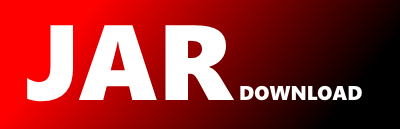
com.airbyte.api.models.shared.SourceGoogleAnalyticsDataApi Maven / Gradle / Ivy
/*
* Code generated by Speakeasy (https://speakeasyapi.dev). DO NOT EDIT.
*/
package com.airbyte.api.models.shared;
import com.airbyte.api.utils.LazySingletonValue;
import com.airbyte.api.utils.Utils;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonFormat;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.core.type.TypeReference;
import java.io.InputStream;
import java.lang.Deprecated;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.time.LocalDate;
import java.util.Optional;
public class SourceGoogleAnalyticsDataApi {
/**
* Enables conversion of `conversions:*` event metrics from integers to floats. This is beneficial for preventing data rounding when the API returns float values for any `conversions:*` fields.
*/
@JsonInclude(Include.NON_ABSENT)
@JsonProperty("convert_conversions_event")
private Optional extends Boolean> convertConversionsEvent;
/**
* Credentials for the service
*/
@JsonInclude(Include.NON_ABSENT)
@JsonProperty("credentials")
private Optional extends SourceGoogleAnalyticsDataApiCredentials> credentials;
/**
* You can add your Custom Analytics report by creating one.
*/
@JsonInclude(Include.NON_ABSENT)
@JsonProperty("custom_reports_array")
private Optional extends java.util.List> customReportsArray;
/**
* The start date from which to replicate report data in the format YYYY-MM-DD. Data generated before this date will not be included in the report. Not applied to custom Cohort reports.
*/
@JsonInclude(Include.NON_ABSENT)
@JsonProperty("date_ranges_start_date")
private Optional extends LocalDate> dateRangesStartDate;
/**
* If false, each row with all metrics equal to 0 will not be returned. If true, these rows will be returned if they are not separately removed by a filter. More information is available in <a href="https://developers.google.com/analytics/devguides/reporting/data/v1/rest/v1beta/properties/runReport#request-body">the documentation</a>.
*/
@JsonInclude(Include.NON_ABSENT)
@JsonProperty("keep_empty_rows")
private Optional extends Boolean> keepEmptyRows;
/**
* A list of your Property IDs. The Property ID is a unique number assigned to each property in Google Analytics, found in your GA4 property URL. This ID allows the connector to track the specific events associated with your property. Refer to the <a href='https://developers.google.com/analytics/devguides/reporting/data/v1/property-id#what_is_my_property_id'>Google Analytics documentation</a> to locate your property ID.
*/
@JsonProperty("property_ids")
private java.util.List propertyIds;
@JsonProperty("sourceType")
private SourceGoogleAnalyticsDataApiGoogleAnalyticsDataApi sourceType;
/**
* The interval in days for each data request made to the Google Analytics API. A larger value speeds up data sync, but increases the chance of data sampling, which may result in inaccuracies. We recommend a value of 1 to minimize sampling, unless speed is an absolute priority over accuracy. Acceptable values range from 1 to 364. Does not apply to custom Cohort reports. More information is available in <a href="https://docs.airbyte.com/integrations/sources/google-analytics-data-api">the documentation</a>.
*/
@JsonInclude(Include.NON_ABSENT)
@JsonProperty("window_in_days")
private Optional extends Long> windowInDays;
@JsonCreator
public SourceGoogleAnalyticsDataApi(
@JsonProperty("convert_conversions_event") Optional extends Boolean> convertConversionsEvent,
@JsonProperty("credentials") Optional extends SourceGoogleAnalyticsDataApiCredentials> credentials,
@JsonProperty("custom_reports_array") Optional extends java.util.List> customReportsArray,
@JsonProperty("date_ranges_start_date") Optional extends LocalDate> dateRangesStartDate,
@JsonProperty("keep_empty_rows") Optional extends Boolean> keepEmptyRows,
@JsonProperty("property_ids") java.util.List propertyIds,
@JsonProperty("window_in_days") Optional extends Long> windowInDays) {
Utils.checkNotNull(convertConversionsEvent, "convertConversionsEvent");
Utils.checkNotNull(credentials, "credentials");
Utils.checkNotNull(customReportsArray, "customReportsArray");
Utils.checkNotNull(dateRangesStartDate, "dateRangesStartDate");
Utils.checkNotNull(keepEmptyRows, "keepEmptyRows");
Utils.checkNotNull(propertyIds, "propertyIds");
Utils.checkNotNull(windowInDays, "windowInDays");
this.convertConversionsEvent = convertConversionsEvent;
this.credentials = credentials;
this.customReportsArray = customReportsArray;
this.dateRangesStartDate = dateRangesStartDate;
this.keepEmptyRows = keepEmptyRows;
this.propertyIds = propertyIds;
this.sourceType = Builder._SINGLETON_VALUE_SourceType.value();
this.windowInDays = windowInDays;
}
public SourceGoogleAnalyticsDataApi(
java.util.List propertyIds) {
this(Optional.empty(), Optional.empty(), Optional.empty(), Optional.empty(), Optional.empty(), propertyIds, Optional.empty());
}
/**
* Enables conversion of `conversions:*` event metrics from integers to floats. This is beneficial for preventing data rounding when the API returns float values for any `conversions:*` fields.
*/
@SuppressWarnings("unchecked")
@JsonIgnore
public Optional convertConversionsEvent() {
return (Optional) convertConversionsEvent;
}
/**
* Credentials for the service
*/
@SuppressWarnings("unchecked")
@JsonIgnore
public Optional credentials() {
return (Optional) credentials;
}
/**
* You can add your Custom Analytics report by creating one.
*/
@SuppressWarnings("unchecked")
@JsonIgnore
public Optional> customReportsArray() {
return (Optional>) customReportsArray;
}
/**
* The start date from which to replicate report data in the format YYYY-MM-DD. Data generated before this date will not be included in the report. Not applied to custom Cohort reports.
*/
@SuppressWarnings("unchecked")
@JsonIgnore
public Optional dateRangesStartDate() {
return (Optional) dateRangesStartDate;
}
/**
* If false, each row with all metrics equal to 0 will not be returned. If true, these rows will be returned if they are not separately removed by a filter. More information is available in <a href="https://developers.google.com/analytics/devguides/reporting/data/v1/rest/v1beta/properties/runReport#request-body">the documentation</a>.
*/
@SuppressWarnings("unchecked")
@JsonIgnore
public Optional keepEmptyRows() {
return (Optional) keepEmptyRows;
}
/**
* A list of your Property IDs. The Property ID is a unique number assigned to each property in Google Analytics, found in your GA4 property URL. This ID allows the connector to track the specific events associated with your property. Refer to the <a href='https://developers.google.com/analytics/devguides/reporting/data/v1/property-id#what_is_my_property_id'>Google Analytics documentation</a> to locate your property ID.
*/
@JsonIgnore
public java.util.List propertyIds() {
return propertyIds;
}
@JsonIgnore
public SourceGoogleAnalyticsDataApiGoogleAnalyticsDataApi sourceType() {
return sourceType;
}
/**
* The interval in days for each data request made to the Google Analytics API. A larger value speeds up data sync, but increases the chance of data sampling, which may result in inaccuracies. We recommend a value of 1 to minimize sampling, unless speed is an absolute priority over accuracy. Acceptable values range from 1 to 364. Does not apply to custom Cohort reports. More information is available in <a href="https://docs.airbyte.com/integrations/sources/google-analytics-data-api">the documentation</a>.
*/
@SuppressWarnings("unchecked")
@JsonIgnore
public Optional windowInDays() {
return (Optional) windowInDays;
}
public final static Builder builder() {
return new Builder();
}
/**
* Enables conversion of `conversions:*` event metrics from integers to floats. This is beneficial for preventing data rounding when the API returns float values for any `conversions:*` fields.
*/
public SourceGoogleAnalyticsDataApi withConvertConversionsEvent(boolean convertConversionsEvent) {
Utils.checkNotNull(convertConversionsEvent, "convertConversionsEvent");
this.convertConversionsEvent = Optional.ofNullable(convertConversionsEvent);
return this;
}
/**
* Enables conversion of `conversions:*` event metrics from integers to floats. This is beneficial for preventing data rounding when the API returns float values for any `conversions:*` fields.
*/
public SourceGoogleAnalyticsDataApi withConvertConversionsEvent(Optional extends Boolean> convertConversionsEvent) {
Utils.checkNotNull(convertConversionsEvent, "convertConversionsEvent");
this.convertConversionsEvent = convertConversionsEvent;
return this;
}
/**
* Credentials for the service
*/
public SourceGoogleAnalyticsDataApi withCredentials(SourceGoogleAnalyticsDataApiCredentials credentials) {
Utils.checkNotNull(credentials, "credentials");
this.credentials = Optional.ofNullable(credentials);
return this;
}
/**
* Credentials for the service
*/
public SourceGoogleAnalyticsDataApi withCredentials(Optional extends SourceGoogleAnalyticsDataApiCredentials> credentials) {
Utils.checkNotNull(credentials, "credentials");
this.credentials = credentials;
return this;
}
/**
* You can add your Custom Analytics report by creating one.
*/
public SourceGoogleAnalyticsDataApi withCustomReportsArray(java.util.List customReportsArray) {
Utils.checkNotNull(customReportsArray, "customReportsArray");
this.customReportsArray = Optional.ofNullable(customReportsArray);
return this;
}
/**
* You can add your Custom Analytics report by creating one.
*/
public SourceGoogleAnalyticsDataApi withCustomReportsArray(Optional extends java.util.List> customReportsArray) {
Utils.checkNotNull(customReportsArray, "customReportsArray");
this.customReportsArray = customReportsArray;
return this;
}
/**
* The start date from which to replicate report data in the format YYYY-MM-DD. Data generated before this date will not be included in the report. Not applied to custom Cohort reports.
*/
public SourceGoogleAnalyticsDataApi withDateRangesStartDate(LocalDate dateRangesStartDate) {
Utils.checkNotNull(dateRangesStartDate, "dateRangesStartDate");
this.dateRangesStartDate = Optional.ofNullable(dateRangesStartDate);
return this;
}
/**
* The start date from which to replicate report data in the format YYYY-MM-DD. Data generated before this date will not be included in the report. Not applied to custom Cohort reports.
*/
public SourceGoogleAnalyticsDataApi withDateRangesStartDate(Optional extends LocalDate> dateRangesStartDate) {
Utils.checkNotNull(dateRangesStartDate, "dateRangesStartDate");
this.dateRangesStartDate = dateRangesStartDate;
return this;
}
/**
* If false, each row with all metrics equal to 0 will not be returned. If true, these rows will be returned if they are not separately removed by a filter. More information is available in <a href="https://developers.google.com/analytics/devguides/reporting/data/v1/rest/v1beta/properties/runReport#request-body">the documentation</a>.
*/
public SourceGoogleAnalyticsDataApi withKeepEmptyRows(boolean keepEmptyRows) {
Utils.checkNotNull(keepEmptyRows, "keepEmptyRows");
this.keepEmptyRows = Optional.ofNullable(keepEmptyRows);
return this;
}
/**
* If false, each row with all metrics equal to 0 will not be returned. If true, these rows will be returned if they are not separately removed by a filter. More information is available in <a href="https://developers.google.com/analytics/devguides/reporting/data/v1/rest/v1beta/properties/runReport#request-body">the documentation</a>.
*/
public SourceGoogleAnalyticsDataApi withKeepEmptyRows(Optional extends Boolean> keepEmptyRows) {
Utils.checkNotNull(keepEmptyRows, "keepEmptyRows");
this.keepEmptyRows = keepEmptyRows;
return this;
}
/**
* A list of your Property IDs. The Property ID is a unique number assigned to each property in Google Analytics, found in your GA4 property URL. This ID allows the connector to track the specific events associated with your property. Refer to the <a href='https://developers.google.com/analytics/devguides/reporting/data/v1/property-id#what_is_my_property_id'>Google Analytics documentation</a> to locate your property ID.
*/
public SourceGoogleAnalyticsDataApi withPropertyIds(java.util.List propertyIds) {
Utils.checkNotNull(propertyIds, "propertyIds");
this.propertyIds = propertyIds;
return this;
}
/**
* The interval in days for each data request made to the Google Analytics API. A larger value speeds up data sync, but increases the chance of data sampling, which may result in inaccuracies. We recommend a value of 1 to minimize sampling, unless speed is an absolute priority over accuracy. Acceptable values range from 1 to 364. Does not apply to custom Cohort reports. More information is available in <a href="https://docs.airbyte.com/integrations/sources/google-analytics-data-api">the documentation</a>.
*/
public SourceGoogleAnalyticsDataApi withWindowInDays(long windowInDays) {
Utils.checkNotNull(windowInDays, "windowInDays");
this.windowInDays = Optional.ofNullable(windowInDays);
return this;
}
/**
* The interval in days for each data request made to the Google Analytics API. A larger value speeds up data sync, but increases the chance of data sampling, which may result in inaccuracies. We recommend a value of 1 to minimize sampling, unless speed is an absolute priority over accuracy. Acceptable values range from 1 to 364. Does not apply to custom Cohort reports. More information is available in <a href="https://docs.airbyte.com/integrations/sources/google-analytics-data-api">the documentation</a>.
*/
public SourceGoogleAnalyticsDataApi withWindowInDays(Optional extends Long> windowInDays) {
Utils.checkNotNull(windowInDays, "windowInDays");
this.windowInDays = windowInDays;
return this;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SourceGoogleAnalyticsDataApi other = (SourceGoogleAnalyticsDataApi) o;
return
java.util.Objects.deepEquals(this.convertConversionsEvent, other.convertConversionsEvent) &&
java.util.Objects.deepEquals(this.credentials, other.credentials) &&
java.util.Objects.deepEquals(this.customReportsArray, other.customReportsArray) &&
java.util.Objects.deepEquals(this.dateRangesStartDate, other.dateRangesStartDate) &&
java.util.Objects.deepEquals(this.keepEmptyRows, other.keepEmptyRows) &&
java.util.Objects.deepEquals(this.propertyIds, other.propertyIds) &&
java.util.Objects.deepEquals(this.sourceType, other.sourceType) &&
java.util.Objects.deepEquals(this.windowInDays, other.windowInDays);
}
@Override
public int hashCode() {
return java.util.Objects.hash(
convertConversionsEvent,
credentials,
customReportsArray,
dateRangesStartDate,
keepEmptyRows,
propertyIds,
sourceType,
windowInDays);
}
@Override
public String toString() {
return Utils.toString(SourceGoogleAnalyticsDataApi.class,
"convertConversionsEvent", convertConversionsEvent,
"credentials", credentials,
"customReportsArray", customReportsArray,
"dateRangesStartDate", dateRangesStartDate,
"keepEmptyRows", keepEmptyRows,
"propertyIds", propertyIds,
"sourceType", sourceType,
"windowInDays", windowInDays);
}
public final static class Builder {
private Optional extends Boolean> convertConversionsEvent;
private Optional extends SourceGoogleAnalyticsDataApiCredentials> credentials = Optional.empty();
private Optional extends java.util.List> customReportsArray = Optional.empty();
private Optional extends LocalDate> dateRangesStartDate = Optional.empty();
private Optional extends Boolean> keepEmptyRows;
private java.util.List propertyIds;
private Optional extends Long> windowInDays;
private Builder() {
// force use of static builder() method
}
/**
* Enables conversion of `conversions:*` event metrics from integers to floats. This is beneficial for preventing data rounding when the API returns float values for any `conversions:*` fields.
*/
public Builder convertConversionsEvent(boolean convertConversionsEvent) {
Utils.checkNotNull(convertConversionsEvent, "convertConversionsEvent");
this.convertConversionsEvent = Optional.ofNullable(convertConversionsEvent);
return this;
}
/**
* Enables conversion of `conversions:*` event metrics from integers to floats. This is beneficial for preventing data rounding when the API returns float values for any `conversions:*` fields.
*/
public Builder convertConversionsEvent(Optional extends Boolean> convertConversionsEvent) {
Utils.checkNotNull(convertConversionsEvent, "convertConversionsEvent");
this.convertConversionsEvent = convertConversionsEvent;
return this;
}
/**
* Credentials for the service
*/
public Builder credentials(SourceGoogleAnalyticsDataApiCredentials credentials) {
Utils.checkNotNull(credentials, "credentials");
this.credentials = Optional.ofNullable(credentials);
return this;
}
/**
* Credentials for the service
*/
public Builder credentials(Optional extends SourceGoogleAnalyticsDataApiCredentials> credentials) {
Utils.checkNotNull(credentials, "credentials");
this.credentials = credentials;
return this;
}
/**
* You can add your Custom Analytics report by creating one.
*/
public Builder customReportsArray(java.util.List customReportsArray) {
Utils.checkNotNull(customReportsArray, "customReportsArray");
this.customReportsArray = Optional.ofNullable(customReportsArray);
return this;
}
/**
* You can add your Custom Analytics report by creating one.
*/
public Builder customReportsArray(Optional extends java.util.List> customReportsArray) {
Utils.checkNotNull(customReportsArray, "customReportsArray");
this.customReportsArray = customReportsArray;
return this;
}
/**
* The start date from which to replicate report data in the format YYYY-MM-DD. Data generated before this date will not be included in the report. Not applied to custom Cohort reports.
*/
public Builder dateRangesStartDate(LocalDate dateRangesStartDate) {
Utils.checkNotNull(dateRangesStartDate, "dateRangesStartDate");
this.dateRangesStartDate = Optional.ofNullable(dateRangesStartDate);
return this;
}
/**
* The start date from which to replicate report data in the format YYYY-MM-DD. Data generated before this date will not be included in the report. Not applied to custom Cohort reports.
*/
public Builder dateRangesStartDate(Optional extends LocalDate> dateRangesStartDate) {
Utils.checkNotNull(dateRangesStartDate, "dateRangesStartDate");
this.dateRangesStartDate = dateRangesStartDate;
return this;
}
/**
* If false, each row with all metrics equal to 0 will not be returned. If true, these rows will be returned if they are not separately removed by a filter. More information is available in <a href="https://developers.google.com/analytics/devguides/reporting/data/v1/rest/v1beta/properties/runReport#request-body">the documentation</a>.
*/
public Builder keepEmptyRows(boolean keepEmptyRows) {
Utils.checkNotNull(keepEmptyRows, "keepEmptyRows");
this.keepEmptyRows = Optional.ofNullable(keepEmptyRows);
return this;
}
/**
* If false, each row with all metrics equal to 0 will not be returned. If true, these rows will be returned if they are not separately removed by a filter. More information is available in <a href="https://developers.google.com/analytics/devguides/reporting/data/v1/rest/v1beta/properties/runReport#request-body">the documentation</a>.
*/
public Builder keepEmptyRows(Optional extends Boolean> keepEmptyRows) {
Utils.checkNotNull(keepEmptyRows, "keepEmptyRows");
this.keepEmptyRows = keepEmptyRows;
return this;
}
/**
* A list of your Property IDs. The Property ID is a unique number assigned to each property in Google Analytics, found in your GA4 property URL. This ID allows the connector to track the specific events associated with your property. Refer to the <a href='https://developers.google.com/analytics/devguides/reporting/data/v1/property-id#what_is_my_property_id'>Google Analytics documentation</a> to locate your property ID.
*/
public Builder propertyIds(java.util.List propertyIds) {
Utils.checkNotNull(propertyIds, "propertyIds");
this.propertyIds = propertyIds;
return this;
}
/**
* The interval in days for each data request made to the Google Analytics API. A larger value speeds up data sync, but increases the chance of data sampling, which may result in inaccuracies. We recommend a value of 1 to minimize sampling, unless speed is an absolute priority over accuracy. Acceptable values range from 1 to 364. Does not apply to custom Cohort reports. More information is available in <a href="https://docs.airbyte.com/integrations/sources/google-analytics-data-api">the documentation</a>.
*/
public Builder windowInDays(long windowInDays) {
Utils.checkNotNull(windowInDays, "windowInDays");
this.windowInDays = Optional.ofNullable(windowInDays);
return this;
}
/**
* The interval in days for each data request made to the Google Analytics API. A larger value speeds up data sync, but increases the chance of data sampling, which may result in inaccuracies. We recommend a value of 1 to minimize sampling, unless speed is an absolute priority over accuracy. Acceptable values range from 1 to 364. Does not apply to custom Cohort reports. More information is available in <a href="https://docs.airbyte.com/integrations/sources/google-analytics-data-api">the documentation</a>.
*/
public Builder windowInDays(Optional extends Long> windowInDays) {
Utils.checkNotNull(windowInDays, "windowInDays");
this.windowInDays = windowInDays;
return this;
}
public SourceGoogleAnalyticsDataApi build() {
if (convertConversionsEvent == null) {
convertConversionsEvent = _SINGLETON_VALUE_ConvertConversionsEvent.value();
}
if (keepEmptyRows == null) {
keepEmptyRows = _SINGLETON_VALUE_KeepEmptyRows.value();
}
if (windowInDays == null) {
windowInDays = _SINGLETON_VALUE_WindowInDays.value();
}
return new SourceGoogleAnalyticsDataApi(
convertConversionsEvent,
credentials,
customReportsArray,
dateRangesStartDate,
keepEmptyRows,
propertyIds,
windowInDays);
}
private static final LazySingletonValue> _SINGLETON_VALUE_ConvertConversionsEvent =
new LazySingletonValue<>(
"convert_conversions_event",
"false",
new TypeReference>() {});
private static final LazySingletonValue> _SINGLETON_VALUE_KeepEmptyRows =
new LazySingletonValue<>(
"keep_empty_rows",
"false",
new TypeReference>() {});
private static final LazySingletonValue _SINGLETON_VALUE_SourceType =
new LazySingletonValue<>(
"sourceType",
"\"google-analytics-data-api\"",
new TypeReference() {});
private static final LazySingletonValue> _SINGLETON_VALUE_WindowInDays =
new LazySingletonValue<>(
"window_in_days",
"1",
new TypeReference>() {});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy