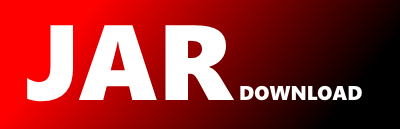
com.airbyte.api.models.shared.SourceGoogleSearchConsole Maven / Gradle / Ivy
/*
* Code generated by Speakeasy (https://speakeasyapi.dev). DO NOT EDIT.
*/
package com.airbyte.api.models.shared;
import com.airbyte.api.utils.LazySingletonValue;
import com.airbyte.api.utils.Utils;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonFormat;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.core.type.TypeReference;
import java.io.InputStream;
import java.lang.Deprecated;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.time.LocalDate;
import java.util.Optional;
public class SourceGoogleSearchConsole {
@JsonProperty("authorization")
private AuthenticationType authorization;
/**
* (DEPRCATED) A JSON array describing the custom reports you want to sync from Google Search Console. See our <a href='https://docs.airbyte.com/integrations/sources/google-search-console'>documentation</a> for more information on formulating custom reports.
*/
@JsonInclude(Include.NON_ABSENT)
@JsonProperty("custom_reports")
private Optional extends String> customReports;
/**
* You can add your Custom Analytics report by creating one.
*/
@JsonInclude(Include.NON_ABSENT)
@JsonProperty("custom_reports_array")
private Optional extends java.util.List> customReportsArray;
/**
* If set to 'final', the returned data will include only finalized, stable data. If set to 'all', fresh data will be included. When using Incremental sync mode, we do not recommend setting this parameter to 'all' as it may cause data loss. More information can be found in our <a href='https://docs.airbyte.com/integrations/source/google-search-console'>full documentation</a>.
*/
@JsonInclude(Include.NON_ABSENT)
@JsonProperty("data_state")
private Optional extends DataFreshness> dataState;
/**
* UTC date in the format YYYY-MM-DD. Any data created after this date will not be replicated. Must be greater or equal to the start date field. Leaving this field blank will replicate all data from the start date onward.
*/
@JsonInclude(Include.NON_ABSENT)
@JsonProperty("end_date")
private Optional extends LocalDate> endDate;
/**
* The URLs of the website property attached to your GSC account. Learn more about properties <a href="https://support.google.com/webmasters/answer/34592?hl=en">here</a>.
*/
@JsonProperty("site_urls")
private java.util.List siteUrls;
@JsonProperty("sourceType")
private SourceGoogleSearchConsoleGoogleSearchConsole sourceType;
/**
* UTC date in the format YYYY-MM-DD. Any data before this date will not be replicated.
*/
@JsonInclude(Include.NON_ABSENT)
@JsonProperty("start_date")
private Optional extends LocalDate> startDate;
@JsonCreator
public SourceGoogleSearchConsole(
@JsonProperty("authorization") AuthenticationType authorization,
@JsonProperty("custom_reports") Optional extends String> customReports,
@JsonProperty("custom_reports_array") Optional extends java.util.List> customReportsArray,
@JsonProperty("data_state") Optional extends DataFreshness> dataState,
@JsonProperty("end_date") Optional extends LocalDate> endDate,
@JsonProperty("site_urls") java.util.List siteUrls,
@JsonProperty("start_date") Optional extends LocalDate> startDate) {
Utils.checkNotNull(authorization, "authorization");
Utils.checkNotNull(customReports, "customReports");
Utils.checkNotNull(customReportsArray, "customReportsArray");
Utils.checkNotNull(dataState, "dataState");
Utils.checkNotNull(endDate, "endDate");
Utils.checkNotNull(siteUrls, "siteUrls");
Utils.checkNotNull(startDate, "startDate");
this.authorization = authorization;
this.customReports = customReports;
this.customReportsArray = customReportsArray;
this.dataState = dataState;
this.endDate = endDate;
this.siteUrls = siteUrls;
this.sourceType = Builder._SINGLETON_VALUE_SourceType.value();
this.startDate = startDate;
}
public SourceGoogleSearchConsole(
AuthenticationType authorization,
java.util.List siteUrls) {
this(authorization, Optional.empty(), Optional.empty(), Optional.empty(), Optional.empty(), siteUrls, Optional.empty());
}
@JsonIgnore
public AuthenticationType authorization() {
return authorization;
}
/**
* (DEPRCATED) A JSON array describing the custom reports you want to sync from Google Search Console. See our <a href='https://docs.airbyte.com/integrations/sources/google-search-console'>documentation</a> for more information on formulating custom reports.
*/
@SuppressWarnings("unchecked")
@JsonIgnore
public Optional customReports() {
return (Optional) customReports;
}
/**
* You can add your Custom Analytics report by creating one.
*/
@SuppressWarnings("unchecked")
@JsonIgnore
public Optional> customReportsArray() {
return (Optional>) customReportsArray;
}
/**
* If set to 'final', the returned data will include only finalized, stable data. If set to 'all', fresh data will be included. When using Incremental sync mode, we do not recommend setting this parameter to 'all' as it may cause data loss. More information can be found in our <a href='https://docs.airbyte.com/integrations/source/google-search-console'>full documentation</a>.
*/
@SuppressWarnings("unchecked")
@JsonIgnore
public Optional dataState() {
return (Optional) dataState;
}
/**
* UTC date in the format YYYY-MM-DD. Any data created after this date will not be replicated. Must be greater or equal to the start date field. Leaving this field blank will replicate all data from the start date onward.
*/
@SuppressWarnings("unchecked")
@JsonIgnore
public Optional endDate() {
return (Optional) endDate;
}
/**
* The URLs of the website property attached to your GSC account. Learn more about properties <a href="https://support.google.com/webmasters/answer/34592?hl=en">here</a>.
*/
@JsonIgnore
public java.util.List siteUrls() {
return siteUrls;
}
@JsonIgnore
public SourceGoogleSearchConsoleGoogleSearchConsole sourceType() {
return sourceType;
}
/**
* UTC date in the format YYYY-MM-DD. Any data before this date will not be replicated.
*/
@SuppressWarnings("unchecked")
@JsonIgnore
public Optional startDate() {
return (Optional) startDate;
}
public final static Builder builder() {
return new Builder();
}
public SourceGoogleSearchConsole withAuthorization(AuthenticationType authorization) {
Utils.checkNotNull(authorization, "authorization");
this.authorization = authorization;
return this;
}
/**
* (DEPRCATED) A JSON array describing the custom reports you want to sync from Google Search Console. See our <a href='https://docs.airbyte.com/integrations/sources/google-search-console'>documentation</a> for more information on formulating custom reports.
*/
public SourceGoogleSearchConsole withCustomReports(String customReports) {
Utils.checkNotNull(customReports, "customReports");
this.customReports = Optional.ofNullable(customReports);
return this;
}
/**
* (DEPRCATED) A JSON array describing the custom reports you want to sync from Google Search Console. See our <a href='https://docs.airbyte.com/integrations/sources/google-search-console'>documentation</a> for more information on formulating custom reports.
*/
public SourceGoogleSearchConsole withCustomReports(Optional extends String> customReports) {
Utils.checkNotNull(customReports, "customReports");
this.customReports = customReports;
return this;
}
/**
* You can add your Custom Analytics report by creating one.
*/
public SourceGoogleSearchConsole withCustomReportsArray(java.util.List customReportsArray) {
Utils.checkNotNull(customReportsArray, "customReportsArray");
this.customReportsArray = Optional.ofNullable(customReportsArray);
return this;
}
/**
* You can add your Custom Analytics report by creating one.
*/
public SourceGoogleSearchConsole withCustomReportsArray(Optional extends java.util.List> customReportsArray) {
Utils.checkNotNull(customReportsArray, "customReportsArray");
this.customReportsArray = customReportsArray;
return this;
}
/**
* If set to 'final', the returned data will include only finalized, stable data. If set to 'all', fresh data will be included. When using Incremental sync mode, we do not recommend setting this parameter to 'all' as it may cause data loss. More information can be found in our <a href='https://docs.airbyte.com/integrations/source/google-search-console'>full documentation</a>.
*/
public SourceGoogleSearchConsole withDataState(DataFreshness dataState) {
Utils.checkNotNull(dataState, "dataState");
this.dataState = Optional.ofNullable(dataState);
return this;
}
/**
* If set to 'final', the returned data will include only finalized, stable data. If set to 'all', fresh data will be included. When using Incremental sync mode, we do not recommend setting this parameter to 'all' as it may cause data loss. More information can be found in our <a href='https://docs.airbyte.com/integrations/source/google-search-console'>full documentation</a>.
*/
public SourceGoogleSearchConsole withDataState(Optional extends DataFreshness> dataState) {
Utils.checkNotNull(dataState, "dataState");
this.dataState = dataState;
return this;
}
/**
* UTC date in the format YYYY-MM-DD. Any data created after this date will not be replicated. Must be greater or equal to the start date field. Leaving this field blank will replicate all data from the start date onward.
*/
public SourceGoogleSearchConsole withEndDate(LocalDate endDate) {
Utils.checkNotNull(endDate, "endDate");
this.endDate = Optional.ofNullable(endDate);
return this;
}
/**
* UTC date in the format YYYY-MM-DD. Any data created after this date will not be replicated. Must be greater or equal to the start date field. Leaving this field blank will replicate all data from the start date onward.
*/
public SourceGoogleSearchConsole withEndDate(Optional extends LocalDate> endDate) {
Utils.checkNotNull(endDate, "endDate");
this.endDate = endDate;
return this;
}
/**
* The URLs of the website property attached to your GSC account. Learn more about properties <a href="https://support.google.com/webmasters/answer/34592?hl=en">here</a>.
*/
public SourceGoogleSearchConsole withSiteUrls(java.util.List siteUrls) {
Utils.checkNotNull(siteUrls, "siteUrls");
this.siteUrls = siteUrls;
return this;
}
/**
* UTC date in the format YYYY-MM-DD. Any data before this date will not be replicated.
*/
public SourceGoogleSearchConsole withStartDate(LocalDate startDate) {
Utils.checkNotNull(startDate, "startDate");
this.startDate = Optional.ofNullable(startDate);
return this;
}
/**
* UTC date in the format YYYY-MM-DD. Any data before this date will not be replicated.
*/
public SourceGoogleSearchConsole withStartDate(Optional extends LocalDate> startDate) {
Utils.checkNotNull(startDate, "startDate");
this.startDate = startDate;
return this;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SourceGoogleSearchConsole other = (SourceGoogleSearchConsole) o;
return
java.util.Objects.deepEquals(this.authorization, other.authorization) &&
java.util.Objects.deepEquals(this.customReports, other.customReports) &&
java.util.Objects.deepEquals(this.customReportsArray, other.customReportsArray) &&
java.util.Objects.deepEquals(this.dataState, other.dataState) &&
java.util.Objects.deepEquals(this.endDate, other.endDate) &&
java.util.Objects.deepEquals(this.siteUrls, other.siteUrls) &&
java.util.Objects.deepEquals(this.sourceType, other.sourceType) &&
java.util.Objects.deepEquals(this.startDate, other.startDate);
}
@Override
public int hashCode() {
return java.util.Objects.hash(
authorization,
customReports,
customReportsArray,
dataState,
endDate,
siteUrls,
sourceType,
startDate);
}
@Override
public String toString() {
return Utils.toString(SourceGoogleSearchConsole.class,
"authorization", authorization,
"customReports", customReports,
"customReportsArray", customReportsArray,
"dataState", dataState,
"endDate", endDate,
"siteUrls", siteUrls,
"sourceType", sourceType,
"startDate", startDate);
}
public final static class Builder {
private AuthenticationType authorization;
private Optional extends String> customReports = Optional.empty();
private Optional extends java.util.List> customReportsArray = Optional.empty();
private Optional extends DataFreshness> dataState;
private Optional extends LocalDate> endDate = Optional.empty();
private java.util.List siteUrls;
private Optional extends LocalDate> startDate;
private Builder() {
// force use of static builder() method
}
public Builder authorization(AuthenticationType authorization) {
Utils.checkNotNull(authorization, "authorization");
this.authorization = authorization;
return this;
}
/**
* (DEPRCATED) A JSON array describing the custom reports you want to sync from Google Search Console. See our <a href='https://docs.airbyte.com/integrations/sources/google-search-console'>documentation</a> for more information on formulating custom reports.
*/
public Builder customReports(String customReports) {
Utils.checkNotNull(customReports, "customReports");
this.customReports = Optional.ofNullable(customReports);
return this;
}
/**
* (DEPRCATED) A JSON array describing the custom reports you want to sync from Google Search Console. See our <a href='https://docs.airbyte.com/integrations/sources/google-search-console'>documentation</a> for more information on formulating custom reports.
*/
public Builder customReports(Optional extends String> customReports) {
Utils.checkNotNull(customReports, "customReports");
this.customReports = customReports;
return this;
}
/**
* You can add your Custom Analytics report by creating one.
*/
public Builder customReportsArray(java.util.List customReportsArray) {
Utils.checkNotNull(customReportsArray, "customReportsArray");
this.customReportsArray = Optional.ofNullable(customReportsArray);
return this;
}
/**
* You can add your Custom Analytics report by creating one.
*/
public Builder customReportsArray(Optional extends java.util.List> customReportsArray) {
Utils.checkNotNull(customReportsArray, "customReportsArray");
this.customReportsArray = customReportsArray;
return this;
}
/**
* If set to 'final', the returned data will include only finalized, stable data. If set to 'all', fresh data will be included. When using Incremental sync mode, we do not recommend setting this parameter to 'all' as it may cause data loss. More information can be found in our <a href='https://docs.airbyte.com/integrations/source/google-search-console'>full documentation</a>.
*/
public Builder dataState(DataFreshness dataState) {
Utils.checkNotNull(dataState, "dataState");
this.dataState = Optional.ofNullable(dataState);
return this;
}
/**
* If set to 'final', the returned data will include only finalized, stable data. If set to 'all', fresh data will be included. When using Incremental sync mode, we do not recommend setting this parameter to 'all' as it may cause data loss. More information can be found in our <a href='https://docs.airbyte.com/integrations/source/google-search-console'>full documentation</a>.
*/
public Builder dataState(Optional extends DataFreshness> dataState) {
Utils.checkNotNull(dataState, "dataState");
this.dataState = dataState;
return this;
}
/**
* UTC date in the format YYYY-MM-DD. Any data created after this date will not be replicated. Must be greater or equal to the start date field. Leaving this field blank will replicate all data from the start date onward.
*/
public Builder endDate(LocalDate endDate) {
Utils.checkNotNull(endDate, "endDate");
this.endDate = Optional.ofNullable(endDate);
return this;
}
/**
* UTC date in the format YYYY-MM-DD. Any data created after this date will not be replicated. Must be greater or equal to the start date field. Leaving this field blank will replicate all data from the start date onward.
*/
public Builder endDate(Optional extends LocalDate> endDate) {
Utils.checkNotNull(endDate, "endDate");
this.endDate = endDate;
return this;
}
/**
* The URLs of the website property attached to your GSC account. Learn more about properties <a href="https://support.google.com/webmasters/answer/34592?hl=en">here</a>.
*/
public Builder siteUrls(java.util.List siteUrls) {
Utils.checkNotNull(siteUrls, "siteUrls");
this.siteUrls = siteUrls;
return this;
}
/**
* UTC date in the format YYYY-MM-DD. Any data before this date will not be replicated.
*/
public Builder startDate(LocalDate startDate) {
Utils.checkNotNull(startDate, "startDate");
this.startDate = Optional.ofNullable(startDate);
return this;
}
/**
* UTC date in the format YYYY-MM-DD. Any data before this date will not be replicated.
*/
public Builder startDate(Optional extends LocalDate> startDate) {
Utils.checkNotNull(startDate, "startDate");
this.startDate = startDate;
return this;
}
public SourceGoogleSearchConsole build() {
if (dataState == null) {
dataState = _SINGLETON_VALUE_DataState.value();
}
if (startDate == null) {
startDate = _SINGLETON_VALUE_StartDate.value();
}
return new SourceGoogleSearchConsole(
authorization,
customReports,
customReportsArray,
dataState,
endDate,
siteUrls,
startDate);
}
private static final LazySingletonValue> _SINGLETON_VALUE_DataState =
new LazySingletonValue<>(
"data_state",
"\"final\"",
new TypeReference>() {});
private static final LazySingletonValue _SINGLETON_VALUE_SourceType =
new LazySingletonValue<>(
"sourceType",
"\"google-search-console\"",
new TypeReference() {});
private static final LazySingletonValue> _SINGLETON_VALUE_StartDate =
new LazySingletonValue<>(
"start_date",
"\"2021-01-01\"",
new TypeReference>() {});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy