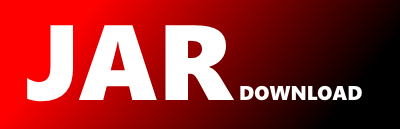
com.airbyte.api.models.shared.PersonalAccessToken Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of api Show documentation
Show all versions of api Show documentation
SDK enabling Java developers to easily integrate with the Airbyte API.
The newest version!
/*
* Code generated by Speakeasy (https://speakeasyapi.dev). DO NOT EDIT.
*/
package com.airbyte.api.models.shared;
import com.airbyte.api.utils.LazySingletonValue;
import com.airbyte.api.utils.Utils;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonFormat;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.core.type.TypeReference;
import java.io.InputStream;
import java.lang.Deprecated;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.util.Optional;
public class PersonalAccessToken {
/**
* The Personal Access Token for the Airtable account. See the <a href="https://airtable.com/developers/web/guides/personal-access-tokens">Support Guide</a> for more information on how to obtain this token.
*/
@JsonProperty("api_key")
private String apiKey;
@JsonInclude(Include.NON_ABSENT)
@JsonProperty("auth_method")
private Optional extends SourceAirtableAuthMethod> authMethod;
@JsonCreator
public PersonalAccessToken(
@JsonProperty("api_key") String apiKey) {
Utils.checkNotNull(apiKey, "apiKey");
this.apiKey = apiKey;
this.authMethod = Builder._SINGLETON_VALUE_AuthMethod.value();
}
/**
* The Personal Access Token for the Airtable account. See the <a href="https://airtable.com/developers/web/guides/personal-access-tokens">Support Guide</a> for more information on how to obtain this token.
*/
@JsonIgnore
public String apiKey() {
return apiKey;
}
@SuppressWarnings("unchecked")
@JsonIgnore
public Optional authMethod() {
return (Optional) authMethod;
}
public final static Builder builder() {
return new Builder();
}
/**
* The Personal Access Token for the Airtable account. See the <a href="https://airtable.com/developers/web/guides/personal-access-tokens">Support Guide</a> for more information on how to obtain this token.
*/
public PersonalAccessToken withApiKey(String apiKey) {
Utils.checkNotNull(apiKey, "apiKey");
this.apiKey = apiKey;
return this;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
PersonalAccessToken other = (PersonalAccessToken) o;
return
java.util.Objects.deepEquals(this.apiKey, other.apiKey) &&
java.util.Objects.deepEquals(this.authMethod, other.authMethod);
}
@Override
public int hashCode() {
return java.util.Objects.hash(
apiKey,
authMethod);
}
@Override
public String toString() {
return Utils.toString(PersonalAccessToken.class,
"apiKey", apiKey,
"authMethod", authMethod);
}
public final static class Builder {
private String apiKey;
private Builder() {
// force use of static builder() method
}
/**
* The Personal Access Token for the Airtable account. See the <a href="https://airtable.com/developers/web/guides/personal-access-tokens">Support Guide</a> for more information on how to obtain this token.
*/
public Builder apiKey(String apiKey) {
Utils.checkNotNull(apiKey, "apiKey");
this.apiKey = apiKey;
return this;
}
public PersonalAccessToken build() {
return new PersonalAccessToken(
apiKey);
}
private static final LazySingletonValue> _SINGLETON_VALUE_AuthMethod =
new LazySingletonValue<>(
"auth_method",
"\"api_key\"",
new TypeReference>() {});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy