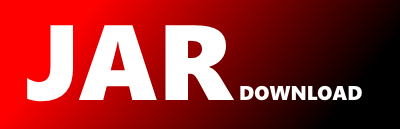
com.airbyte.api.models.shared.AuthenticateViaGoogleOauth Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of api Show documentation
Show all versions of api Show documentation
SDK enabling Java developers to easily integrate with the Airbyte API.
The newest version!
/*
* Code generated by Speakeasy (https://speakeasyapi.dev). DO NOT EDIT.
*/
package com.airbyte.api.models.shared;
import com.airbyte.api.utils.LazySingletonValue;
import com.airbyte.api.utils.Utils;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonFormat;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.core.type.TypeReference;
import java.io.InputStream;
import java.lang.Deprecated;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.util.Optional;
public class AuthenticateViaGoogleOauth {
/**
* Access Token for making authenticated requests.
*/
@JsonInclude(Include.NON_ABSENT)
@JsonProperty("access_token")
private Optional extends String> accessToken;
@JsonInclude(Include.NON_ABSENT)
@JsonProperty("auth_type")
private Optional extends SourceGoogleAnalyticsDataApiAuthType> authType;
/**
* The Client ID of your Google Analytics developer application.
*/
@JsonProperty("client_id")
private String clientId;
/**
* The Client Secret of your Google Analytics developer application.
*/
@JsonProperty("client_secret")
private String clientSecret;
/**
* The token for obtaining a new access token.
*/
@JsonProperty("refresh_token")
private String refreshToken;
@JsonCreator
public AuthenticateViaGoogleOauth(
@JsonProperty("access_token") Optional extends String> accessToken,
@JsonProperty("client_id") String clientId,
@JsonProperty("client_secret") String clientSecret,
@JsonProperty("refresh_token") String refreshToken) {
Utils.checkNotNull(accessToken, "accessToken");
Utils.checkNotNull(clientId, "clientId");
Utils.checkNotNull(clientSecret, "clientSecret");
Utils.checkNotNull(refreshToken, "refreshToken");
this.accessToken = accessToken;
this.authType = Builder._SINGLETON_VALUE_AuthType.value();
this.clientId = clientId;
this.clientSecret = clientSecret;
this.refreshToken = refreshToken;
}
public AuthenticateViaGoogleOauth(
String clientId,
String clientSecret,
String refreshToken) {
this(Optional.empty(), clientId, clientSecret, refreshToken);
}
/**
* Access Token for making authenticated requests.
*/
@SuppressWarnings("unchecked")
@JsonIgnore
public Optional accessToken() {
return (Optional) accessToken;
}
@SuppressWarnings("unchecked")
@JsonIgnore
public Optional authType() {
return (Optional) authType;
}
/**
* The Client ID of your Google Analytics developer application.
*/
@JsonIgnore
public String clientId() {
return clientId;
}
/**
* The Client Secret of your Google Analytics developer application.
*/
@JsonIgnore
public String clientSecret() {
return clientSecret;
}
/**
* The token for obtaining a new access token.
*/
@JsonIgnore
public String refreshToken() {
return refreshToken;
}
public final static Builder builder() {
return new Builder();
}
/**
* Access Token for making authenticated requests.
*/
public AuthenticateViaGoogleOauth withAccessToken(String accessToken) {
Utils.checkNotNull(accessToken, "accessToken");
this.accessToken = Optional.ofNullable(accessToken);
return this;
}
/**
* Access Token for making authenticated requests.
*/
public AuthenticateViaGoogleOauth withAccessToken(Optional extends String> accessToken) {
Utils.checkNotNull(accessToken, "accessToken");
this.accessToken = accessToken;
return this;
}
/**
* The Client ID of your Google Analytics developer application.
*/
public AuthenticateViaGoogleOauth withClientId(String clientId) {
Utils.checkNotNull(clientId, "clientId");
this.clientId = clientId;
return this;
}
/**
* The Client Secret of your Google Analytics developer application.
*/
public AuthenticateViaGoogleOauth withClientSecret(String clientSecret) {
Utils.checkNotNull(clientSecret, "clientSecret");
this.clientSecret = clientSecret;
return this;
}
/**
* The token for obtaining a new access token.
*/
public AuthenticateViaGoogleOauth withRefreshToken(String refreshToken) {
Utils.checkNotNull(refreshToken, "refreshToken");
this.refreshToken = refreshToken;
return this;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
AuthenticateViaGoogleOauth other = (AuthenticateViaGoogleOauth) o;
return
java.util.Objects.deepEquals(this.accessToken, other.accessToken) &&
java.util.Objects.deepEquals(this.authType, other.authType) &&
java.util.Objects.deepEquals(this.clientId, other.clientId) &&
java.util.Objects.deepEquals(this.clientSecret, other.clientSecret) &&
java.util.Objects.deepEquals(this.refreshToken, other.refreshToken);
}
@Override
public int hashCode() {
return java.util.Objects.hash(
accessToken,
authType,
clientId,
clientSecret,
refreshToken);
}
@Override
public String toString() {
return Utils.toString(AuthenticateViaGoogleOauth.class,
"accessToken", accessToken,
"authType", authType,
"clientId", clientId,
"clientSecret", clientSecret,
"refreshToken", refreshToken);
}
public final static class Builder {
private Optional extends String> accessToken = Optional.empty();
private String clientId;
private String clientSecret;
private String refreshToken;
private Builder() {
// force use of static builder() method
}
/**
* Access Token for making authenticated requests.
*/
public Builder accessToken(String accessToken) {
Utils.checkNotNull(accessToken, "accessToken");
this.accessToken = Optional.ofNullable(accessToken);
return this;
}
/**
* Access Token for making authenticated requests.
*/
public Builder accessToken(Optional extends String> accessToken) {
Utils.checkNotNull(accessToken, "accessToken");
this.accessToken = accessToken;
return this;
}
/**
* The Client ID of your Google Analytics developer application.
*/
public Builder clientId(String clientId) {
Utils.checkNotNull(clientId, "clientId");
this.clientId = clientId;
return this;
}
/**
* The Client Secret of your Google Analytics developer application.
*/
public Builder clientSecret(String clientSecret) {
Utils.checkNotNull(clientSecret, "clientSecret");
this.clientSecret = clientSecret;
return this;
}
/**
* The token for obtaining a new access token.
*/
public Builder refreshToken(String refreshToken) {
Utils.checkNotNull(refreshToken, "refreshToken");
this.refreshToken = refreshToken;
return this;
}
public AuthenticateViaGoogleOauth build() {
return new AuthenticateViaGoogleOauth(
accessToken,
clientId,
clientSecret,
refreshToken);
}
private static final LazySingletonValue> _SINGLETON_VALUE_AuthType =
new LazySingletonValue<>(
"auth_type",
"\"Client\"",
new TypeReference>() {});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy