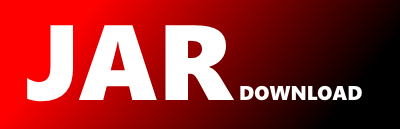
com.airbyte.api.models.shared.GoogleAdsCredentials Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of api Show documentation
Show all versions of api Show documentation
SDK enabling Java developers to easily integrate with the Airbyte API.
The newest version!
/*
* Code generated by Speakeasy (https://speakeasyapi.dev). DO NOT EDIT.
*/
package com.airbyte.api.models.shared;
import com.airbyte.api.utils.Utils;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonFormat;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.core.type.TypeReference;
import java.io.InputStream;
import java.lang.Deprecated;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.util.Optional;
public class GoogleAdsCredentials {
/**
* The Client ID of your Google Ads developer application. For detailed instructions on finding this value, refer to our <a href="https://docs.airbyte.com/integrations/sources/google-ads#setup-guide">documentation</a>.
*/
@JsonInclude(Include.NON_ABSENT)
@JsonProperty("client_id")
private Optional extends String> clientId;
/**
* The Client Secret of your Google Ads developer application. For detailed instructions on finding this value, refer to our <a href="https://docs.airbyte.com/integrations/sources/google-ads#setup-guide">documentation</a>.
*/
@JsonInclude(Include.NON_ABSENT)
@JsonProperty("client_secret")
private Optional extends String> clientSecret;
/**
* The Developer Token granted by Google to use their APIs. For detailed instructions on finding this value, refer to our <a href="https://docs.airbyte.com/integrations/sources/google-ads#setup-guide">documentation</a>.
*/
@JsonInclude(Include.NON_ABSENT)
@JsonProperty("developer_token")
private Optional extends String> developerToken;
@JsonCreator
public GoogleAdsCredentials(
@JsonProperty("client_id") Optional extends String> clientId,
@JsonProperty("client_secret") Optional extends String> clientSecret,
@JsonProperty("developer_token") Optional extends String> developerToken) {
Utils.checkNotNull(clientId, "clientId");
Utils.checkNotNull(clientSecret, "clientSecret");
Utils.checkNotNull(developerToken, "developerToken");
this.clientId = clientId;
this.clientSecret = clientSecret;
this.developerToken = developerToken;
}
public GoogleAdsCredentials() {
this(Optional.empty(), Optional.empty(), Optional.empty());
}
/**
* The Client ID of your Google Ads developer application. For detailed instructions on finding this value, refer to our <a href="https://docs.airbyte.com/integrations/sources/google-ads#setup-guide">documentation</a>.
*/
@SuppressWarnings("unchecked")
@JsonIgnore
public Optional clientId() {
return (Optional) clientId;
}
/**
* The Client Secret of your Google Ads developer application. For detailed instructions on finding this value, refer to our <a href="https://docs.airbyte.com/integrations/sources/google-ads#setup-guide">documentation</a>.
*/
@SuppressWarnings("unchecked")
@JsonIgnore
public Optional clientSecret() {
return (Optional) clientSecret;
}
/**
* The Developer Token granted by Google to use their APIs. For detailed instructions on finding this value, refer to our <a href="https://docs.airbyte.com/integrations/sources/google-ads#setup-guide">documentation</a>.
*/
@SuppressWarnings("unchecked")
@JsonIgnore
public Optional developerToken() {
return (Optional) developerToken;
}
public final static Builder builder() {
return new Builder();
}
/**
* The Client ID of your Google Ads developer application. For detailed instructions on finding this value, refer to our <a href="https://docs.airbyte.com/integrations/sources/google-ads#setup-guide">documentation</a>.
*/
public GoogleAdsCredentials withClientId(String clientId) {
Utils.checkNotNull(clientId, "clientId");
this.clientId = Optional.ofNullable(clientId);
return this;
}
/**
* The Client ID of your Google Ads developer application. For detailed instructions on finding this value, refer to our <a href="https://docs.airbyte.com/integrations/sources/google-ads#setup-guide">documentation</a>.
*/
public GoogleAdsCredentials withClientId(Optional extends String> clientId) {
Utils.checkNotNull(clientId, "clientId");
this.clientId = clientId;
return this;
}
/**
* The Client Secret of your Google Ads developer application. For detailed instructions on finding this value, refer to our <a href="https://docs.airbyte.com/integrations/sources/google-ads#setup-guide">documentation</a>.
*/
public GoogleAdsCredentials withClientSecret(String clientSecret) {
Utils.checkNotNull(clientSecret, "clientSecret");
this.clientSecret = Optional.ofNullable(clientSecret);
return this;
}
/**
* The Client Secret of your Google Ads developer application. For detailed instructions on finding this value, refer to our <a href="https://docs.airbyte.com/integrations/sources/google-ads#setup-guide">documentation</a>.
*/
public GoogleAdsCredentials withClientSecret(Optional extends String> clientSecret) {
Utils.checkNotNull(clientSecret, "clientSecret");
this.clientSecret = clientSecret;
return this;
}
/**
* The Developer Token granted by Google to use their APIs. For detailed instructions on finding this value, refer to our <a href="https://docs.airbyte.com/integrations/sources/google-ads#setup-guide">documentation</a>.
*/
public GoogleAdsCredentials withDeveloperToken(String developerToken) {
Utils.checkNotNull(developerToken, "developerToken");
this.developerToken = Optional.ofNullable(developerToken);
return this;
}
/**
* The Developer Token granted by Google to use their APIs. For detailed instructions on finding this value, refer to our <a href="https://docs.airbyte.com/integrations/sources/google-ads#setup-guide">documentation</a>.
*/
public GoogleAdsCredentials withDeveloperToken(Optional extends String> developerToken) {
Utils.checkNotNull(developerToken, "developerToken");
this.developerToken = developerToken;
return this;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
GoogleAdsCredentials other = (GoogleAdsCredentials) o;
return
java.util.Objects.deepEquals(this.clientId, other.clientId) &&
java.util.Objects.deepEquals(this.clientSecret, other.clientSecret) &&
java.util.Objects.deepEquals(this.developerToken, other.developerToken);
}
@Override
public int hashCode() {
return java.util.Objects.hash(
clientId,
clientSecret,
developerToken);
}
@Override
public String toString() {
return Utils.toString(GoogleAdsCredentials.class,
"clientId", clientId,
"clientSecret", clientSecret,
"developerToken", developerToken);
}
public final static class Builder {
private Optional extends String> clientId = Optional.empty();
private Optional extends String> clientSecret = Optional.empty();
private Optional extends String> developerToken = Optional.empty();
private Builder() {
// force use of static builder() method
}
/**
* The Client ID of your Google Ads developer application. For detailed instructions on finding this value, refer to our <a href="https://docs.airbyte.com/integrations/sources/google-ads#setup-guide">documentation</a>.
*/
public Builder clientId(String clientId) {
Utils.checkNotNull(clientId, "clientId");
this.clientId = Optional.ofNullable(clientId);
return this;
}
/**
* The Client ID of your Google Ads developer application. For detailed instructions on finding this value, refer to our <a href="https://docs.airbyte.com/integrations/sources/google-ads#setup-guide">documentation</a>.
*/
public Builder clientId(Optional extends String> clientId) {
Utils.checkNotNull(clientId, "clientId");
this.clientId = clientId;
return this;
}
/**
* The Client Secret of your Google Ads developer application. For detailed instructions on finding this value, refer to our <a href="https://docs.airbyte.com/integrations/sources/google-ads#setup-guide">documentation</a>.
*/
public Builder clientSecret(String clientSecret) {
Utils.checkNotNull(clientSecret, "clientSecret");
this.clientSecret = Optional.ofNullable(clientSecret);
return this;
}
/**
* The Client Secret of your Google Ads developer application. For detailed instructions on finding this value, refer to our <a href="https://docs.airbyte.com/integrations/sources/google-ads#setup-guide">documentation</a>.
*/
public Builder clientSecret(Optional extends String> clientSecret) {
Utils.checkNotNull(clientSecret, "clientSecret");
this.clientSecret = clientSecret;
return this;
}
/**
* The Developer Token granted by Google to use their APIs. For detailed instructions on finding this value, refer to our <a href="https://docs.airbyte.com/integrations/sources/google-ads#setup-guide">documentation</a>.
*/
public Builder developerToken(String developerToken) {
Utils.checkNotNull(developerToken, "developerToken");
this.developerToken = Optional.ofNullable(developerToken);
return this;
}
/**
* The Developer Token granted by Google to use their APIs. For detailed instructions on finding this value, refer to our <a href="https://docs.airbyte.com/integrations/sources/google-ads#setup-guide">documentation</a>.
*/
public Builder developerToken(Optional extends String> developerToken) {
Utils.checkNotNull(developerToken, "developerToken");
this.developerToken = developerToken;
return this;
}
public GoogleAdsCredentials build() {
return new GoogleAdsCredentials(
clientId,
clientSecret,
developerToken);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy