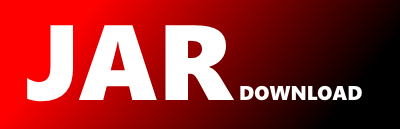
com.aerospike.vector.client.proto.TransactServiceGrpc Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of avs-client-java Show documentation
Show all versions of avs-client-java Show documentation
This project includes the Java client for Aerospike Vector Search for high-performance data interactions.
The newest version!
package com.aerospike.vector.client.proto;
import static io.grpc.MethodDescriptor.generateFullMethodName;
/**
*
* Record transaction services.
*
*/
@javax.annotation.Generated(
value = "by gRPC proto compiler (version 1.68.1)",
comments = "Source: transact.proto")
@io.grpc.stub.annotations.GrpcGenerated
public final class TransactServiceGrpc {
private TransactServiceGrpc() {}
public static final java.lang.String SERVICE_NAME = "aerospike.vector.TransactService";
// Static method descriptors that strictly reflect the proto.
private static volatile io.grpc.MethodDescriptor getPutMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "Put",
requestType = com.aerospike.vector.client.proto.PutRequest.class,
responseType = com.google.protobuf.Empty.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getPutMethod() {
io.grpc.MethodDescriptor getPutMethod;
if ((getPutMethod = TransactServiceGrpc.getPutMethod) == null) {
synchronized (TransactServiceGrpc.class) {
if ((getPutMethod = TransactServiceGrpc.getPutMethod) == null) {
TransactServiceGrpc.getPutMethod = getPutMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "Put"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.aerospike.vector.client.proto.PutRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.google.protobuf.Empty.getDefaultInstance()))
.setSchemaDescriptor(new TransactServiceMethodDescriptorSupplier("Put"))
.build();
}
}
}
return getPutMethod;
}
private static volatile io.grpc.MethodDescriptor getGetMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "Get",
requestType = com.aerospike.vector.client.proto.GetRequest.class,
responseType = com.aerospike.vector.client.proto.Record.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getGetMethod() {
io.grpc.MethodDescriptor getGetMethod;
if ((getGetMethod = TransactServiceGrpc.getGetMethod) == null) {
synchronized (TransactServiceGrpc.class) {
if ((getGetMethod = TransactServiceGrpc.getGetMethod) == null) {
TransactServiceGrpc.getGetMethod = getGetMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "Get"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.aerospike.vector.client.proto.GetRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.aerospike.vector.client.proto.Record.getDefaultInstance()))
.setSchemaDescriptor(new TransactServiceMethodDescriptorSupplier("Get"))
.build();
}
}
}
return getGetMethod;
}
private static volatile io.grpc.MethodDescriptor getDeleteMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "Delete",
requestType = com.aerospike.vector.client.proto.DeleteRequest.class,
responseType = com.google.protobuf.Empty.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getDeleteMethod() {
io.grpc.MethodDescriptor getDeleteMethod;
if ((getDeleteMethod = TransactServiceGrpc.getDeleteMethod) == null) {
synchronized (TransactServiceGrpc.class) {
if ((getDeleteMethod = TransactServiceGrpc.getDeleteMethod) == null) {
TransactServiceGrpc.getDeleteMethod = getDeleteMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "Delete"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.aerospike.vector.client.proto.DeleteRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.google.protobuf.Empty.getDefaultInstance()))
.setSchemaDescriptor(new TransactServiceMethodDescriptorSupplier("Delete"))
.build();
}
}
}
return getDeleteMethod;
}
private static volatile io.grpc.MethodDescriptor getExistsMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "Exists",
requestType = com.aerospike.vector.client.proto.ExistsRequest.class,
responseType = com.aerospike.vector.client.proto.Boolean.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getExistsMethod() {
io.grpc.MethodDescriptor getExistsMethod;
if ((getExistsMethod = TransactServiceGrpc.getExistsMethod) == null) {
synchronized (TransactServiceGrpc.class) {
if ((getExistsMethod = TransactServiceGrpc.getExistsMethod) == null) {
TransactServiceGrpc.getExistsMethod = getExistsMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "Exists"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.aerospike.vector.client.proto.ExistsRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.aerospike.vector.client.proto.Boolean.getDefaultInstance()))
.setSchemaDescriptor(new TransactServiceMethodDescriptorSupplier("Exists"))
.build();
}
}
}
return getExistsMethod;
}
private static volatile io.grpc.MethodDescriptor getIsIndexedMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "IsIndexed",
requestType = com.aerospike.vector.client.proto.IsIndexedRequest.class,
responseType = com.aerospike.vector.client.proto.Boolean.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getIsIndexedMethod() {
io.grpc.MethodDescriptor getIsIndexedMethod;
if ((getIsIndexedMethod = TransactServiceGrpc.getIsIndexedMethod) == null) {
synchronized (TransactServiceGrpc.class) {
if ((getIsIndexedMethod = TransactServiceGrpc.getIsIndexedMethod) == null) {
TransactServiceGrpc.getIsIndexedMethod = getIsIndexedMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "IsIndexed"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.aerospike.vector.client.proto.IsIndexedRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.aerospike.vector.client.proto.Boolean.getDefaultInstance()))
.setSchemaDescriptor(new TransactServiceMethodDescriptorSupplier("IsIndexed"))
.build();
}
}
}
return getIsIndexedMethod;
}
private static volatile io.grpc.MethodDescriptor getVectorSearchMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "VectorSearch",
requestType = com.aerospike.vector.client.proto.VectorSearchRequest.class,
responseType = com.aerospike.vector.client.proto.Neighbor.class,
methodType = io.grpc.MethodDescriptor.MethodType.SERVER_STREAMING)
public static io.grpc.MethodDescriptor getVectorSearchMethod() {
io.grpc.MethodDescriptor getVectorSearchMethod;
if ((getVectorSearchMethod = TransactServiceGrpc.getVectorSearchMethod) == null) {
synchronized (TransactServiceGrpc.class) {
if ((getVectorSearchMethod = TransactServiceGrpc.getVectorSearchMethod) == null) {
TransactServiceGrpc.getVectorSearchMethod = getVectorSearchMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.SERVER_STREAMING)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "VectorSearch"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.aerospike.vector.client.proto.VectorSearchRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.aerospike.vector.client.proto.Neighbor.getDefaultInstance()))
.setSchemaDescriptor(new TransactServiceMethodDescriptorSupplier("VectorSearch"))
.build();
}
}
}
return getVectorSearchMethod;
}
/**
* Creates a new async stub that supports all call types for the service
*/
public static TransactServiceStub newStub(io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public TransactServiceStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new TransactServiceStub(channel, callOptions);
}
};
return TransactServiceStub.newStub(factory, channel);
}
/**
* Creates a new blocking-style stub that supports unary and streaming output calls on the service
*/
public static TransactServiceBlockingStub newBlockingStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public TransactServiceBlockingStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new TransactServiceBlockingStub(channel, callOptions);
}
};
return TransactServiceBlockingStub.newStub(factory, channel);
}
/**
* Creates a new ListenableFuture-style stub that supports unary calls on the service
*/
public static TransactServiceFutureStub newFutureStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public TransactServiceFutureStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new TransactServiceFutureStub(channel, callOptions);
}
};
return TransactServiceFutureStub.newStub(factory, channel);
}
/**
*
* Record transaction services.
*
*/
public interface AsyncService {
/**
*
* Update/insert records.
*
*/
default void put(com.aerospike.vector.client.proto.PutRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getPutMethod(), responseObserver);
}
/**
*
* Get a record.
*
*/
default void get(com.aerospike.vector.client.proto.GetRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getGetMethod(), responseObserver);
}
/**
*
* Delete a record.
*
*/
default void delete(com.aerospike.vector.client.proto.DeleteRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getDeleteMethod(), responseObserver);
}
/**
*
* Check if a record exists.
*
*/
default void exists(com.aerospike.vector.client.proto.ExistsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getExistsMethod(), responseObserver);
}
/**
*
* Check is a record is indexed.
*
*/
default void isIndexed(com.aerospike.vector.client.proto.IsIndexedRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getIsIndexedMethod(), responseObserver);
}
/**
*
* Perform a vector nearest neighbor search.
*
*/
default void vectorSearch(com.aerospike.vector.client.proto.VectorSearchRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getVectorSearchMethod(), responseObserver);
}
}
/**
* Base class for the server implementation of the service TransactService.
*
* Record transaction services.
*
*/
public static abstract class TransactServiceImplBase
implements io.grpc.BindableService, AsyncService {
@java.lang.Override public final io.grpc.ServerServiceDefinition bindService() {
return TransactServiceGrpc.bindService(this);
}
}
/**
* A stub to allow clients to do asynchronous rpc calls to service TransactService.
*
* Record transaction services.
*
*/
public static final class TransactServiceStub
extends io.grpc.stub.AbstractAsyncStub {
private TransactServiceStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected TransactServiceStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new TransactServiceStub(channel, callOptions);
}
/**
*
* Update/insert records.
*
*/
public void put(com.aerospike.vector.client.proto.PutRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getPutMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Get a record.
*
*/
public void get(com.aerospike.vector.client.proto.GetRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getGetMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Delete a record.
*
*/
public void delete(com.aerospike.vector.client.proto.DeleteRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getDeleteMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Check if a record exists.
*
*/
public void exists(com.aerospike.vector.client.proto.ExistsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getExistsMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Check is a record is indexed.
*
*/
public void isIndexed(com.aerospike.vector.client.proto.IsIndexedRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getIsIndexedMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Perform a vector nearest neighbor search.
*
*/
public void vectorSearch(com.aerospike.vector.client.proto.VectorSearchRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncServerStreamingCall(
getChannel().newCall(getVectorSearchMethod(), getCallOptions()), request, responseObserver);
}
}
/**
* A stub to allow clients to do synchronous rpc calls to service TransactService.
*
* Record transaction services.
*
*/
public static final class TransactServiceBlockingStub
extends io.grpc.stub.AbstractBlockingStub {
private TransactServiceBlockingStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected TransactServiceBlockingStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new TransactServiceBlockingStub(channel, callOptions);
}
/**
*
* Update/insert records.
*
*/
public com.google.protobuf.Empty put(com.aerospike.vector.client.proto.PutRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getPutMethod(), getCallOptions(), request);
}
/**
*
* Get a record.
*
*/
public com.aerospike.vector.client.proto.Record get(com.aerospike.vector.client.proto.GetRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getGetMethod(), getCallOptions(), request);
}
/**
*
* Delete a record.
*
*/
public com.google.protobuf.Empty delete(com.aerospike.vector.client.proto.DeleteRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getDeleteMethod(), getCallOptions(), request);
}
/**
*
* Check if a record exists.
*
*/
public com.aerospike.vector.client.proto.Boolean exists(com.aerospike.vector.client.proto.ExistsRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getExistsMethod(), getCallOptions(), request);
}
/**
*
* Check is a record is indexed.
*
*/
public com.aerospike.vector.client.proto.Boolean isIndexed(com.aerospike.vector.client.proto.IsIndexedRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getIsIndexedMethod(), getCallOptions(), request);
}
/**
*
* Perform a vector nearest neighbor search.
*
*/
public java.util.Iterator vectorSearch(
com.aerospike.vector.client.proto.VectorSearchRequest request) {
return io.grpc.stub.ClientCalls.blockingServerStreamingCall(
getChannel(), getVectorSearchMethod(), getCallOptions(), request);
}
}
/**
* A stub to allow clients to do ListenableFuture-style rpc calls to service TransactService.
*
* Record transaction services.
*
*/
public static final class TransactServiceFutureStub
extends io.grpc.stub.AbstractFutureStub {
private TransactServiceFutureStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected TransactServiceFutureStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new TransactServiceFutureStub(channel, callOptions);
}
/**
*
* Update/insert records.
*
*/
public com.google.common.util.concurrent.ListenableFuture put(
com.aerospike.vector.client.proto.PutRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getPutMethod(), getCallOptions()), request);
}
/**
*
* Get a record.
*
*/
public com.google.common.util.concurrent.ListenableFuture get(
com.aerospike.vector.client.proto.GetRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getGetMethod(), getCallOptions()), request);
}
/**
*
* Delete a record.
*
*/
public com.google.common.util.concurrent.ListenableFuture delete(
com.aerospike.vector.client.proto.DeleteRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getDeleteMethod(), getCallOptions()), request);
}
/**
*
* Check if a record exists.
*
*/
public com.google.common.util.concurrent.ListenableFuture exists(
com.aerospike.vector.client.proto.ExistsRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getExistsMethod(), getCallOptions()), request);
}
/**
*
* Check is a record is indexed.
*
*/
public com.google.common.util.concurrent.ListenableFuture isIndexed(
com.aerospike.vector.client.proto.IsIndexedRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getIsIndexedMethod(), getCallOptions()), request);
}
}
private static final int METHODID_PUT = 0;
private static final int METHODID_GET = 1;
private static final int METHODID_DELETE = 2;
private static final int METHODID_EXISTS = 3;
private static final int METHODID_IS_INDEXED = 4;
private static final int METHODID_VECTOR_SEARCH = 5;
private static final class MethodHandlers implements
io.grpc.stub.ServerCalls.UnaryMethod,
io.grpc.stub.ServerCalls.ServerStreamingMethod,
io.grpc.stub.ServerCalls.ClientStreamingMethod,
io.grpc.stub.ServerCalls.BidiStreamingMethod {
private final AsyncService serviceImpl;
private final int methodId;
MethodHandlers(AsyncService serviceImpl, int methodId) {
this.serviceImpl = serviceImpl;
this.methodId = methodId;
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public void invoke(Req request, io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
case METHODID_PUT:
serviceImpl.put((com.aerospike.vector.client.proto.PutRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_GET:
serviceImpl.get((com.aerospike.vector.client.proto.GetRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_DELETE:
serviceImpl.delete((com.aerospike.vector.client.proto.DeleteRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_EXISTS:
serviceImpl.exists((com.aerospike.vector.client.proto.ExistsRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_IS_INDEXED:
serviceImpl.isIndexed((com.aerospike.vector.client.proto.IsIndexedRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_VECTOR_SEARCH:
serviceImpl.vectorSearch((com.aerospike.vector.client.proto.VectorSearchRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
default:
throw new AssertionError();
}
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public io.grpc.stub.StreamObserver invoke(
io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
default:
throw new AssertionError();
}
}
}
public static final io.grpc.ServerServiceDefinition bindService(AsyncService service) {
return io.grpc.ServerServiceDefinition.builder(getServiceDescriptor())
.addMethod(
getPutMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.aerospike.vector.client.proto.PutRequest,
com.google.protobuf.Empty>(
service, METHODID_PUT)))
.addMethod(
getGetMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.aerospike.vector.client.proto.GetRequest,
com.aerospike.vector.client.proto.Record>(
service, METHODID_GET)))
.addMethod(
getDeleteMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.aerospike.vector.client.proto.DeleteRequest,
com.google.protobuf.Empty>(
service, METHODID_DELETE)))
.addMethod(
getExistsMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.aerospike.vector.client.proto.ExistsRequest,
com.aerospike.vector.client.proto.Boolean>(
service, METHODID_EXISTS)))
.addMethod(
getIsIndexedMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.aerospike.vector.client.proto.IsIndexedRequest,
com.aerospike.vector.client.proto.Boolean>(
service, METHODID_IS_INDEXED)))
.addMethod(
getVectorSearchMethod(),
io.grpc.stub.ServerCalls.asyncServerStreamingCall(
new MethodHandlers<
com.aerospike.vector.client.proto.VectorSearchRequest,
com.aerospike.vector.client.proto.Neighbor>(
service, METHODID_VECTOR_SEARCH)))
.build();
}
private static abstract class TransactServiceBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoFileDescriptorSupplier, io.grpc.protobuf.ProtoServiceDescriptorSupplier {
TransactServiceBaseDescriptorSupplier() {}
@java.lang.Override
public com.google.protobuf.Descriptors.FileDescriptor getFileDescriptor() {
return com.aerospike.vector.client.proto.Transact.getDescriptor();
}
@java.lang.Override
public com.google.protobuf.Descriptors.ServiceDescriptor getServiceDescriptor() {
return getFileDescriptor().findServiceByName("TransactService");
}
}
private static final class TransactServiceFileDescriptorSupplier
extends TransactServiceBaseDescriptorSupplier {
TransactServiceFileDescriptorSupplier() {}
}
private static final class TransactServiceMethodDescriptorSupplier
extends TransactServiceBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoMethodDescriptorSupplier {
private final java.lang.String methodName;
TransactServiceMethodDescriptorSupplier(java.lang.String methodName) {
this.methodName = methodName;
}
@java.lang.Override
public com.google.protobuf.Descriptors.MethodDescriptor getMethodDescriptor() {
return getServiceDescriptor().findMethodByName(methodName);
}
}
private static volatile io.grpc.ServiceDescriptor serviceDescriptor;
public static io.grpc.ServiceDescriptor getServiceDescriptor() {
io.grpc.ServiceDescriptor result = serviceDescriptor;
if (result == null) {
synchronized (TransactServiceGrpc.class) {
result = serviceDescriptor;
if (result == null) {
serviceDescriptor = result = io.grpc.ServiceDescriptor.newBuilder(SERVICE_NAME)
.setSchemaDescriptor(new TransactServiceFileDescriptorSupplier())
.addMethod(getPutMethod())
.addMethod(getGetMethod())
.addMethod(getDeleteMethod())
.addMethod(getExistsMethod())
.addMethod(getIsIndexedMethod())
.addMethod(getVectorSearchMethod())
.build();
}
}
}
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy