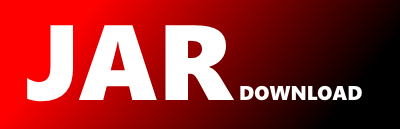
com.aerospike.vector.client.proto.UserAdminServiceGrpc Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of avs-client-java Show documentation
Show all versions of avs-client-java Show documentation
This project includes the Java client for Aerospike Vector Search for high-performance data interactions.
The newest version!
package com.aerospike.vector.client.proto;
import static io.grpc.MethodDescriptor.generateFullMethodName;
/**
*
* User admin service
*
*/
@javax.annotation.Generated(
value = "by gRPC proto compiler (version 1.68.1)",
comments = "Source: user-admin.proto")
@io.grpc.stub.annotations.GrpcGenerated
public final class UserAdminServiceGrpc {
private UserAdminServiceGrpc() {}
public static final java.lang.String SERVICE_NAME = "aerospike.vector.UserAdminService";
// Static method descriptors that strictly reflect the proto.
private static volatile io.grpc.MethodDescriptor getAddUserMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "AddUser",
requestType = com.aerospike.vector.client.proto.AddUserRequest.class,
responseType = com.google.protobuf.Empty.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getAddUserMethod() {
io.grpc.MethodDescriptor getAddUserMethod;
if ((getAddUserMethod = UserAdminServiceGrpc.getAddUserMethod) == null) {
synchronized (UserAdminServiceGrpc.class) {
if ((getAddUserMethod = UserAdminServiceGrpc.getAddUserMethod) == null) {
UserAdminServiceGrpc.getAddUserMethod = getAddUserMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "AddUser"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.aerospike.vector.client.proto.AddUserRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.google.protobuf.Empty.getDefaultInstance()))
.setSchemaDescriptor(new UserAdminServiceMethodDescriptorSupplier("AddUser"))
.build();
}
}
}
return getAddUserMethod;
}
private static volatile io.grpc.MethodDescriptor getUpdateCredentialsMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "UpdateCredentials",
requestType = com.aerospike.vector.client.proto.UpdateCredentialsRequest.class,
responseType = com.google.protobuf.Empty.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getUpdateCredentialsMethod() {
io.grpc.MethodDescriptor getUpdateCredentialsMethod;
if ((getUpdateCredentialsMethod = UserAdminServiceGrpc.getUpdateCredentialsMethod) == null) {
synchronized (UserAdminServiceGrpc.class) {
if ((getUpdateCredentialsMethod = UserAdminServiceGrpc.getUpdateCredentialsMethod) == null) {
UserAdminServiceGrpc.getUpdateCredentialsMethod = getUpdateCredentialsMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "UpdateCredentials"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.aerospike.vector.client.proto.UpdateCredentialsRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.google.protobuf.Empty.getDefaultInstance()))
.setSchemaDescriptor(new UserAdminServiceMethodDescriptorSupplier("UpdateCredentials"))
.build();
}
}
}
return getUpdateCredentialsMethod;
}
private static volatile io.grpc.MethodDescriptor getDropUserMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "DropUser",
requestType = com.aerospike.vector.client.proto.DropUserRequest.class,
responseType = com.google.protobuf.Empty.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getDropUserMethod() {
io.grpc.MethodDescriptor getDropUserMethod;
if ((getDropUserMethod = UserAdminServiceGrpc.getDropUserMethod) == null) {
synchronized (UserAdminServiceGrpc.class) {
if ((getDropUserMethod = UserAdminServiceGrpc.getDropUserMethod) == null) {
UserAdminServiceGrpc.getDropUserMethod = getDropUserMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "DropUser"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.aerospike.vector.client.proto.DropUserRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.google.protobuf.Empty.getDefaultInstance()))
.setSchemaDescriptor(new UserAdminServiceMethodDescriptorSupplier("DropUser"))
.build();
}
}
}
return getDropUserMethod;
}
private static volatile io.grpc.MethodDescriptor getGetUserMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "GetUser",
requestType = com.aerospike.vector.client.proto.GetUserRequest.class,
responseType = com.aerospike.vector.client.proto.User.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getGetUserMethod() {
io.grpc.MethodDescriptor getGetUserMethod;
if ((getGetUserMethod = UserAdminServiceGrpc.getGetUserMethod) == null) {
synchronized (UserAdminServiceGrpc.class) {
if ((getGetUserMethod = UserAdminServiceGrpc.getGetUserMethod) == null) {
UserAdminServiceGrpc.getGetUserMethod = getGetUserMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "GetUser"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.aerospike.vector.client.proto.GetUserRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.aerospike.vector.client.proto.User.getDefaultInstance()))
.setSchemaDescriptor(new UserAdminServiceMethodDescriptorSupplier("GetUser"))
.build();
}
}
}
return getGetUserMethod;
}
private static volatile io.grpc.MethodDescriptor getListUsersMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ListUsers",
requestType = com.google.protobuf.Empty.class,
responseType = com.aerospike.vector.client.proto.ListUsersResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getListUsersMethod() {
io.grpc.MethodDescriptor getListUsersMethod;
if ((getListUsersMethod = UserAdminServiceGrpc.getListUsersMethod) == null) {
synchronized (UserAdminServiceGrpc.class) {
if ((getListUsersMethod = UserAdminServiceGrpc.getListUsersMethod) == null) {
UserAdminServiceGrpc.getListUsersMethod = getListUsersMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ListUsers"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.google.protobuf.Empty.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.aerospike.vector.client.proto.ListUsersResponse.getDefaultInstance()))
.setSchemaDescriptor(new UserAdminServiceMethodDescriptorSupplier("ListUsers"))
.build();
}
}
}
return getListUsersMethod;
}
private static volatile io.grpc.MethodDescriptor getGrantRolesMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "GrantRoles",
requestType = com.aerospike.vector.client.proto.GrantRolesRequest.class,
responseType = com.google.protobuf.Empty.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getGrantRolesMethod() {
io.grpc.MethodDescriptor getGrantRolesMethod;
if ((getGrantRolesMethod = UserAdminServiceGrpc.getGrantRolesMethod) == null) {
synchronized (UserAdminServiceGrpc.class) {
if ((getGrantRolesMethod = UserAdminServiceGrpc.getGrantRolesMethod) == null) {
UserAdminServiceGrpc.getGrantRolesMethod = getGrantRolesMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "GrantRoles"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.aerospike.vector.client.proto.GrantRolesRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.google.protobuf.Empty.getDefaultInstance()))
.setSchemaDescriptor(new UserAdminServiceMethodDescriptorSupplier("GrantRoles"))
.build();
}
}
}
return getGrantRolesMethod;
}
private static volatile io.grpc.MethodDescriptor getRevokeRolesMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "RevokeRoles",
requestType = com.aerospike.vector.client.proto.RevokeRolesRequest.class,
responseType = com.google.protobuf.Empty.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getRevokeRolesMethod() {
io.grpc.MethodDescriptor getRevokeRolesMethod;
if ((getRevokeRolesMethod = UserAdminServiceGrpc.getRevokeRolesMethod) == null) {
synchronized (UserAdminServiceGrpc.class) {
if ((getRevokeRolesMethod = UserAdminServiceGrpc.getRevokeRolesMethod) == null) {
UserAdminServiceGrpc.getRevokeRolesMethod = getRevokeRolesMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "RevokeRoles"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.aerospike.vector.client.proto.RevokeRolesRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.google.protobuf.Empty.getDefaultInstance()))
.setSchemaDescriptor(new UserAdminServiceMethodDescriptorSupplier("RevokeRoles"))
.build();
}
}
}
return getRevokeRolesMethod;
}
private static volatile io.grpc.MethodDescriptor getListRolesMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ListRoles",
requestType = com.google.protobuf.Empty.class,
responseType = com.aerospike.vector.client.proto.ListRolesResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getListRolesMethod() {
io.grpc.MethodDescriptor getListRolesMethod;
if ((getListRolesMethod = UserAdminServiceGrpc.getListRolesMethod) == null) {
synchronized (UserAdminServiceGrpc.class) {
if ((getListRolesMethod = UserAdminServiceGrpc.getListRolesMethod) == null) {
UserAdminServiceGrpc.getListRolesMethod = getListRolesMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ListRoles"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.google.protobuf.Empty.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.aerospike.vector.client.proto.ListRolesResponse.getDefaultInstance()))
.setSchemaDescriptor(new UserAdminServiceMethodDescriptorSupplier("ListRoles"))
.build();
}
}
}
return getListRolesMethod;
}
/**
* Creates a new async stub that supports all call types for the service
*/
public static UserAdminServiceStub newStub(io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public UserAdminServiceStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new UserAdminServiceStub(channel, callOptions);
}
};
return UserAdminServiceStub.newStub(factory, channel);
}
/**
* Creates a new blocking-style stub that supports unary and streaming output calls on the service
*/
public static UserAdminServiceBlockingStub newBlockingStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public UserAdminServiceBlockingStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new UserAdminServiceBlockingStub(channel, callOptions);
}
};
return UserAdminServiceBlockingStub.newStub(factory, channel);
}
/**
* Creates a new ListenableFuture-style stub that supports unary calls on the service
*/
public static UserAdminServiceFutureStub newFutureStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public UserAdminServiceFutureStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new UserAdminServiceFutureStub(channel, callOptions);
}
};
return UserAdminServiceFutureStub.newStub(factory, channel);
}
/**
*
* User admin service
*
*/
public interface AsyncService {
/**
*
* Add a new user.
*
*/
default void addUser(com.aerospike.vector.client.proto.AddUserRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getAddUserMethod(), responseObserver);
}
/**
*
* Update user credentials.
*
*/
default void updateCredentials(com.aerospike.vector.client.proto.UpdateCredentialsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getUpdateCredentialsMethod(), responseObserver);
}
/**
*
* Drop a user.
*
*/
default void dropUser(com.aerospike.vector.client.proto.DropUserRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getDropUserMethod(), responseObserver);
}
/**
*
* Get details for a user.
*
*/
default void getUser(com.aerospike.vector.client.proto.GetUserRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getGetUserMethod(), responseObserver);
}
/**
*
* List users.
*
*/
default void listUsers(com.google.protobuf.Empty request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getListUsersMethod(), responseObserver);
}
/**
*
* Grant roles to a user.
*
*/
default void grantRoles(com.aerospike.vector.client.proto.GrantRolesRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getGrantRolesMethod(), responseObserver);
}
/**
*
* Revoke roles from a user.
*
*/
default void revokeRoles(com.aerospike.vector.client.proto.RevokeRolesRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getRevokeRolesMethod(), responseObserver);
}
/**
*
* List roles.
*
*/
default void listRoles(com.google.protobuf.Empty request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getListRolesMethod(), responseObserver);
}
}
/**
* Base class for the server implementation of the service UserAdminService.
*
* User admin service
*
*/
public static abstract class UserAdminServiceImplBase
implements io.grpc.BindableService, AsyncService {
@java.lang.Override public final io.grpc.ServerServiceDefinition bindService() {
return UserAdminServiceGrpc.bindService(this);
}
}
/**
* A stub to allow clients to do asynchronous rpc calls to service UserAdminService.
*
* User admin service
*
*/
public static final class UserAdminServiceStub
extends io.grpc.stub.AbstractAsyncStub {
private UserAdminServiceStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected UserAdminServiceStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new UserAdminServiceStub(channel, callOptions);
}
/**
*
* Add a new user.
*
*/
public void addUser(com.aerospike.vector.client.proto.AddUserRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getAddUserMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Update user credentials.
*
*/
public void updateCredentials(com.aerospike.vector.client.proto.UpdateCredentialsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getUpdateCredentialsMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Drop a user.
*
*/
public void dropUser(com.aerospike.vector.client.proto.DropUserRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getDropUserMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Get details for a user.
*
*/
public void getUser(com.aerospike.vector.client.proto.GetUserRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getGetUserMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* List users.
*
*/
public void listUsers(com.google.protobuf.Empty request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getListUsersMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Grant roles to a user.
*
*/
public void grantRoles(com.aerospike.vector.client.proto.GrantRolesRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getGrantRolesMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Revoke roles from a user.
*
*/
public void revokeRoles(com.aerospike.vector.client.proto.RevokeRolesRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getRevokeRolesMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* List roles.
*
*/
public void listRoles(com.google.protobuf.Empty request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getListRolesMethod(), getCallOptions()), request, responseObserver);
}
}
/**
* A stub to allow clients to do synchronous rpc calls to service UserAdminService.
*
* User admin service
*
*/
public static final class UserAdminServiceBlockingStub
extends io.grpc.stub.AbstractBlockingStub {
private UserAdminServiceBlockingStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected UserAdminServiceBlockingStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new UserAdminServiceBlockingStub(channel, callOptions);
}
/**
*
* Add a new user.
*
*/
public com.google.protobuf.Empty addUser(com.aerospike.vector.client.proto.AddUserRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getAddUserMethod(), getCallOptions(), request);
}
/**
*
* Update user credentials.
*
*/
public com.google.protobuf.Empty updateCredentials(com.aerospike.vector.client.proto.UpdateCredentialsRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getUpdateCredentialsMethod(), getCallOptions(), request);
}
/**
*
* Drop a user.
*
*/
public com.google.protobuf.Empty dropUser(com.aerospike.vector.client.proto.DropUserRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getDropUserMethod(), getCallOptions(), request);
}
/**
*
* Get details for a user.
*
*/
public com.aerospike.vector.client.proto.User getUser(com.aerospike.vector.client.proto.GetUserRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getGetUserMethod(), getCallOptions(), request);
}
/**
*
* List users.
*
*/
public com.aerospike.vector.client.proto.ListUsersResponse listUsers(com.google.protobuf.Empty request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getListUsersMethod(), getCallOptions(), request);
}
/**
*
* Grant roles to a user.
*
*/
public com.google.protobuf.Empty grantRoles(com.aerospike.vector.client.proto.GrantRolesRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getGrantRolesMethod(), getCallOptions(), request);
}
/**
*
* Revoke roles from a user.
*
*/
public com.google.protobuf.Empty revokeRoles(com.aerospike.vector.client.proto.RevokeRolesRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getRevokeRolesMethod(), getCallOptions(), request);
}
/**
*
* List roles.
*
*/
public com.aerospike.vector.client.proto.ListRolesResponse listRoles(com.google.protobuf.Empty request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getListRolesMethod(), getCallOptions(), request);
}
}
/**
* A stub to allow clients to do ListenableFuture-style rpc calls to service UserAdminService.
*
* User admin service
*
*/
public static final class UserAdminServiceFutureStub
extends io.grpc.stub.AbstractFutureStub {
private UserAdminServiceFutureStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected UserAdminServiceFutureStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new UserAdminServiceFutureStub(channel, callOptions);
}
/**
*
* Add a new user.
*
*/
public com.google.common.util.concurrent.ListenableFuture addUser(
com.aerospike.vector.client.proto.AddUserRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getAddUserMethod(), getCallOptions()), request);
}
/**
*
* Update user credentials.
*
*/
public com.google.common.util.concurrent.ListenableFuture updateCredentials(
com.aerospike.vector.client.proto.UpdateCredentialsRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getUpdateCredentialsMethod(), getCallOptions()), request);
}
/**
*
* Drop a user.
*
*/
public com.google.common.util.concurrent.ListenableFuture dropUser(
com.aerospike.vector.client.proto.DropUserRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getDropUserMethod(), getCallOptions()), request);
}
/**
*
* Get details for a user.
*
*/
public com.google.common.util.concurrent.ListenableFuture getUser(
com.aerospike.vector.client.proto.GetUserRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getGetUserMethod(), getCallOptions()), request);
}
/**
*
* List users.
*
*/
public com.google.common.util.concurrent.ListenableFuture listUsers(
com.google.protobuf.Empty request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getListUsersMethod(), getCallOptions()), request);
}
/**
*
* Grant roles to a user.
*
*/
public com.google.common.util.concurrent.ListenableFuture grantRoles(
com.aerospike.vector.client.proto.GrantRolesRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getGrantRolesMethod(), getCallOptions()), request);
}
/**
*
* Revoke roles from a user.
*
*/
public com.google.common.util.concurrent.ListenableFuture revokeRoles(
com.aerospike.vector.client.proto.RevokeRolesRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getRevokeRolesMethod(), getCallOptions()), request);
}
/**
*
* List roles.
*
*/
public com.google.common.util.concurrent.ListenableFuture listRoles(
com.google.protobuf.Empty request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getListRolesMethod(), getCallOptions()), request);
}
}
private static final int METHODID_ADD_USER = 0;
private static final int METHODID_UPDATE_CREDENTIALS = 1;
private static final int METHODID_DROP_USER = 2;
private static final int METHODID_GET_USER = 3;
private static final int METHODID_LIST_USERS = 4;
private static final int METHODID_GRANT_ROLES = 5;
private static final int METHODID_REVOKE_ROLES = 6;
private static final int METHODID_LIST_ROLES = 7;
private static final class MethodHandlers implements
io.grpc.stub.ServerCalls.UnaryMethod,
io.grpc.stub.ServerCalls.ServerStreamingMethod,
io.grpc.stub.ServerCalls.ClientStreamingMethod,
io.grpc.stub.ServerCalls.BidiStreamingMethod {
private final AsyncService serviceImpl;
private final int methodId;
MethodHandlers(AsyncService serviceImpl, int methodId) {
this.serviceImpl = serviceImpl;
this.methodId = methodId;
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public void invoke(Req request, io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
case METHODID_ADD_USER:
serviceImpl.addUser((com.aerospike.vector.client.proto.AddUserRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_UPDATE_CREDENTIALS:
serviceImpl.updateCredentials((com.aerospike.vector.client.proto.UpdateCredentialsRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_DROP_USER:
serviceImpl.dropUser((com.aerospike.vector.client.proto.DropUserRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_GET_USER:
serviceImpl.getUser((com.aerospike.vector.client.proto.GetUserRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_LIST_USERS:
serviceImpl.listUsers((com.google.protobuf.Empty) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_GRANT_ROLES:
serviceImpl.grantRoles((com.aerospike.vector.client.proto.GrantRolesRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_REVOKE_ROLES:
serviceImpl.revokeRoles((com.aerospike.vector.client.proto.RevokeRolesRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_LIST_ROLES:
serviceImpl.listRoles((com.google.protobuf.Empty) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
default:
throw new AssertionError();
}
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public io.grpc.stub.StreamObserver invoke(
io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
default:
throw new AssertionError();
}
}
}
public static final io.grpc.ServerServiceDefinition bindService(AsyncService service) {
return io.grpc.ServerServiceDefinition.builder(getServiceDescriptor())
.addMethod(
getAddUserMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.aerospike.vector.client.proto.AddUserRequest,
com.google.protobuf.Empty>(
service, METHODID_ADD_USER)))
.addMethod(
getUpdateCredentialsMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.aerospike.vector.client.proto.UpdateCredentialsRequest,
com.google.protobuf.Empty>(
service, METHODID_UPDATE_CREDENTIALS)))
.addMethod(
getDropUserMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.aerospike.vector.client.proto.DropUserRequest,
com.google.protobuf.Empty>(
service, METHODID_DROP_USER)))
.addMethod(
getGetUserMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.aerospike.vector.client.proto.GetUserRequest,
com.aerospike.vector.client.proto.User>(
service, METHODID_GET_USER)))
.addMethod(
getListUsersMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.google.protobuf.Empty,
com.aerospike.vector.client.proto.ListUsersResponse>(
service, METHODID_LIST_USERS)))
.addMethod(
getGrantRolesMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.aerospike.vector.client.proto.GrantRolesRequest,
com.google.protobuf.Empty>(
service, METHODID_GRANT_ROLES)))
.addMethod(
getRevokeRolesMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.aerospike.vector.client.proto.RevokeRolesRequest,
com.google.protobuf.Empty>(
service, METHODID_REVOKE_ROLES)))
.addMethod(
getListRolesMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.google.protobuf.Empty,
com.aerospike.vector.client.proto.ListRolesResponse>(
service, METHODID_LIST_ROLES)))
.build();
}
private static abstract class UserAdminServiceBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoFileDescriptorSupplier, io.grpc.protobuf.ProtoServiceDescriptorSupplier {
UserAdminServiceBaseDescriptorSupplier() {}
@java.lang.Override
public com.google.protobuf.Descriptors.FileDescriptor getFileDescriptor() {
return com.aerospike.vector.client.proto.UserAdmin.getDescriptor();
}
@java.lang.Override
public com.google.protobuf.Descriptors.ServiceDescriptor getServiceDescriptor() {
return getFileDescriptor().findServiceByName("UserAdminService");
}
}
private static final class UserAdminServiceFileDescriptorSupplier
extends UserAdminServiceBaseDescriptorSupplier {
UserAdminServiceFileDescriptorSupplier() {}
}
private static final class UserAdminServiceMethodDescriptorSupplier
extends UserAdminServiceBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoMethodDescriptorSupplier {
private final java.lang.String methodName;
UserAdminServiceMethodDescriptorSupplier(java.lang.String methodName) {
this.methodName = methodName;
}
@java.lang.Override
public com.google.protobuf.Descriptors.MethodDescriptor getMethodDescriptor() {
return getServiceDescriptor().findMethodByName(methodName);
}
}
private static volatile io.grpc.ServiceDescriptor serviceDescriptor;
public static io.grpc.ServiceDescriptor getServiceDescriptor() {
io.grpc.ServiceDescriptor result = serviceDescriptor;
if (result == null) {
synchronized (UserAdminServiceGrpc.class) {
result = serviceDescriptor;
if (result == null) {
serviceDescriptor = result = io.grpc.ServiceDescriptor.newBuilder(SERVICE_NAME)
.setSchemaDescriptor(new UserAdminServiceFileDescriptorSupplier())
.addMethod(getAddUserMethod())
.addMethod(getUpdateCredentialsMethod())
.addMethod(getDropUserMethod())
.addMethod(getGetUserMethod())
.addMethod(getListUsersMethod())
.addMethod(getGrantRolesMethod())
.addMethod(getRevokeRolesMethod())
.addMethod(getListRolesMethod())
.build();
}
}
}
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy