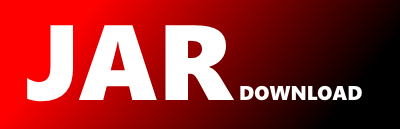
com.airbyte.api.models.shared.SSHSecureShell Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of api Show documentation
Show all versions of api Show documentation
SDK enabling Java developers to easily integrate with the Airbyte API.
The newest version!
/*
* Code generated by Speakeasy (https://speakeasyapi.dev). DO NOT EDIT.
*/
package com.airbyte.api.models.shared;
import com.airbyte.api.utils.LazySingletonValue;
import com.airbyte.api.utils.Utils;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonFormat;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.core.type.TypeReference;
import java.io.InputStream;
import java.lang.Deprecated;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.util.Optional;
public class SSHSecureShell {
@JsonProperty("host")
private String host;
@JsonInclude(Include.NON_ABSENT)
@JsonProperty("password")
private Optional extends String> password;
@JsonInclude(Include.NON_ABSENT)
@JsonProperty("port")
private Optional extends String> port;
@JsonProperty("storage")
private SourceFileSchemasProviderStorageProviderStorage storage;
@JsonProperty("user")
private String user;
@JsonCreator
public SSHSecureShell(
@JsonProperty("host") String host,
@JsonProperty("password") Optional extends String> password,
@JsonProperty("port") Optional extends String> port,
@JsonProperty("user") String user) {
Utils.checkNotNull(host, "host");
Utils.checkNotNull(password, "password");
Utils.checkNotNull(port, "port");
Utils.checkNotNull(user, "user");
this.host = host;
this.password = password;
this.port = port;
this.storage = Builder._SINGLETON_VALUE_Storage.value();
this.user = user;
}
public SSHSecureShell(
String host,
String user) {
this(host, Optional.empty(), Optional.empty(), user);
}
@JsonIgnore
public String host() {
return host;
}
@SuppressWarnings("unchecked")
@JsonIgnore
public Optional password() {
return (Optional) password;
}
@SuppressWarnings("unchecked")
@JsonIgnore
public Optional port() {
return (Optional) port;
}
@JsonIgnore
public SourceFileSchemasProviderStorageProviderStorage storage() {
return storage;
}
@JsonIgnore
public String user() {
return user;
}
public final static Builder builder() {
return new Builder();
}
public SSHSecureShell withHost(String host) {
Utils.checkNotNull(host, "host");
this.host = host;
return this;
}
public SSHSecureShell withPassword(String password) {
Utils.checkNotNull(password, "password");
this.password = Optional.ofNullable(password);
return this;
}
public SSHSecureShell withPassword(Optional extends String> password) {
Utils.checkNotNull(password, "password");
this.password = password;
return this;
}
public SSHSecureShell withPort(String port) {
Utils.checkNotNull(port, "port");
this.port = Optional.ofNullable(port);
return this;
}
public SSHSecureShell withPort(Optional extends String> port) {
Utils.checkNotNull(port, "port");
this.port = port;
return this;
}
public SSHSecureShell withUser(String user) {
Utils.checkNotNull(user, "user");
this.user = user;
return this;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SSHSecureShell other = (SSHSecureShell) o;
return
java.util.Objects.deepEquals(this.host, other.host) &&
java.util.Objects.deepEquals(this.password, other.password) &&
java.util.Objects.deepEquals(this.port, other.port) &&
java.util.Objects.deepEquals(this.storage, other.storage) &&
java.util.Objects.deepEquals(this.user, other.user);
}
@Override
public int hashCode() {
return java.util.Objects.hash(
host,
password,
port,
storage,
user);
}
@Override
public String toString() {
return Utils.toString(SSHSecureShell.class,
"host", host,
"password", password,
"port", port,
"storage", storage,
"user", user);
}
public final static class Builder {
private String host;
private Optional extends String> password = Optional.empty();
private Optional extends String> port;
private String user;
private Builder() {
// force use of static builder() method
}
public Builder host(String host) {
Utils.checkNotNull(host, "host");
this.host = host;
return this;
}
public Builder password(String password) {
Utils.checkNotNull(password, "password");
this.password = Optional.ofNullable(password);
return this;
}
public Builder password(Optional extends String> password) {
Utils.checkNotNull(password, "password");
this.password = password;
return this;
}
public Builder port(String port) {
Utils.checkNotNull(port, "port");
this.port = Optional.ofNullable(port);
return this;
}
public Builder port(Optional extends String> port) {
Utils.checkNotNull(port, "port");
this.port = port;
return this;
}
public Builder user(String user) {
Utils.checkNotNull(user, "user");
this.user = user;
return this;
}
public SSHSecureShell build() {
if (port == null) {
port = _SINGLETON_VALUE_Port.value();
}
return new SSHSecureShell(
host,
password,
port,
user);
}
private static final LazySingletonValue> _SINGLETON_VALUE_Port =
new LazySingletonValue<>(
"port",
"\"22\"",
new TypeReference>() {});
private static final LazySingletonValue _SINGLETON_VALUE_Storage =
new LazySingletonValue<>(
"storage",
"\"SSH\"",
new TypeReference() {});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy