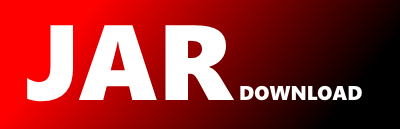
com.airbyte.api.models.shared.SourceMailchimpOAuth20 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of api Show documentation
Show all versions of api Show documentation
SDK enabling Java developers to easily integrate with the Airbyte API.
The newest version!
/*
* Code generated by Speakeasy (https://speakeasyapi.dev). DO NOT EDIT.
*/
package com.airbyte.api.models.shared;
import com.airbyte.api.utils.LazySingletonValue;
import com.airbyte.api.utils.Utils;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonFormat;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.core.type.TypeReference;
import java.io.InputStream;
import java.lang.Deprecated;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.util.Optional;
public class SourceMailchimpOAuth20 {
/**
* An access token generated using the above client ID and secret.
*/
@JsonProperty("access_token")
private String accessToken;
@JsonProperty("auth_type")
private SourceMailchimpAuthType authType;
/**
* The Client ID of your OAuth application.
*/
@JsonInclude(Include.NON_ABSENT)
@JsonProperty("client_id")
private Optional extends String> clientId;
/**
* The Client Secret of your OAuth application.
*/
@JsonInclude(Include.NON_ABSENT)
@JsonProperty("client_secret")
private Optional extends String> clientSecret;
@JsonCreator
public SourceMailchimpOAuth20(
@JsonProperty("access_token") String accessToken,
@JsonProperty("client_id") Optional extends String> clientId,
@JsonProperty("client_secret") Optional extends String> clientSecret) {
Utils.checkNotNull(accessToken, "accessToken");
Utils.checkNotNull(clientId, "clientId");
Utils.checkNotNull(clientSecret, "clientSecret");
this.accessToken = accessToken;
this.authType = Builder._SINGLETON_VALUE_AuthType.value();
this.clientId = clientId;
this.clientSecret = clientSecret;
}
public SourceMailchimpOAuth20(
String accessToken) {
this(accessToken, Optional.empty(), Optional.empty());
}
/**
* An access token generated using the above client ID and secret.
*/
@JsonIgnore
public String accessToken() {
return accessToken;
}
@JsonIgnore
public SourceMailchimpAuthType authType() {
return authType;
}
/**
* The Client ID of your OAuth application.
*/
@SuppressWarnings("unchecked")
@JsonIgnore
public Optional clientId() {
return (Optional) clientId;
}
/**
* The Client Secret of your OAuth application.
*/
@SuppressWarnings("unchecked")
@JsonIgnore
public Optional clientSecret() {
return (Optional) clientSecret;
}
public final static Builder builder() {
return new Builder();
}
/**
* An access token generated using the above client ID and secret.
*/
public SourceMailchimpOAuth20 withAccessToken(String accessToken) {
Utils.checkNotNull(accessToken, "accessToken");
this.accessToken = accessToken;
return this;
}
/**
* The Client ID of your OAuth application.
*/
public SourceMailchimpOAuth20 withClientId(String clientId) {
Utils.checkNotNull(clientId, "clientId");
this.clientId = Optional.ofNullable(clientId);
return this;
}
/**
* The Client ID of your OAuth application.
*/
public SourceMailchimpOAuth20 withClientId(Optional extends String> clientId) {
Utils.checkNotNull(clientId, "clientId");
this.clientId = clientId;
return this;
}
/**
* The Client Secret of your OAuth application.
*/
public SourceMailchimpOAuth20 withClientSecret(String clientSecret) {
Utils.checkNotNull(clientSecret, "clientSecret");
this.clientSecret = Optional.ofNullable(clientSecret);
return this;
}
/**
* The Client Secret of your OAuth application.
*/
public SourceMailchimpOAuth20 withClientSecret(Optional extends String> clientSecret) {
Utils.checkNotNull(clientSecret, "clientSecret");
this.clientSecret = clientSecret;
return this;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SourceMailchimpOAuth20 other = (SourceMailchimpOAuth20) o;
return
java.util.Objects.deepEquals(this.accessToken, other.accessToken) &&
java.util.Objects.deepEquals(this.authType, other.authType) &&
java.util.Objects.deepEquals(this.clientId, other.clientId) &&
java.util.Objects.deepEquals(this.clientSecret, other.clientSecret);
}
@Override
public int hashCode() {
return java.util.Objects.hash(
accessToken,
authType,
clientId,
clientSecret);
}
@Override
public String toString() {
return Utils.toString(SourceMailchimpOAuth20.class,
"accessToken", accessToken,
"authType", authType,
"clientId", clientId,
"clientSecret", clientSecret);
}
public final static class Builder {
private String accessToken;
private Optional extends String> clientId = Optional.empty();
private Optional extends String> clientSecret = Optional.empty();
private Builder() {
// force use of static builder() method
}
/**
* An access token generated using the above client ID and secret.
*/
public Builder accessToken(String accessToken) {
Utils.checkNotNull(accessToken, "accessToken");
this.accessToken = accessToken;
return this;
}
/**
* The Client ID of your OAuth application.
*/
public Builder clientId(String clientId) {
Utils.checkNotNull(clientId, "clientId");
this.clientId = Optional.ofNullable(clientId);
return this;
}
/**
* The Client ID of your OAuth application.
*/
public Builder clientId(Optional extends String> clientId) {
Utils.checkNotNull(clientId, "clientId");
this.clientId = clientId;
return this;
}
/**
* The Client Secret of your OAuth application.
*/
public Builder clientSecret(String clientSecret) {
Utils.checkNotNull(clientSecret, "clientSecret");
this.clientSecret = Optional.ofNullable(clientSecret);
return this;
}
/**
* The Client Secret of your OAuth application.
*/
public Builder clientSecret(Optional extends String> clientSecret) {
Utils.checkNotNull(clientSecret, "clientSecret");
this.clientSecret = clientSecret;
return this;
}
public SourceMailchimpOAuth20 build() {
return new SourceMailchimpOAuth20(
accessToken,
clientId,
clientSecret);
}
private static final LazySingletonValue _SINGLETON_VALUE_AuthType =
new LazySingletonValue<>(
"auth_type",
"\"oauth2.0\"",
new TypeReference() {});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy