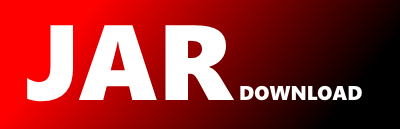
com.airbyte.api.models.shared.SourceGetlago Maven / Gradle / Ivy
/*
* Code generated by Speakeasy (https://speakeasyapi.dev). DO NOT EDIT.
*/
package com.airbyte.api.models.shared;
import com.airbyte.api.utils.LazySingletonValue;
import com.airbyte.api.utils.Utils;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonFormat;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.core.type.TypeReference;
import java.io.InputStream;
import java.lang.Deprecated;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.util.Optional;
public class SourceGetlago {
/**
* Your API Key. See <a href="https://doc.getlago.com/docs/api/intro">here</a>.
*/
@JsonProperty("api_key")
private String apiKey;
/**
* Your Lago API URL
*/
@JsonInclude(Include.NON_ABSENT)
@JsonProperty("api_url")
private Optional extends String> apiUrl;
@JsonProperty("sourceType")
private Getlago sourceType;
@JsonCreator
public SourceGetlago(
@JsonProperty("api_key") String apiKey,
@JsonProperty("api_url") Optional extends String> apiUrl) {
Utils.checkNotNull(apiKey, "apiKey");
Utils.checkNotNull(apiUrl, "apiUrl");
this.apiKey = apiKey;
this.apiUrl = apiUrl;
this.sourceType = Builder._SINGLETON_VALUE_SourceType.value();
}
public SourceGetlago(
String apiKey) {
this(apiKey, Optional.empty());
}
/**
* Your API Key. See <a href="https://doc.getlago.com/docs/api/intro">here</a>.
*/
@JsonIgnore
public String apiKey() {
return apiKey;
}
/**
* Your Lago API URL
*/
@SuppressWarnings("unchecked")
@JsonIgnore
public Optional apiUrl() {
return (Optional) apiUrl;
}
@JsonIgnore
public Getlago sourceType() {
return sourceType;
}
public final static Builder builder() {
return new Builder();
}
/**
* Your API Key. See <a href="https://doc.getlago.com/docs/api/intro">here</a>.
*/
public SourceGetlago withApiKey(String apiKey) {
Utils.checkNotNull(apiKey, "apiKey");
this.apiKey = apiKey;
return this;
}
/**
* Your Lago API URL
*/
public SourceGetlago withApiUrl(String apiUrl) {
Utils.checkNotNull(apiUrl, "apiUrl");
this.apiUrl = Optional.ofNullable(apiUrl);
return this;
}
/**
* Your Lago API URL
*/
public SourceGetlago withApiUrl(Optional extends String> apiUrl) {
Utils.checkNotNull(apiUrl, "apiUrl");
this.apiUrl = apiUrl;
return this;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SourceGetlago other = (SourceGetlago) o;
return
java.util.Objects.deepEquals(this.apiKey, other.apiKey) &&
java.util.Objects.deepEquals(this.apiUrl, other.apiUrl) &&
java.util.Objects.deepEquals(this.sourceType, other.sourceType);
}
@Override
public int hashCode() {
return java.util.Objects.hash(
apiKey,
apiUrl,
sourceType);
}
@Override
public String toString() {
return Utils.toString(SourceGetlago.class,
"apiKey", apiKey,
"apiUrl", apiUrl,
"sourceType", sourceType);
}
public final static class Builder {
private String apiKey;
private Optional extends String> apiUrl;
private Builder() {
// force use of static builder() method
}
/**
* Your API Key. See <a href="https://doc.getlago.com/docs/api/intro">here</a>.
*/
public Builder apiKey(String apiKey) {
Utils.checkNotNull(apiKey, "apiKey");
this.apiKey = apiKey;
return this;
}
/**
* Your Lago API URL
*/
public Builder apiUrl(String apiUrl) {
Utils.checkNotNull(apiUrl, "apiUrl");
this.apiUrl = Optional.ofNullable(apiUrl);
return this;
}
/**
* Your Lago API URL
*/
public Builder apiUrl(Optional extends String> apiUrl) {
Utils.checkNotNull(apiUrl, "apiUrl");
this.apiUrl = apiUrl;
return this;
}
public SourceGetlago build() {
if (apiUrl == null) {
apiUrl = _SINGLETON_VALUE_ApiUrl.value();
}
return new SourceGetlago(
apiKey,
apiUrl);
}
private static final LazySingletonValue> _SINGLETON_VALUE_ApiUrl =
new LazySingletonValue<>(
"api_url",
"\"https://api.getlago.com/api/v1\"",
new TypeReference>() {});
private static final LazySingletonValue _SINGLETON_VALUE_SourceType =
new LazySingletonValue<>(
"sourceType",
"\"getlago\"",
new TypeReference() {});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy