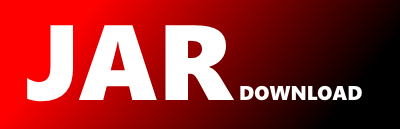
com.afrozaar.wordpress.wpapi.v2.response.PagedResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wp-api-v2-client-java Show documentation
Show all versions of wp-api-v2-client-java Show documentation
A Java client implementation to the WordPress WP-API v2 plugin.
package com.afrozaar.wordpress.wpapi.v2.response;
import com.afrozaar.wordpress.wpapi.v2.Strings;
import com.afrozaar.wordpress.wpapi.v2.request.Request;
import org.springframework.http.HttpHeaders;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.net.URI;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.Function;
public class PagedResponse {
final Class clazz;
public static final Function, String> NEXT = response -> response.getNext().get();
public static final Function, String> PREV = response -> response.getPrevious().get();
private static final Logger LOG = LoggerFactory.getLogger(PagedResponse.class);
// captures the state of a response from wordpress
final String self;
final String next;
final String previous;
final int pages;
final List list;
public PagedResponse(Class clazz, String self, String next, String previous, int pages, List list) {
this.clazz = clazz;
this.self = self;
this.next = next;
this.previous = previous;
this.pages = pages;
this.list = list;
}
public boolean hasNext() {
return Objects.nonNull(next);
}
public Optional getNext() {
return Optional.ofNullable(next);
}
public boolean hasPrevious() {
return Objects.nonNull(previous);
}
public Optional getPrevious() {
return Optional.ofNullable(previous);
}
public String getSelf() {
return self;
}
public List getList() {
return list;
}
public void debug() {
LOG.trace("response.self = {}", this.self);
LOG.trace("response.prev = {}", this.previous);
LOG.trace("response.next = {}", this.next);
LOG.trace("response.pages = {}", this.pages);
LOG.trace("response.list.size = {}", this.list.size());
}
public URI getUri(Function, String> direction) {
return Request.fromLink(direction.apply(this));
}
public Class getClazz() {
return clazz;
}
public static class Builder {
private String next;
// captures the state of a response from wordpress
private String self;
private String previous;
private List posts;
private int pages;
private Class t1;
private Builder(Class t1) {
this.t1 = t1;
}
public static Builder aPagedResponse(Class t) {
return new Builder<>(t);
}
public Builder withNext(Optional next) {
next.ifPresent(n -> this.next = n);
return this;
}
public Builder withSelf(String self) {
this.self = self;
return this;
}
public Builder withPrevious(Optional previous) {
previous.ifPresent(p -> this.previous = p);
return this;
}
public Builder withPosts(List posts) {
this.posts = posts;
return this;
}
public PagedResponse build() {
return new PagedResponse<>(t1, self, next, previous, pages, posts);
}
public Builder withPages(int pages) {
this.pages = pages;
return this;
}
public Builder withPages(HttpHeaders headers) {
headers.get(Strings.HEADER_TOTAL_PAGES).stream()
.findFirst()
.ifPresent(pages -> Builder.this.withPages(Integer.valueOf(pages)));
return this;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy