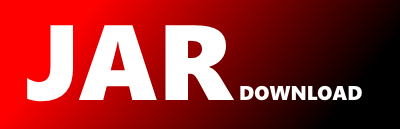
com.ait.tooling.json.parser.JSONParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ahome-tooling-json Show documentation
Show all versions of ahome-tooling-json Show documentation
Ahome Tooling JSON - Java JSON Parser and Serializer, heavily mooded from JSON Simple.
/*
* Copyright (c) 2014,2015 Ahome' Innovation Technologies. All rights reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.ait.tooling.json.parser;
import java.io.IOException;
import java.io.Reader;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import com.ait.tooling.common.server.io.NoSyncStringReader;
import com.ait.tooling.json.JSONArray;
import com.ait.tooling.json.JSONObject;
/**
* Parser for JSON text. Please note that JSONParser is NOT thread-safe.
*
* @author FangYidong
*/
public final class JSONParser
{
public static final int S_INIT = 0;
public static final int S_IN_FINISHED_VALUE = 1; // string,number,boolean,null,object,array
public static final int S_IN_OBJECT = 2;
public static final int S_IN_ARRAY = 3;
public static final int S_PASSED_PAIR_KEY = 4;
public static final int S_IN_PAIR_VALUE = 5;
public static final int S_END = 6;
public static final int S_IN_ERROR = -1;
private Yylex m_lexer = new Yylex((Reader) null);
private Yytoken m_token = null;
private int m_phase = S_INIT;
public JSONParser()
{
}
private int peekStatus(LinkedList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy