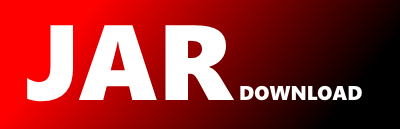
com.agapsys.agreste.test.TestUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of agreste-test Show documentation
Show all versions of agreste-test Show documentation
Testing utilities for AGRESTE-based applications
/*
* Copyright 2016 Agapsys Tecnologia Ltda-ME.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.agapsys.agreste.test;
import com.agapsys.agreste.HttpExchange;
import com.agapsys.http.HttpDelete;
import com.agapsys.http.HttpGet;
import com.agapsys.http.HttpHead;
import com.agapsys.http.HttpOptions;
import com.agapsys.http.HttpRequest;
import com.agapsys.http.HttpResponse.StringResponse;
import com.agapsys.http.HttpTrace;
import com.agapsys.http.StringEntityRequest;
import com.agapsys.http.StringEntityRequest.StringEntityPatch;
import com.agapsys.http.StringEntityRequest.StringEntityPost;
import com.agapsys.http.StringEntityRequest.StringEntityPut;
import com.agapsys.rcf.HttpMethod;
import com.agapsys.rcf.HttpSerializer;
import com.agapsys.rcf.HttpSerializer.SerializerException;
import com.agapsys.rcf.JsonHttpSerializer;
import com.agapsys.utils.console.printer.ConsoleColor;
import com.agapsys.utils.console.printer.ConsolePrinter;
import com.agapsys.web.toolkit.modules.PersistenceModule;
import java.io.IOException;
import java.lang.reflect.Constructor;
import java.lang.reflect.InvocationTargetException;
import java.util.List;
import javax.persistence.EntityManager;
/**
* Testing utilities for AGRESTE-based applications
* @author Leandro Oliveira ([email protected])
*/
public class TestUtils extends com.agapsys.web.toolkit.TestUtils {
// STATIC SCOPE =============================================================
private static TestUtils singleton = null;
public static TestUtils getInstance() {
if (singleton == null)
singleton = new TestUtils();
return singleton;
}
public static class RestEndpoint {
private final TestUtils testUtils = getInstance();
public final HttpMethod method;
public final String uri;
public RestEndpoint(HttpMethod method, String uri) {
if (method == null)
throw new IllegalArgumentException("Null HTTP method");
if (uri == null || uri.trim().isEmpty())
throw new IllegalArgumentException("Null/Empty URI");
this.method = method;
this.uri = uri;
}
public HttpRequest getRequest(String params, Object...paramArgs) {
if (params == null)
params = "";
if (paramArgs.length > 0)
params = String.format(params, paramArgs);
params = params.trim();
String finalUri = params.isEmpty() ? uri : String.format("%s?%s", getUri(), params);
switch (method) {
case DELETE:
return new HttpDelete(finalUri);
case GET:
return new HttpGet(finalUri);
case HEAD:
return new HttpHead(finalUri);
case OPTIONS:
return new HttpOptions(finalUri);
case TRACE:
return new HttpTrace(finalUri);
case PATCH:
return testUtils.createObjectRequest(StringEntityPatch.class, null, finalUri);
case POST:
return testUtils.createObjectRequest(StringEntityPost.class, null, finalUri);
case PUT:
return testUtils.createObjectRequest(StringEntityPut.class, null, finalUri);
default:
throw new UnsupportedOperationException("Unsupported method: " + getMethod().name());
}
}
public final HttpRequest getRequest() {
return getRequest("");
}
public HttpMethod getMethod() {
return method;
}
public String getUri() {
return uri;
}
@Override
public String toString() {
return String.format("%s %s", method.name(), uri);
}
}
public static class EntityRestEndpoint extends RestEndpoint {
private final TestUtils testUtils = getInstance();
private HttpSerializer serializer;
private boolean isEntityMethod(HttpMethod method) {
switch(method) {
case POST:
case PUT:
case PATCH:
return true;
}
return false;
}
public EntityRestEndpoint(HttpMethod method, HttpSerializer serializer, String uri) {
super(method, uri);
if (!isEntityMethod(method))
throw new UnsupportedOperationException("Unsupported method: " + method.name());
if (serializer == null)
throw new IllegalArgumentException("Serializer cannot be null");
this.serializer = serializer;
}
public EntityRestEndpoint(HttpMethod method, String uri) {
this(method, HttpExchange.DEFAULT_SERIALIZER, uri);
}
public HttpSerializer getSerializer() {
return serializer;
}
public HttpRequest getRequest(Object dto, String uriParams, Object...uriParamArgs) {
if (uriParams == null)
uriParams = "";
if (uriParamArgs.length > 0)
uriParams = String.format(uriParams, uriParamArgs);
uriParams = uriParams.trim();
String finalUri = uriParams.isEmpty() ? getUri() : String.format("%s?%s", getUri(), uriParams);
Class extends StringEntityRequest> requestClass;
switch (getMethod()) {
case POST:
requestClass = StringEntityRequest.StringEntityPost.class;
break;
case PUT:
requestClass = StringEntityRequest.StringEntityPut.class;
break;
case PATCH:
requestClass = StringEntityRequest.StringEntityPatch.class;
break;
default:
throw new UnsupportedOperationException("Unsupported method: " + getMethod().name());
}
return testUtils.createObjectRequest(getSerializer(), requestClass, dto, finalUri);
}
public HttpRequest getRequest(Object dto) {
return getRequest(dto, "");
}
}
// =========================================================================
// INSTANCE SCOPE ==========================================================
protected TestUtils() {}
/**
* Creates a {@linkplain StringEntityRequest}
* @param Type of returned request
* @param requestClass {@linkplain StringEntityRequest} subclass
* @param serializer object serializer
* @param obj object to be serialized and added to request
* @param uri request URI
* @param uriParams optional request URI parameters
* @return request containing given object
*/
public T createObjectRequest(HttpSerializer serializer, Class requestClass, Object obj, String uri, Object...uriParams) {
try {
Constructor c = requestClass.getConstructor(String.class, String.class, String.class, Object[].class);
T t = (T) c.newInstance(serializer.getContentType(), serializer.getCharset(), uri, uriParams);
if (obj != null)
t.setContentBody(serializer.toString(obj));
return t;
} catch (NoSuchMethodException | SecurityException | InstantiationException | IllegalAccessException |IllegalArgumentException | InvocationTargetException ex) {
throw new RuntimeException(ex);
}
}
public final T createObjectRequest(Class requestClass, Object obj, String uri, Object...uriParams) {
return createObjectRequest(HttpExchange.DEFAULT_SERIALIZER, requestClass, obj, uri, uriParams);
}
/**
* Read an object from a {@linkplain StringResponse}.
* @param type of object to be read
* @param objClass object class
* @param serializer object serializer used when reading the response
* @param resp server response
* @return read object.
*/
public T readObjectResponse(HttpSerializer serializer, Class objClass, StringResponse resp) {
try {
return (T) serializer.readObject(resp.getContentInputStream(), serializer.getCharset(), objClass);
} catch (IOException | SerializerException ex) {
throw new RuntimeException(ex);
}
}
public final T readObjectResponse(Class objClass, StringResponse resp) {
return readObjectResponse(HttpExchange.DEFAULT_SERIALIZER, objClass, resp);
}
/**
* Reads a JSON list from given response.
* @param List element type
* @param jsonSerializer JSON serializer.
* @param elementClass List element class.
* @param resp server response
* @return JSON list contained in given response.
*/
public List readJsonList(JsonHttpSerializer jsonSerializer, Class elementClass, StringResponse resp) {
try {
return jsonSerializer.getJsonList(resp.getContentInputStream(), "utf-8", elementClass);
} catch (IOException | SerializerException ex) {
throw new RuntimeException(ex);
}
}
/**
* Reads a JSON list from given response.
* @param List element type
* @param elementClass List element class.
* @param resp server response
* @return JSON list contained in given response.
*/
public final List readJsonList(Class elementClass, StringResponse resp) {
return readJsonList((JsonHttpSerializer)HttpExchange.DEFAULT_SERIALIZER, elementClass, resp);
}
@Override
public void println(String msg, Object...msgArgs) {
println(ConsoleColor.MAGENTA, msg, msgArgs);
}
@Override
public void print(String msg, Object...msgArgs) {
print(ConsoleColor.MAGENTA, msg, msgArgs);
}
/**
* Prints a colored message to console.
* @param fgColor foreground color
* @param msg message to be print
* @param msgArgs optional message arguments
*/
public void println(ConsoleColor fgColor, String msg, Object...msgArgs) {
ConsolePrinter.println(fgColor, msg, msgArgs);
}
/**
* Prints a colored message to console.
* @param fgColor foreground color
* @param msg message to be print
* @param msgArgs optional message arguments
*/
public void print(ConsoleColor fgColor, String msg, Object...msgArgs) {
ConsolePrinter.print(fgColor, msg, msgArgs);
}
/**
* Returns an {@linkplain EntityManager} provided by {@linkplain PersistenceModule} registered with running application.
* @return {@linkplain EntityManager} instance. Do not forget to close it after use in order to avoid resource leakage.
*/
public EntityManager getApplicationEntityManager() {
return getApplicationModule(PersistenceModule.class).getEntityManager();
}
// =========================================================================
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy