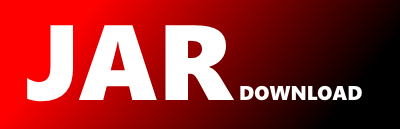
com.agapsys.web.toolkit.AbstractApplication Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of web-app-toolkit-core Show documentation
Show all versions of web-app-toolkit-core Show documentation
Essential tools for back-end applications
The newest version!
/*
* Copyright 2016 Agapsys Tecnologia Ltda-ME.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.agapsys.web.toolkit;
import com.agapsys.web.toolkit.services.LogService;
import com.agapsys.web.toolkit.utils.FileUtils;
import com.agapsys.web.toolkit.utils.FileUtils.AccessError;
import com.agapsys.web.toolkit.utils.SingletonManager;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.math.BigDecimal;
import java.util.Collections;
import java.util.Date;
import java.util.LinkedHashMap;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.NoSuchElementException;
import java.util.Properties;
/**
* Represents an application.
*/
public abstract class AbstractApplication {
//
// =========================================================================
private static interface StringConverter {
public T fromString(String str);
public String toString(T t);
}
private static final Map STRING_CONVERTER_MAP;
static {
Map stringConverterMap = new LinkedHashMap<>();
STRING_CONVERTER_MAP = Collections.unmodifiableMap(stringConverterMap);
stringConverterMap.put(String.class, new StringConverter() {
@Override
public String fromString(String str) {
return str;
}
@Override
public String toString(String str) {
return str;
}
});
stringConverterMap.put(Byte.class, new StringConverter() {
@Override
public Byte fromString(String str) {
return Byte.parseByte(str);
}
@Override
public String toString(Byte b) {
return Byte.toString(b);
}
});
stringConverterMap.put(Short.class, new StringConverter() {
@Override
public Short fromString(String str) {
return Short.parseShort(str);
}
@Override
public String toString(Short s) {
return Short.toString(s);
}
});
stringConverterMap.put(Integer.class, new StringConverter() {
@Override
public Integer fromString(String str) {
return Integer.parseInt(str);
}
@Override
public String toString(Integer i) {
return Integer.toString(i);
}
});
stringConverterMap.put(Long.class, new StringConverter() {
@Override
public Long fromString(String str) {
return Long.parseLong(str);
}
@Override
public String toString(Long l) {
return Long.toString(l);
}
});
stringConverterMap.put(Float.class, new StringConverter() {
@Override
public Float fromString(String str) {
return Float.parseFloat(str);
}
@Override
public String toString(Float f) {
return Float.toString(f);
}
});
stringConverterMap.put(Double.class, new StringConverter() {
@Override
public Double fromString(String str) {
return Double.parseDouble(str);
}
@Override
public String toString(Double d) {
return Double.toString(d);
}
});
stringConverterMap.put(BigDecimal.class, new StringConverter() {
@Override
public BigDecimal fromString(String str) {
return new BigDecimal(str);
}
@Override
public String toString(BigDecimal bd) {
return bd.toPlainString();
}
});
stringConverterMap.put(Date.class, new StringConverter() {
@Override
public Date fromString(String str) {
return new Date(Long.parseLong(str));
}
@Override
public String toString(Date d) {
return Long.toString(d.getTime());
}
});
stringConverterMap.put(Boolean.class, new StringConverter() {
@Override
public Boolean fromString(String str) {
return Boolean.parseBoolean(str);
}
@Override
public String toString(Boolean t) {
return Boolean.toString(t);
}
});
}
private static final String APP_NAME_PATTERN = "^[a-zA-Z][a-zA-Z0-9\\-_]*$";
protected static final String PROPERTIES_FILENAME = "application.properties";
protected static final String LOG_DIR = "log";
private static AbstractApplication runningInstance = null;
private static void __setRunningInstance(AbstractApplication app) {
synchronized(AbstractApplication.class) {
runningInstance = app;
}
}
public static AbstractApplication getRunningInstance() {
synchronized(AbstractApplication.class) {
return runningInstance;
}
}
// =========================================================================
//
private final SingletonManager serviceManager = new SingletonManager<>(Service.class);
private final List initializedServiceList = new LinkedList<>();
private final List> serviceCircularRefCheckList = new LinkedList<>();
private final Properties properties = new Properties();
private volatile boolean settingsLoaded;
private File appDirectory;
private volatile boolean running;
/** Resets instance. */
private synchronized void __reset() {
properties.clear();
serviceManager.clear();
initializedServiceList.clear();
serviceCircularRefCheckList.clear();
settingsLoaded = false;
}
/** Returns the circular reference path ending in given class. */
private synchronized String __getCicularReferencePath(Class extends Service> clazz) {
StringBuilder sb = new StringBuilder();
int i = 0;
for (Class extends Service> serviceClass : serviceCircularRefCheckList) {
if (i > 0)
sb.append(" --> ");
sb.append(serviceClass.getName());
i++;
}
sb.append(" --> ").append(clazz.getName());
return sb.toString();
}
/** Stops initialized services in appropriate sequence. */
private synchronized void __stopServices() {
for (int i = initializedServiceList.size() - 1; i >= 0; i--) {
Service service = initializedServiceList.get(i);
service._stop();
}
}
/** Always returns a non-null instance. */
private synchronized Properties __getDefaultProperties() {
Properties mProperties = getDefaultProperties();
if (mProperties == null)
mProperties = new Properties();
return mProperties;
}
/**
* Loads application properties.
*
* @param createFile define if a default properties file should be created when there is default properties.
* @throws IOException if there was an I/O error while reading/creating properties file.
*/
private synchronized void __loadProperties(boolean createFile) throws IOException {
settingsLoaded = false;
properties.clear();
File propertiesFile = new File(getDirectory(), PROPERTIES_FILENAME);
// Loads properties from file...
if (propertiesFile.exists()) {
try (FileInputStream fis = new FileInputStream(propertiesFile)) {
properties.load(fis);
}
}
// Applies default properties...
for (Map.Entry
© 2015 - 2025 Weber Informatics LLC | Privacy Policy