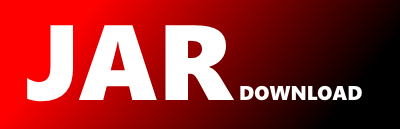
com.agilejava.docbkx.maven.DocbkxTemplateMojo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of docbkx-maven-plugin Show documentation
Show all versions of docbkx-maven-plugin Show documentation
A Maven plugin for generating HTML from DocBook.
The newest version!
package com.agilejava.docbkx.maven;
import javax.xml.transform.URIResolver;
import javax.xml.transform.Transformer;
import org.apache.maven.plugin.MojoExecutionException;
import java.io.File;
import java.util.List;
import java.util.ArrayList;
import java.util.Properties;
import org.apache.maven.project.MavenProject;
import org.apache.tools.ant.Target;
import org.xml.sax.XMLReader;
import org.xml.sax.SAXException;
/**
* A Maven plugin for generating template output from DocBook documents, using version
* 1.79.1 of the DocBook XSL stylesheets. See
* http://docbook.sourceforge.net/.
*
* @goal generate-template
* @configurator override
*/
public class DocbkxTemplateMojo
extends com.agilejava.docbkx.maven.AbstractTransformerMojo
{
/**
* The plugin dependencies.
*
* @parameter property="plugin.artifacts"
* @required
* @readonly
*/
List artifacts;
/**
* Ant tasks to be executed before the transformation. Comparable
* to the tasks property in the maven-antrun-plugin.
*
* @parameter
*/
private Target preProcess;
/**
* Ant tasks to be executed after the transformation. Comparable
* to the tasks property in the maven-antrun-plugin.
*
* @parameter
*/
private Target postProcess;
/**
* @parameter property="project"
* @required
* @readonly
*/
private MavenProject project;
/**
* A list of entities to be substituted in the source
* documents. Note that you can only specify entities if
* your source DocBook document contains a DTD
* declaration. Otherwise it will not have any effect at all.
*
* @parameter
*/
private List entities;
/**
* A list of additional XSL parameters to give to the XSLT engine.
* These parameters overrides regular docbook ones as they are last
* configured.
* For regular docbook parameters perfer the use of this plugin facilities
* offering nammed paramters.
* These parameters feet well for custom properties you may have defined
* within your customization layer.
*
* @parameter
*/
private List customizationParameters = new ArrayList();
/**
* List of additional System properties.
*
* @parameter
*/
private Properties systemProperties;
/**
* The pattern of the files to be included.
*
* @parameter default-value="*.xml"
*/
private String includes;
/**
* A boolean, indicating if XInclude should be supported.
*
* @parameter default="false"
*/
private boolean xincludeSupported;
/**
* The location of the stylesheet customization.
*
* @parameter
*/
private File templateCustomization;
/**
* The extension of the target file name.
*
* @parameter default-value="xsl"
*/
private String targetFileExtension;
/**
* The target directory to which all output will be written.
*
* @parameter default-value="${basedir}/target/docbkx/template"
*/
private File targetDirectory;
/**
* The directory containing the source DocBook files.
*
* @parameter default-value="${basedir}/src/docbkx"
*/
private File sourceDirectory;
/**
* The directory containing the resolved DocBook source before given to the transformer.
*
* @parameter
*/
private File generatedSourceDirectory;
private boolean useStandardOutput = true;
/**
* If the XSLT engine should print xsl:messages to standard output.
*
* @parameter property="docbkx.showXslMessages"
*/
private boolean showXslMessages = false;
/**
* Skip the execution of the plugin.
*
* @parameter property="docbkx.skip" default-value="false"
* @since 2.0.15
*/
private boolean skip;
protected void configure(Transformer transformer) {
getLog().debug("Configure the transformer.");
}
public File getSourceDirectory() {
return sourceDirectory;
}
public File getTargetDirectory() {
return targetDirectory;
}
public File getGeneratedSourceDirectory() {
return generatedSourceDirectory;
}
public String getDefaultStylesheetLocation() {
return "docbook/template/titlepage.xsl";
}
public String getType() {
return "template";
}
public String getStylesheetLocation() {
if (templateCustomization != null) {
int foundOffcet = templateCustomization.toString().indexOf("classpath:");
if(foundOffcet != -1) {
final String withinPath = templateCustomization.toString().substring(foundOffcet + 11);
getLog().debug("User Customization changed to classpath: " + withinPath);
return withinPath;
} else {
getLog().debug("User Customization provided: " + templateCustomization.getAbsolutePath());
return templateCustomization.getAbsolutePath();
}
} else if (getNonDefaultStylesheetLocation() == null) {
getLog().debug("Using default Customization: " + getDefaultStylesheetLocation());
return getDefaultStylesheetLocation();
} else {
getLog().debug("Using non-default Customization: " + getNonDefaultStylesheetLocation());
return getNonDefaultStylesheetLocation();
}
}
public String getTargetFileExtension() {
return targetFileExtension;
}
public void setTargetFileExtension(String extension) {
targetFileExtension = extension;
}
public String[] getIncludes() {
String[] results = includes.split(",");
for (int i = 0; i < results.length; i++) {
results[i] = results[i].trim();
}
return results;
}
public List getEntities() {
return entities;
}
public List getCustomizationParameters()
{
return customizationParameters;
}
public Properties getSystemProperties()
{
return systemProperties;
}
public Target getPreProcess() {
return preProcess;
}
public Target getPostProcess() {
return postProcess;
}
public MavenProject getMavenProject() {
return project;
}
public List getArtifacts() {
return artifacts;
}
protected boolean getXIncludeSupported() {
return xincludeSupported;
}
/**
* Returns false if the stylesheet is responsible to create the output file(s) using its own naming scheme.
*
* @return If using the standard output.
*/
protected boolean isUseStandardOutput() {
return useStandardOutput;
}
protected boolean isShowXslMessages() {
return showXslMessages;
}
protected void setShowXslMessages(boolean showXslMessages) {
this.showXslMessages = showXslMessages;
}
protected void setUseStandardOutput(boolean useStandardOutput) {
this.useStandardOutput = useStandardOutput;
}
protected void setSkip(boolean skip) {
this.skip = skip;
}
protected boolean isSkip() {
return this.skip;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy