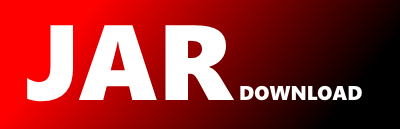
com.aerospike.vector.client.dbclient.IClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of avs-client-java Show documentation
Show all versions of avs-client-java Show documentation
This project includes the Java client for Aerospike Vector Search for high-performance data interactions.
The newest version!
/* (C)2024 */
package com.aerospike.vector.client.dbclient;
import com.aerospike.vector.client.KeySearchQuery;
import com.aerospike.vector.client.Projection;
import com.aerospike.vector.client.VectorSearchQuery;
import com.aerospike.vector.client.proto.*;
import com.google.common.util.concurrent.ListenableFuture;
import java.util.List;
import java.util.Map;
import javax.annotation.Nullable;
import org.checkerframework.checker.nullness.qual.NonNull;
/**
* Interface defining operations for managing vector data in a database. Provides methods to store,
* retrieve, and search vector data, as well as checking existence and indexing status of items in
* the database.
*/
public interface IClient {
/**
* Stores data synchronously in the specified namespace and set.
*
* @param namespace the database namespace.
* @param set the set within the namespace, must not be null.
* @param key the key for the data item.
* @param fields a map of bin names to their respective values.
* @param ignoreMemQueueFull to not throw RESOURCE_EXHAUSTED error on vector record write and
* silently ignore indexing queue full error. If this options is used, the healer becomes
* responsible for indexing this record after it encounters.
* @param writeType {@link WriteType}.
*/
void put(
String namespace,
@NonNull String set,
Object key,
Map fields,
boolean ignoreMemQueueFull,
WriteType writeType);
/**
* Asynchronously stores data in the specified namespace and set. This method initiates the put
* operation and immediately returns a {@link ListenableFuture} that represents the pending
* result of the operation. The future completes when the put operation has successfully
* completed or failed, indicating the outcome.
*
* @param namespace the database namespace where the data will be stored.
* @param set the set within the namespace; must not be null. Represents a subgroup within the
* namespace where the data item will be placed.
* @param key the unique identifier for the data item within the set.
* @param fields a map of bin names to their respective values, representing the data to be
* stored.
* @param ignoreMemQueueFull to not throw RESOURCE_EXHAUSTED error on vector record write and
* silently ignore indexing queue full error. If this options is used, the healer becomes
* responsible for indexing this record after it encounters.
* @param writeType {@link WriteType}.
* @throws IllegalArgumentException if the set is null.
*/
void putAsync(
String namespace,
@NonNull String set,
Object key,
Map fields,
boolean ignoreMemQueueFull,
WriteType writeType);
/**
* Retrieves an object from the database by key, optionally specifying bins to retrieve.
*
* @param namespace the database namespace.
* @param set the set within the namespace, can be null.
* @param key the key for the data item.
* @param projection specifies projections to be included in the result.
* @return a map of bin names to their respective values, null if record was not found.
*/
Map get(
String namespace, @Nullable String set, Object key, Projection projection);
/**
* Delete the record in Aerospike vector database.
*
* @param namespace namespace of the record.
* @param set optionally set of the record.
* @param key record key to be deleted.
*/
void delete(String namespace, @Nullable String set, Object key);
/**
* Checks if an object exists in the database.
*
* @param namespace the database namespace.
* @param set the set within the namespace, can be null.
* @param key the key for the data item.
* @return true if the item exists, otherwise false.
*/
boolean exists(String namespace, @Nullable String set, Object key);
/**
* Check if the given record is indexed in the specified index.
*
* @param namespace record namespace.
* @param set optional record set.
* @param key record key.
* @param indexName name of the index.
* @return if the record is indexed or not.
*/
boolean isIndexed(String namespace, @Nullable String set, Object key, String indexName);
/**
* Waits for the completion of index creation for a specified amount of time.
*
* @param indexId The identifier for the index whose creation completion is to be awaited.
* @param timeoutMillis The maximum time, in milliseconds, to wait for index creation to
* complete.
*/
void waitForIndexCompletion(IndexId indexId, long timeoutMillis);
/**
* Performs a synchronous search for vectors similar to the specified query vector. This method
* executes the search and waits for the operation to complete before returning the results. It
* returns a list of {@link Neighbor} objects that represent the vectors most similar to the
* specified query vector.
*
* @param query The query vector to use as the basis for the search.
* @return a list of {@link Neighbor} objects representing the search results, sorted by their
* similarity to the query vector.
*/
List vectorSearch(VectorSearchQuery query);
/**
* Performs an asynchronous search for vectors similar to the specified query vector. This
* method sends the search request and returns immediately, providing results asynchronously to
* the specified listener.
*
* @param query The query vector to use as the basis for the search.
* @param listener The listener that handles the results or any errors encountered during the
* search.
*/
void vectorSearchAsync(VectorSearchQuery query, VectorSearchListener listener);
/**
* Performs a synchronous search for vectors using given KeySearchQuery. {@link KeySearchQuery}
*
* @param query KeySearchQuery object.
* @param vectorBin field name which contains vector information.
* @return list of neighbors based retrieved based on the query search criteria.
*/
List searchByKey(KeySearchQuery query, String vectorBin);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy