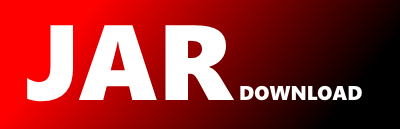
com.airbyte.api.Connections Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of api Show documentation
Show all versions of api Show documentation
SDK enabling Java developers to easily integrate with the Airbyte API.
The newest version!
/*
* Code generated by Speakeasy (https://speakeasyapi.dev). DO NOT EDIT.
*/
package com.airbyte.api;
import com.airbyte.api.models.errors.SDKError;
import com.airbyte.api.models.operations.SDKMethodInterfaces.*;
import com.airbyte.api.utils.HTTPClient;
import com.airbyte.api.utils.HTTPRequest;
import com.airbyte.api.utils.Hook.AfterErrorContextImpl;
import com.airbyte.api.utils.Hook.AfterSuccessContextImpl;
import com.airbyte.api.utils.Hook.BeforeRequestContextImpl;
import com.airbyte.api.utils.JSON;
import com.airbyte.api.utils.Retries.NonRetryableException;
import com.airbyte.api.utils.SerializedBody;
import com.airbyte.api.utils.Utils;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import java.io.InputStream;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
import java.nio.charset.StandardCharsets;
import java.util.Optional;
import org.apache.http.NameValuePair;
import org.openapitools.jackson.nullable.JsonNullable;
public class Connections implements
MethodCallCreateConnection,
MethodCallDeleteConnection,
MethodCallGetConnection,
MethodCallListConnections,
MethodCallPatchConnection {
private final SDKConfiguration sdkConfiguration;
Connections(SDKConfiguration sdkConfiguration) {
this.sdkConfiguration = sdkConfiguration;
}
/**
* Create a connection
* @return The call builder
*/
public com.airbyte.api.models.operations.CreateConnectionRequestBuilder createConnection() {
return new com.airbyte.api.models.operations.CreateConnectionRequestBuilder(this);
}
/**
* Create a connection
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public com.airbyte.api.models.operations.CreateConnectionResponse createConnection(
com.airbyte.api.models.shared.ConnectionCreateRequest request) throws Exception {
String _baseUrl = this.sdkConfiguration.serverUrl;
String _url = Utils.generateURL(
_baseUrl,
"/connections");
HTTPRequest _req = new HTTPRequest(_url, "POST");
Object _convertedRequest = Utils.convertToShape(request, Utils.JsonShape.DEFAULT,
new TypeReference() {});
SerializedBody _serializedRequestBody = Utils.serializeRequestBody(
_convertedRequest, "request", "json", false);
if (_serializedRequestBody == null) {
throw new Exception("Request body is required");
}
_req.setBody(Optional.ofNullable(_serializedRequestBody));
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
this.sdkConfiguration.userAgent);
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl("createConnection", sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "400", "403", "4XX", "5XX")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl("createConnection", sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl("createConnection", sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(new AfterErrorContextImpl("createConnection", sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
com.airbyte.api.models.operations.CreateConnectionResponse.Builder _resBuilder =
com.airbyte.api.models.operations.CreateConnectionResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
com.airbyte.api.models.operations.CreateConnectionResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "200")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
com.airbyte.api.models.shared.ConnectionResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withConnectionResponse(java.util.Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.toByteArrayAndClose(_httpRes.body()));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "400", "403", "4XX", "5XX")) {
// no content
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"API error occurred",
Utils.toByteArrayAndClose(_httpRes.body()));
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.toByteArrayAndClose(_httpRes.body()));
}
/**
* Delete a Connection
* @return The call builder
*/
public com.airbyte.api.models.operations.DeleteConnectionRequestBuilder deleteConnection() {
return new com.airbyte.api.models.operations.DeleteConnectionRequestBuilder(this);
}
/**
* Delete a Connection
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public com.airbyte.api.models.operations.DeleteConnectionResponse deleteConnection(
com.airbyte.api.models.operations.DeleteConnectionRequest request) throws Exception {
String _baseUrl = this.sdkConfiguration.serverUrl;
String _url = Utils.generateURL(
com.airbyte.api.models.operations.DeleteConnectionRequest.class,
_baseUrl,
"/connections/{connectionId}",
request, null);
HTTPRequest _req = new HTTPRequest(_url, "DELETE");
_req.addHeader("Accept", "*/*")
.addHeader("user-agent",
this.sdkConfiguration.userAgent);
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl("deleteConnection", sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "403", "404", "4XX", "5XX")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl("deleteConnection", sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl("deleteConnection", sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(new AfterErrorContextImpl("deleteConnection", sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
com.airbyte.api.models.operations.DeleteConnectionResponse.Builder _resBuilder =
com.airbyte.api.models.operations.DeleteConnectionResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
com.airbyte.api.models.operations.DeleteConnectionResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "204")) {
// no content
return _res;
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "403", "404", "4XX", "5XX")) {
// no content
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"API error occurred",
Utils.toByteArrayAndClose(_httpRes.body()));
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.toByteArrayAndClose(_httpRes.body()));
}
/**
* Get Connection details
* @return The call builder
*/
public com.airbyte.api.models.operations.GetConnectionRequestBuilder getConnection() {
return new com.airbyte.api.models.operations.GetConnectionRequestBuilder(this);
}
/**
* Get Connection details
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public com.airbyte.api.models.operations.GetConnectionResponse getConnection(
com.airbyte.api.models.operations.GetConnectionRequest request) throws Exception {
String _baseUrl = this.sdkConfiguration.serverUrl;
String _url = Utils.generateURL(
com.airbyte.api.models.operations.GetConnectionRequest.class,
_baseUrl,
"/connections/{connectionId}",
request, null);
HTTPRequest _req = new HTTPRequest(_url, "GET");
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
this.sdkConfiguration.userAgent);
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl("getConnection", sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "403", "404", "4XX", "5XX")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl("getConnection", sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl("getConnection", sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(new AfterErrorContextImpl("getConnection", sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
com.airbyte.api.models.operations.GetConnectionResponse.Builder _resBuilder =
com.airbyte.api.models.operations.GetConnectionResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
com.airbyte.api.models.operations.GetConnectionResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "200")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
com.airbyte.api.models.shared.ConnectionResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withConnectionResponse(java.util.Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.toByteArrayAndClose(_httpRes.body()));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "403", "404", "4XX", "5XX")) {
// no content
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"API error occurred",
Utils.toByteArrayAndClose(_httpRes.body()));
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.toByteArrayAndClose(_httpRes.body()));
}
/**
* List connections
* @return The call builder
*/
public com.airbyte.api.models.operations.ListConnectionsRequestBuilder listConnections() {
return new com.airbyte.api.models.operations.ListConnectionsRequestBuilder(this);
}
/**
* List connections
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public com.airbyte.api.models.operations.ListConnectionsResponse listConnections(
com.airbyte.api.models.operations.ListConnectionsRequest request) throws Exception {
String _baseUrl = this.sdkConfiguration.serverUrl;
String _url = Utils.generateURL(
_baseUrl,
"/connections");
HTTPRequest _req = new HTTPRequest(_url, "GET");
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
this.sdkConfiguration.userAgent);
_req.addQueryParams(Utils.getQueryParams(
com.airbyte.api.models.operations.ListConnectionsRequest.class,
request,
null));
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl("listConnections", sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "403", "404", "4XX", "5XX")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl("listConnections", sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl("listConnections", sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(new AfterErrorContextImpl("listConnections", sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
com.airbyte.api.models.operations.ListConnectionsResponse.Builder _resBuilder =
com.airbyte.api.models.operations.ListConnectionsResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
com.airbyte.api.models.operations.ListConnectionsResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "200")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
com.airbyte.api.models.shared.ConnectionsResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withConnectionsResponse(java.util.Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.toByteArrayAndClose(_httpRes.body()));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "403", "404", "4XX", "5XX")) {
// no content
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"API error occurred",
Utils.toByteArrayAndClose(_httpRes.body()));
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.toByteArrayAndClose(_httpRes.body()));
}
/**
* Update Connection details
* @return The call builder
*/
public com.airbyte.api.models.operations.PatchConnectionRequestBuilder patchConnection() {
return new com.airbyte.api.models.operations.PatchConnectionRequestBuilder(this);
}
/**
* Update Connection details
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public com.airbyte.api.models.operations.PatchConnectionResponse patchConnection(
com.airbyte.api.models.operations.PatchConnectionRequest request) throws Exception {
String _baseUrl = this.sdkConfiguration.serverUrl;
String _url = Utils.generateURL(
com.airbyte.api.models.operations.PatchConnectionRequest.class,
_baseUrl,
"/connections/{connectionId}",
request, null);
HTTPRequest _req = new HTTPRequest(_url, "PATCH");
Object _convertedRequest = Utils.convertToShape(request, Utils.JsonShape.DEFAULT,
new TypeReference() {});
SerializedBody _serializedRequestBody = Utils.serializeRequestBody(
_convertedRequest, "connectionPatchRequest", "json", false);
if (_serializedRequestBody == null) {
throw new Exception("Request body is required");
}
_req.setBody(Optional.ofNullable(_serializedRequestBody));
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
this.sdkConfiguration.userAgent);
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl("patchConnection", sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "403", "404", "4XX", "5XX")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl("patchConnection", sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl("patchConnection", sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(new AfterErrorContextImpl("patchConnection", sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
com.airbyte.api.models.operations.PatchConnectionResponse.Builder _resBuilder =
com.airbyte.api.models.operations.PatchConnectionResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
com.airbyte.api.models.operations.PatchConnectionResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "200")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
com.airbyte.api.models.shared.ConnectionResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withConnectionResponse(java.util.Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.toByteArrayAndClose(_httpRes.body()));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "403", "404", "4XX", "5XX")) {
// no content
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"API error occurred",
Utils.toByteArrayAndClose(_httpRes.body()));
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.toByteArrayAndClose(_httpRes.body()));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy