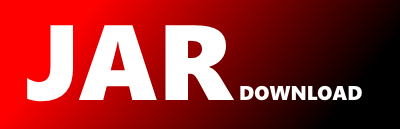
com.airbyte.api.models.shared.SourceOrbit Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of api Show documentation
Show all versions of api Show documentation
SDK enabling Java developers to easily integrate with the Airbyte API.
The newest version!
/*
* Code generated by Speakeasy (https://speakeasyapi.dev). DO NOT EDIT.
*/
package com.airbyte.api.models.shared;
import com.airbyte.api.utils.LazySingletonValue;
import com.airbyte.api.utils.Utils;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonFormat;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.core.type.TypeReference;
import java.io.InputStream;
import java.lang.Deprecated;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.util.Optional;
public class SourceOrbit {
/**
* Authorizes you to work with Orbit workspaces associated with the token.
*/
@JsonProperty("api_token")
private String apiToken;
@JsonProperty("sourceType")
private Orbit sourceType;
/**
* Date in the format 2022-06-26. Only load members whose last activities are after this date.
*/
@JsonInclude(Include.NON_ABSENT)
@JsonProperty("start_date")
private Optional extends String> startDate;
/**
* The unique name of the workspace that your API token is associated with.
*/
@JsonProperty("workspace")
private String workspace;
@JsonCreator
public SourceOrbit(
@JsonProperty("api_token") String apiToken,
@JsonProperty("start_date") Optional extends String> startDate,
@JsonProperty("workspace") String workspace) {
Utils.checkNotNull(apiToken, "apiToken");
Utils.checkNotNull(startDate, "startDate");
Utils.checkNotNull(workspace, "workspace");
this.apiToken = apiToken;
this.sourceType = Builder._SINGLETON_VALUE_SourceType.value();
this.startDate = startDate;
this.workspace = workspace;
}
public SourceOrbit(
String apiToken,
String workspace) {
this(apiToken, Optional.empty(), workspace);
}
/**
* Authorizes you to work with Orbit workspaces associated with the token.
*/
@JsonIgnore
public String apiToken() {
return apiToken;
}
@JsonIgnore
public Orbit sourceType() {
return sourceType;
}
/**
* Date in the format 2022-06-26. Only load members whose last activities are after this date.
*/
@SuppressWarnings("unchecked")
@JsonIgnore
public Optional startDate() {
return (Optional) startDate;
}
/**
* The unique name of the workspace that your API token is associated with.
*/
@JsonIgnore
public String workspace() {
return workspace;
}
public final static Builder builder() {
return new Builder();
}
/**
* Authorizes you to work with Orbit workspaces associated with the token.
*/
public SourceOrbit withApiToken(String apiToken) {
Utils.checkNotNull(apiToken, "apiToken");
this.apiToken = apiToken;
return this;
}
/**
* Date in the format 2022-06-26. Only load members whose last activities are after this date.
*/
public SourceOrbit withStartDate(String startDate) {
Utils.checkNotNull(startDate, "startDate");
this.startDate = Optional.ofNullable(startDate);
return this;
}
/**
* Date in the format 2022-06-26. Only load members whose last activities are after this date.
*/
public SourceOrbit withStartDate(Optional extends String> startDate) {
Utils.checkNotNull(startDate, "startDate");
this.startDate = startDate;
return this;
}
/**
* The unique name of the workspace that your API token is associated with.
*/
public SourceOrbit withWorkspace(String workspace) {
Utils.checkNotNull(workspace, "workspace");
this.workspace = workspace;
return this;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SourceOrbit other = (SourceOrbit) o;
return
java.util.Objects.deepEquals(this.apiToken, other.apiToken) &&
java.util.Objects.deepEquals(this.sourceType, other.sourceType) &&
java.util.Objects.deepEquals(this.startDate, other.startDate) &&
java.util.Objects.deepEquals(this.workspace, other.workspace);
}
@Override
public int hashCode() {
return java.util.Objects.hash(
apiToken,
sourceType,
startDate,
workspace);
}
@Override
public String toString() {
return Utils.toString(SourceOrbit.class,
"apiToken", apiToken,
"sourceType", sourceType,
"startDate", startDate,
"workspace", workspace);
}
public final static class Builder {
private String apiToken;
private Optional extends String> startDate = Optional.empty();
private String workspace;
private Builder() {
// force use of static builder() method
}
/**
* Authorizes you to work with Orbit workspaces associated with the token.
*/
public Builder apiToken(String apiToken) {
Utils.checkNotNull(apiToken, "apiToken");
this.apiToken = apiToken;
return this;
}
/**
* Date in the format 2022-06-26. Only load members whose last activities are after this date.
*/
public Builder startDate(String startDate) {
Utils.checkNotNull(startDate, "startDate");
this.startDate = Optional.ofNullable(startDate);
return this;
}
/**
* Date in the format 2022-06-26. Only load members whose last activities are after this date.
*/
public Builder startDate(Optional extends String> startDate) {
Utils.checkNotNull(startDate, "startDate");
this.startDate = startDate;
return this;
}
/**
* The unique name of the workspace that your API token is associated with.
*/
public Builder workspace(String workspace) {
Utils.checkNotNull(workspace, "workspace");
this.workspace = workspace;
return this;
}
public SourceOrbit build() {
return new SourceOrbit(
apiToken,
startDate,
workspace);
}
private static final LazySingletonValue _SINGLETON_VALUE_SourceType =
new LazySingletonValue<>(
"sourceType",
"\"orbit\"",
new TypeReference() {});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy