistanceMetric,
@Nullable String setFilter,
@Nullable HnswParams indexParams,
@Nullable IndexStorage storage,
@Nullable Map labels,
long timeoutInMillis)
throws RuntimeException;
/**
* Update index attributes.
*
* @param indexId unique identifier for the index.
*/
void indexUpdate(
IndexId indexId,
@Nullable Integer maxMemQueueSize,
@Nullable HnswBatchingParams batchingParam,
@Nullable HnswCachingParams cachingParams,
@Nullable HnswHealerParams healerParams,
@Nullable HnswIndexMergeParams mergeParams,
@Nullable Map labels)
throws RuntimeException;
/**
* Deletes an index based on its unique identifier.
*
* @param indexId Unique identifier for the index to be dropped.
* @param timeoutInMillis The maximum time in milliseconds that the method will wait for the
* index to get deleted.
* @throws RuntimeException if there is a runtime error during index deletion.
*/
void indexDrop(IndexId indexId, long timeoutInMillis) throws RuntimeException;
/**
* Garbage collect vertices identified as invalid before cutoff timestamp.
*
* @param indexId the index from which to garbage collect the invalid vertices.
* @param cutoffTimestamp the cutoff timestamp (Unix timestamp) for garbage collecting invalid
* vertices.
*/
void gcInvalidVertices(IndexId indexId, long cutoffTimestamp) throws RuntimeException;
/**
* Lists all indexes in the database.
*
* @param applyDefaults whether to apply default values to parameters which a user does not set.
* @return A list of IndexDefinition objects, each representing an index in the database.
* @throws RuntimeException if there is a runtime error during the retrieval of index
* information.
*/
List indexList(boolean applyDefaults) throws RuntimeException;
/**
* Retrieves the current status of an index.
*
* @param indexId Unique identifier for the index.
* @return An IndexStatusResponse object containing the current status of the index.
* @throws RuntimeException if there is a runtime error while fetching the index status.
*/
IndexStatusResponse indexStatus(IndexId indexId) throws RuntimeException;
/**
* Retrieves the definition of a specific index by its identifier.
*
* @param indexId Unique identifier for the index.
* @return An IndexDefinition object representing the configuration and metadata of the index.
* @throws RuntimeException if there is a runtime error while fetching the index definition.
*/
IndexDefinition getIndex(IndexId indexId, boolean applyDefaults) throws RuntimeException;
/**
* Get information about a user.
*
* @param username the username to fetch the user information for.
* @return the user information.
*/
User getUser(String username);
/**
* Add a user and grant roles.
*
* @param credentials new user credentials.
* @param roles the roles to grant to the new application user.
*/
void addUser(com.aerospike.vector.client.auth.Credentials credentials, Set roles);
/**
* Update user with new credentials.
*
* @param credentials new user credentials.
*/
void updateCredentials(com.aerospike.vector.client.auth.Credentials credentials);
/**
* Drop a user.
*
* @param username the username to drop.
*/
void dropUser(String username);
/**
* List all users.
*
* @return a list of all users
*/
List userList();
/**
* Grant roles to a user.
*
* @param username the username to grant roles to
* @param roles the roles to grant
*/
void grantRoles(String username, Set roles);
/**
* Revoke roles from a user.
*
* @param username the username to revoke roles from
* @param roles the roles to revoke
*/
void revokeRoles(String username, Set roles);
/**
* List all roles.
*
* @return a list of all roles
*/
List roleList();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy