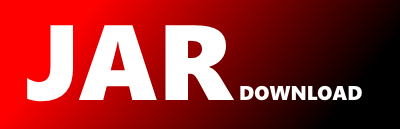
com.airbyte.api.models.shared.SourcePexelsApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of api Show documentation
Show all versions of api Show documentation
SDK enabling Java developers to easily integrate with the Airbyte API.
The newest version!
/*
* Code generated by Speakeasy (https://speakeasyapi.dev). DO NOT EDIT.
*/
package com.airbyte.api.models.shared;
import com.airbyte.api.utils.LazySingletonValue;
import com.airbyte.api.utils.Utils;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonFormat;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.core.type.TypeReference;
import java.io.InputStream;
import java.lang.Deprecated;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.util.Optional;
public class SourcePexelsApi {
/**
* API key is required to access pexels api, For getting your's goto https://www.pexels.com/api/documentation and create account for free.
*/
@JsonProperty("api_key")
private String apiKey;
/**
* Optional, Desired photo color. Supported colors red, orange, yellow, green, turquoise, blue, violet, pink, brown, black, gray, white or any hexidecimal color code.
*/
@JsonInclude(Include.NON_ABSENT)
@JsonProperty("color")
private Optional extends String> color;
/**
* Optional, The locale of the search you are performing. The current supported locales are 'en-US' 'pt-BR' 'es-ES' 'ca-ES' 'de-DE' 'it-IT' 'fr-FR' 'sv-SE' 'id-ID' 'pl-PL' 'ja-JP' 'zh-TW' 'zh-CN' 'ko-KR' 'th-TH' 'nl-NL' 'hu-HU' 'vi-VN' 'cs-CZ' 'da-DK' 'fi-FI' 'uk-UA' 'el-GR' 'ro-RO' 'nb-NO' 'sk-SK' 'tr-TR' 'ru-RU'.
*/
@JsonInclude(Include.NON_ABSENT)
@JsonProperty("locale")
private Optional extends String> locale;
/**
* Optional, Desired photo orientation. The current supported orientations are landscape, portrait or square
*/
@JsonInclude(Include.NON_ABSENT)
@JsonProperty("orientation")
private Optional extends String> orientation;
/**
* Optional, the search query, Example Ocean, Tigers, Pears, etc.
*/
@JsonProperty("query")
private String query;
/**
* Optional, Minimum photo size. The current supported sizes are large(24MP), medium(12MP) or small(4MP).
*/
@JsonInclude(Include.NON_ABSENT)
@JsonProperty("size")
private Optional extends String> size;
@JsonProperty("sourceType")
private PexelsApi sourceType;
@JsonCreator
public SourcePexelsApi(
@JsonProperty("api_key") String apiKey,
@JsonProperty("color") Optional extends String> color,
@JsonProperty("locale") Optional extends String> locale,
@JsonProperty("orientation") Optional extends String> orientation,
@JsonProperty("query") String query,
@JsonProperty("size") Optional extends String> size) {
Utils.checkNotNull(apiKey, "apiKey");
Utils.checkNotNull(color, "color");
Utils.checkNotNull(locale, "locale");
Utils.checkNotNull(orientation, "orientation");
Utils.checkNotNull(query, "query");
Utils.checkNotNull(size, "size");
this.apiKey = apiKey;
this.color = color;
this.locale = locale;
this.orientation = orientation;
this.query = query;
this.size = size;
this.sourceType = Builder._SINGLETON_VALUE_SourceType.value();
}
public SourcePexelsApi(
String apiKey,
String query) {
this(apiKey, Optional.empty(), Optional.empty(), Optional.empty(), query, Optional.empty());
}
/**
* API key is required to access pexels api, For getting your's goto https://www.pexels.com/api/documentation and create account for free.
*/
@JsonIgnore
public String apiKey() {
return apiKey;
}
/**
* Optional, Desired photo color. Supported colors red, orange, yellow, green, turquoise, blue, violet, pink, brown, black, gray, white or any hexidecimal color code.
*/
@SuppressWarnings("unchecked")
@JsonIgnore
public Optional color() {
return (Optional) color;
}
/**
* Optional, The locale of the search you are performing. The current supported locales are 'en-US' 'pt-BR' 'es-ES' 'ca-ES' 'de-DE' 'it-IT' 'fr-FR' 'sv-SE' 'id-ID' 'pl-PL' 'ja-JP' 'zh-TW' 'zh-CN' 'ko-KR' 'th-TH' 'nl-NL' 'hu-HU' 'vi-VN' 'cs-CZ' 'da-DK' 'fi-FI' 'uk-UA' 'el-GR' 'ro-RO' 'nb-NO' 'sk-SK' 'tr-TR' 'ru-RU'.
*/
@SuppressWarnings("unchecked")
@JsonIgnore
public Optional locale() {
return (Optional) locale;
}
/**
* Optional, Desired photo orientation. The current supported orientations are landscape, portrait or square
*/
@SuppressWarnings("unchecked")
@JsonIgnore
public Optional orientation() {
return (Optional) orientation;
}
/**
* Optional, the search query, Example Ocean, Tigers, Pears, etc.
*/
@JsonIgnore
public String query() {
return query;
}
/**
* Optional, Minimum photo size. The current supported sizes are large(24MP), medium(12MP) or small(4MP).
*/
@SuppressWarnings("unchecked")
@JsonIgnore
public Optional size() {
return (Optional) size;
}
@JsonIgnore
public PexelsApi sourceType() {
return sourceType;
}
public final static Builder builder() {
return new Builder();
}
/**
* API key is required to access pexels api, For getting your's goto https://www.pexels.com/api/documentation and create account for free.
*/
public SourcePexelsApi withApiKey(String apiKey) {
Utils.checkNotNull(apiKey, "apiKey");
this.apiKey = apiKey;
return this;
}
/**
* Optional, Desired photo color. Supported colors red, orange, yellow, green, turquoise, blue, violet, pink, brown, black, gray, white or any hexidecimal color code.
*/
public SourcePexelsApi withColor(String color) {
Utils.checkNotNull(color, "color");
this.color = Optional.ofNullable(color);
return this;
}
/**
* Optional, Desired photo color. Supported colors red, orange, yellow, green, turquoise, blue, violet, pink, brown, black, gray, white or any hexidecimal color code.
*/
public SourcePexelsApi withColor(Optional extends String> color) {
Utils.checkNotNull(color, "color");
this.color = color;
return this;
}
/**
* Optional, The locale of the search you are performing. The current supported locales are 'en-US' 'pt-BR' 'es-ES' 'ca-ES' 'de-DE' 'it-IT' 'fr-FR' 'sv-SE' 'id-ID' 'pl-PL' 'ja-JP' 'zh-TW' 'zh-CN' 'ko-KR' 'th-TH' 'nl-NL' 'hu-HU' 'vi-VN' 'cs-CZ' 'da-DK' 'fi-FI' 'uk-UA' 'el-GR' 'ro-RO' 'nb-NO' 'sk-SK' 'tr-TR' 'ru-RU'.
*/
public SourcePexelsApi withLocale(String locale) {
Utils.checkNotNull(locale, "locale");
this.locale = Optional.ofNullable(locale);
return this;
}
/**
* Optional, The locale of the search you are performing. The current supported locales are 'en-US' 'pt-BR' 'es-ES' 'ca-ES' 'de-DE' 'it-IT' 'fr-FR' 'sv-SE' 'id-ID' 'pl-PL' 'ja-JP' 'zh-TW' 'zh-CN' 'ko-KR' 'th-TH' 'nl-NL' 'hu-HU' 'vi-VN' 'cs-CZ' 'da-DK' 'fi-FI' 'uk-UA' 'el-GR' 'ro-RO' 'nb-NO' 'sk-SK' 'tr-TR' 'ru-RU'.
*/
public SourcePexelsApi withLocale(Optional extends String> locale) {
Utils.checkNotNull(locale, "locale");
this.locale = locale;
return this;
}
/**
* Optional, Desired photo orientation. The current supported orientations are landscape, portrait or square
*/
public SourcePexelsApi withOrientation(String orientation) {
Utils.checkNotNull(orientation, "orientation");
this.orientation = Optional.ofNullable(orientation);
return this;
}
/**
* Optional, Desired photo orientation. The current supported orientations are landscape, portrait or square
*/
public SourcePexelsApi withOrientation(Optional extends String> orientation) {
Utils.checkNotNull(orientation, "orientation");
this.orientation = orientation;
return this;
}
/**
* Optional, the search query, Example Ocean, Tigers, Pears, etc.
*/
public SourcePexelsApi withQuery(String query) {
Utils.checkNotNull(query, "query");
this.query = query;
return this;
}
/**
* Optional, Minimum photo size. The current supported sizes are large(24MP), medium(12MP) or small(4MP).
*/
public SourcePexelsApi withSize(String size) {
Utils.checkNotNull(size, "size");
this.size = Optional.ofNullable(size);
return this;
}
/**
* Optional, Minimum photo size. The current supported sizes are large(24MP), medium(12MP) or small(4MP).
*/
public SourcePexelsApi withSize(Optional extends String> size) {
Utils.checkNotNull(size, "size");
this.size = size;
return this;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SourcePexelsApi other = (SourcePexelsApi) o;
return
java.util.Objects.deepEquals(this.apiKey, other.apiKey) &&
java.util.Objects.deepEquals(this.color, other.color) &&
java.util.Objects.deepEquals(this.locale, other.locale) &&
java.util.Objects.deepEquals(this.orientation, other.orientation) &&
java.util.Objects.deepEquals(this.query, other.query) &&
java.util.Objects.deepEquals(this.size, other.size) &&
java.util.Objects.deepEquals(this.sourceType, other.sourceType);
}
@Override
public int hashCode() {
return java.util.Objects.hash(
apiKey,
color,
locale,
orientation,
query,
size,
sourceType);
}
@Override
public String toString() {
return Utils.toString(SourcePexelsApi.class,
"apiKey", apiKey,
"color", color,
"locale", locale,
"orientation", orientation,
"query", query,
"size", size,
"sourceType", sourceType);
}
public final static class Builder {
private String apiKey;
private Optional extends String> color = Optional.empty();
private Optional extends String> locale = Optional.empty();
private Optional extends String> orientation = Optional.empty();
private String query;
private Optional extends String> size = Optional.empty();
private Builder() {
// force use of static builder() method
}
/**
* API key is required to access pexels api, For getting your's goto https://www.pexels.com/api/documentation and create account for free.
*/
public Builder apiKey(String apiKey) {
Utils.checkNotNull(apiKey, "apiKey");
this.apiKey = apiKey;
return this;
}
/**
* Optional, Desired photo color. Supported colors red, orange, yellow, green, turquoise, blue, violet, pink, brown, black, gray, white or any hexidecimal color code.
*/
public Builder color(String color) {
Utils.checkNotNull(color, "color");
this.color = Optional.ofNullable(color);
return this;
}
/**
* Optional, Desired photo color. Supported colors red, orange, yellow, green, turquoise, blue, violet, pink, brown, black, gray, white or any hexidecimal color code.
*/
public Builder color(Optional extends String> color) {
Utils.checkNotNull(color, "color");
this.color = color;
return this;
}
/**
* Optional, The locale of the search you are performing. The current supported locales are 'en-US' 'pt-BR' 'es-ES' 'ca-ES' 'de-DE' 'it-IT' 'fr-FR' 'sv-SE' 'id-ID' 'pl-PL' 'ja-JP' 'zh-TW' 'zh-CN' 'ko-KR' 'th-TH' 'nl-NL' 'hu-HU' 'vi-VN' 'cs-CZ' 'da-DK' 'fi-FI' 'uk-UA' 'el-GR' 'ro-RO' 'nb-NO' 'sk-SK' 'tr-TR' 'ru-RU'.
*/
public Builder locale(String locale) {
Utils.checkNotNull(locale, "locale");
this.locale = Optional.ofNullable(locale);
return this;
}
/**
* Optional, The locale of the search you are performing. The current supported locales are 'en-US' 'pt-BR' 'es-ES' 'ca-ES' 'de-DE' 'it-IT' 'fr-FR' 'sv-SE' 'id-ID' 'pl-PL' 'ja-JP' 'zh-TW' 'zh-CN' 'ko-KR' 'th-TH' 'nl-NL' 'hu-HU' 'vi-VN' 'cs-CZ' 'da-DK' 'fi-FI' 'uk-UA' 'el-GR' 'ro-RO' 'nb-NO' 'sk-SK' 'tr-TR' 'ru-RU'.
*/
public Builder locale(Optional extends String> locale) {
Utils.checkNotNull(locale, "locale");
this.locale = locale;
return this;
}
/**
* Optional, Desired photo orientation. The current supported orientations are landscape, portrait or square
*/
public Builder orientation(String orientation) {
Utils.checkNotNull(orientation, "orientation");
this.orientation = Optional.ofNullable(orientation);
return this;
}
/**
* Optional, Desired photo orientation. The current supported orientations are landscape, portrait or square
*/
public Builder orientation(Optional extends String> orientation) {
Utils.checkNotNull(orientation, "orientation");
this.orientation = orientation;
return this;
}
/**
* Optional, the search query, Example Ocean, Tigers, Pears, etc.
*/
public Builder query(String query) {
Utils.checkNotNull(query, "query");
this.query = query;
return this;
}
/**
* Optional, Minimum photo size. The current supported sizes are large(24MP), medium(12MP) or small(4MP).
*/
public Builder size(String size) {
Utils.checkNotNull(size, "size");
this.size = Optional.ofNullable(size);
return this;
}
/**
* Optional, Minimum photo size. The current supported sizes are large(24MP), medium(12MP) or small(4MP).
*/
public Builder size(Optional extends String> size) {
Utils.checkNotNull(size, "size");
this.size = size;
return this;
}
public SourcePexelsApi build() {
return new SourcePexelsApi(
apiKey,
color,
locale,
orientation,
query,
size);
}
private static final LazySingletonValue _SINGLETON_VALUE_SourceType =
new LazySingletonValue<>(
"sourceType",
"\"pexels-api\"",
new TypeReference() {});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy