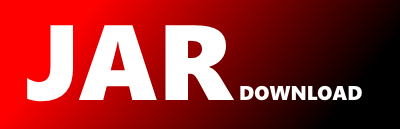
com.airbyte.api.models.shared.DestinationElasticsearch Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of api Show documentation
Show all versions of api Show documentation
SDK enabling Java developers to easily integrate with the Airbyte API.
The newest version!
/*
* Code generated by Speakeasy (https://speakeasyapi.dev). DO NOT EDIT.
*/
package com.airbyte.api.models.shared;
import com.airbyte.api.utils.LazySingletonValue;
import com.airbyte.api.utils.Utils;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonFormat;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.core.type.TypeReference;
import java.io.InputStream;
import java.lang.Deprecated;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.util.Optional;
public class DestinationElasticsearch {
/**
* The type of authentication to be used
*/
@JsonInclude(Include.NON_ABSENT)
@JsonProperty("authenticationMethod")
private Optional extends AuthenticationMethod> authenticationMethod;
/**
* CA certificate
*/
@JsonInclude(Include.NON_ABSENT)
@JsonProperty("ca_certificate")
private Optional extends String> caCertificate;
@JsonProperty("destinationType")
private Elasticsearch destinationType;
/**
* The full url of the Elasticsearch server
*/
@JsonProperty("endpoint")
private String endpoint;
/**
* If a primary key identifier is defined in the source, an upsert will be performed using the primary key value as the elasticsearch doc id. Does not support composite primary keys.
*/
@JsonInclude(Include.NON_ABSENT)
@JsonProperty("upsert")
private Optional extends Boolean> upsert;
@JsonCreator
public DestinationElasticsearch(
@JsonProperty("authenticationMethod") Optional extends AuthenticationMethod> authenticationMethod,
@JsonProperty("ca_certificate") Optional extends String> caCertificate,
@JsonProperty("endpoint") String endpoint,
@JsonProperty("upsert") Optional extends Boolean> upsert) {
Utils.checkNotNull(authenticationMethod, "authenticationMethod");
Utils.checkNotNull(caCertificate, "caCertificate");
Utils.checkNotNull(endpoint, "endpoint");
Utils.checkNotNull(upsert, "upsert");
this.authenticationMethod = authenticationMethod;
this.caCertificate = caCertificate;
this.destinationType = Builder._SINGLETON_VALUE_DestinationType.value();
this.endpoint = endpoint;
this.upsert = upsert;
}
public DestinationElasticsearch(
String endpoint) {
this(Optional.empty(), Optional.empty(), endpoint, Optional.empty());
}
/**
* The type of authentication to be used
*/
@SuppressWarnings("unchecked")
@JsonIgnore
public Optional authenticationMethod() {
return (Optional) authenticationMethod;
}
/**
* CA certificate
*/
@SuppressWarnings("unchecked")
@JsonIgnore
public Optional caCertificate() {
return (Optional) caCertificate;
}
@JsonIgnore
public Elasticsearch destinationType() {
return destinationType;
}
/**
* The full url of the Elasticsearch server
*/
@JsonIgnore
public String endpoint() {
return endpoint;
}
/**
* If a primary key identifier is defined in the source, an upsert will be performed using the primary key value as the elasticsearch doc id. Does not support composite primary keys.
*/
@SuppressWarnings("unchecked")
@JsonIgnore
public Optional upsert() {
return (Optional) upsert;
}
public final static Builder builder() {
return new Builder();
}
/**
* The type of authentication to be used
*/
public DestinationElasticsearch withAuthenticationMethod(AuthenticationMethod authenticationMethod) {
Utils.checkNotNull(authenticationMethod, "authenticationMethod");
this.authenticationMethod = Optional.ofNullable(authenticationMethod);
return this;
}
/**
* The type of authentication to be used
*/
public DestinationElasticsearch withAuthenticationMethod(Optional extends AuthenticationMethod> authenticationMethod) {
Utils.checkNotNull(authenticationMethod, "authenticationMethod");
this.authenticationMethod = authenticationMethod;
return this;
}
/**
* CA certificate
*/
public DestinationElasticsearch withCaCertificate(String caCertificate) {
Utils.checkNotNull(caCertificate, "caCertificate");
this.caCertificate = Optional.ofNullable(caCertificate);
return this;
}
/**
* CA certificate
*/
public DestinationElasticsearch withCaCertificate(Optional extends String> caCertificate) {
Utils.checkNotNull(caCertificate, "caCertificate");
this.caCertificate = caCertificate;
return this;
}
/**
* The full url of the Elasticsearch server
*/
public DestinationElasticsearch withEndpoint(String endpoint) {
Utils.checkNotNull(endpoint, "endpoint");
this.endpoint = endpoint;
return this;
}
/**
* If a primary key identifier is defined in the source, an upsert will be performed using the primary key value as the elasticsearch doc id. Does not support composite primary keys.
*/
public DestinationElasticsearch withUpsert(boolean upsert) {
Utils.checkNotNull(upsert, "upsert");
this.upsert = Optional.ofNullable(upsert);
return this;
}
/**
* If a primary key identifier is defined in the source, an upsert will be performed using the primary key value as the elasticsearch doc id. Does not support composite primary keys.
*/
public DestinationElasticsearch withUpsert(Optional extends Boolean> upsert) {
Utils.checkNotNull(upsert, "upsert");
this.upsert = upsert;
return this;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
DestinationElasticsearch other = (DestinationElasticsearch) o;
return
java.util.Objects.deepEquals(this.authenticationMethod, other.authenticationMethod) &&
java.util.Objects.deepEquals(this.caCertificate, other.caCertificate) &&
java.util.Objects.deepEquals(this.destinationType, other.destinationType) &&
java.util.Objects.deepEquals(this.endpoint, other.endpoint) &&
java.util.Objects.deepEquals(this.upsert, other.upsert);
}
@Override
public int hashCode() {
return java.util.Objects.hash(
authenticationMethod,
caCertificate,
destinationType,
endpoint,
upsert);
}
@Override
public String toString() {
return Utils.toString(DestinationElasticsearch.class,
"authenticationMethod", authenticationMethod,
"caCertificate", caCertificate,
"destinationType", destinationType,
"endpoint", endpoint,
"upsert", upsert);
}
public final static class Builder {
private Optional extends AuthenticationMethod> authenticationMethod = Optional.empty();
private Optional extends String> caCertificate = Optional.empty();
private String endpoint;
private Optional extends Boolean> upsert;
private Builder() {
// force use of static builder() method
}
/**
* The type of authentication to be used
*/
public Builder authenticationMethod(AuthenticationMethod authenticationMethod) {
Utils.checkNotNull(authenticationMethod, "authenticationMethod");
this.authenticationMethod = Optional.ofNullable(authenticationMethod);
return this;
}
/**
* The type of authentication to be used
*/
public Builder authenticationMethod(Optional extends AuthenticationMethod> authenticationMethod) {
Utils.checkNotNull(authenticationMethod, "authenticationMethod");
this.authenticationMethod = authenticationMethod;
return this;
}
/**
* CA certificate
*/
public Builder caCertificate(String caCertificate) {
Utils.checkNotNull(caCertificate, "caCertificate");
this.caCertificate = Optional.ofNullable(caCertificate);
return this;
}
/**
* CA certificate
*/
public Builder caCertificate(Optional extends String> caCertificate) {
Utils.checkNotNull(caCertificate, "caCertificate");
this.caCertificate = caCertificate;
return this;
}
/**
* The full url of the Elasticsearch server
*/
public Builder endpoint(String endpoint) {
Utils.checkNotNull(endpoint, "endpoint");
this.endpoint = endpoint;
return this;
}
/**
* If a primary key identifier is defined in the source, an upsert will be performed using the primary key value as the elasticsearch doc id. Does not support composite primary keys.
*/
public Builder upsert(boolean upsert) {
Utils.checkNotNull(upsert, "upsert");
this.upsert = Optional.ofNullable(upsert);
return this;
}
/**
* If a primary key identifier is defined in the source, an upsert will be performed using the primary key value as the elasticsearch doc id. Does not support composite primary keys.
*/
public Builder upsert(Optional extends Boolean> upsert) {
Utils.checkNotNull(upsert, "upsert");
this.upsert = upsert;
return this;
}
public DestinationElasticsearch build() {
if (upsert == null) {
upsert = _SINGLETON_VALUE_Upsert.value();
}
return new DestinationElasticsearch(
authenticationMethod,
caCertificate,
endpoint,
upsert);
}
private static final LazySingletonValue _SINGLETON_VALUE_DestinationType =
new LazySingletonValue<>(
"destinationType",
"\"elasticsearch\"",
new TypeReference() {});
private static final LazySingletonValue> _SINGLETON_VALUE_Upsert =
new LazySingletonValue<>(
"upsert",
"true",
new TypeReference>() {});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy