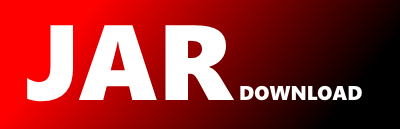
com.airbyte.api.models.shared.SourceSftpBulkCSVFormat Maven / Gradle / Ivy
/*
* Code generated by Speakeasy (https://speakeasyapi.dev). DO NOT EDIT.
*/
package com.airbyte.api.models.shared;
import com.airbyte.api.utils.LazySingletonValue;
import com.airbyte.api.utils.Utils;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonFormat;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.core.type.TypeReference;
import java.io.InputStream;
import java.lang.Deprecated;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.util.Optional;
public class SourceSftpBulkCSVFormat {
/**
* The character delimiting individual cells in the CSV data. This may only be a 1-character string. For tab-delimited data enter '\t'.
*/
@JsonInclude(Include.NON_ABSENT)
@JsonProperty("delimiter")
private Optional extends String> delimiter;
/**
* Whether two quotes in a quoted CSV value denote a single quote in the data.
*/
@JsonInclude(Include.NON_ABSENT)
@JsonProperty("double_quote")
private Optional extends Boolean> doubleQuote;
/**
* The character encoding of the CSV data. Leave blank to default to <strong>UTF8</strong>. See <a href="https://docs.python.org/3/library/codecs.html#standard-encodings" target="_blank">list of python encodings</a> for allowable options.
*/
@JsonInclude(Include.NON_ABSENT)
@JsonProperty("encoding")
private Optional extends String> encoding;
/**
* The character used for escaping special characters. To disallow escaping, leave this field blank.
*/
@JsonInclude(Include.NON_ABSENT)
@JsonProperty("escape_char")
private Optional extends String> escapeChar;
/**
* A set of case-sensitive strings that should be interpreted as false values.
*/
@JsonInclude(Include.NON_ABSENT)
@JsonProperty("false_values")
private Optional extends java.util.List> falseValues;
@JsonInclude(Include.NON_ABSENT)
@JsonProperty("filetype")
private Optional extends SourceSftpBulkSchemasFiletype> filetype;
/**
* How headers will be defined. `User Provided` assumes the CSV does not have a header row and uses the headers provided and `Autogenerated` assumes the CSV does not have a header row and the CDK will generate headers using for `f{i}` where `i` is the index starting from 0. Else, the default behavior is to use the header from the CSV file. If a user wants to autogenerate or provide column names for a CSV having headers, they can skip rows.
*/
@JsonInclude(Include.NON_ABSENT)
@JsonProperty("header_definition")
private Optional extends SourceSftpBulkCSVHeaderDefinition> headerDefinition;
/**
* Whether to ignore errors that occur when the number of fields in the CSV does not match the number of columns in the schema.
*/
@JsonInclude(Include.NON_ABSENT)
@JsonProperty("ignore_errors_on_fields_mismatch")
private Optional extends Boolean> ignoreErrorsOnFieldsMismatch;
/**
* How to infer the types of the columns. If none, inference default to strings.
*/
@JsonInclude(Include.NON_ABSENT)
@JsonProperty("inference_type")
private Optional extends SourceSftpBulkInferenceType> inferenceType;
/**
* A set of case-sensitive strings that should be interpreted as null values. For example, if the value 'NA' should be interpreted as null, enter 'NA' in this field.
*/
@JsonInclude(Include.NON_ABSENT)
@JsonProperty("null_values")
private Optional extends java.util.List> nullValues;
/**
* The character used for quoting CSV values. To disallow quoting, make this field blank.
*/
@JsonInclude(Include.NON_ABSENT)
@JsonProperty("quote_char")
private Optional extends String> quoteChar;
/**
* The number of rows to skip after the header row.
*/
@JsonInclude(Include.NON_ABSENT)
@JsonProperty("skip_rows_after_header")
private Optional extends Long> skipRowsAfterHeader;
/**
* The number of rows to skip before the header row. For example, if the header row is on the 3rd row, enter 2 in this field.
*/
@JsonInclude(Include.NON_ABSENT)
@JsonProperty("skip_rows_before_header")
private Optional extends Long> skipRowsBeforeHeader;
/**
* Whether strings can be interpreted as null values. If true, strings that match the null_values set will be interpreted as null. If false, strings that match the null_values set will be interpreted as the string itself.
*/
@JsonInclude(Include.NON_ABSENT)
@JsonProperty("strings_can_be_null")
private Optional extends Boolean> stringsCanBeNull;
/**
* A set of case-sensitive strings that should be interpreted as true values.
*/
@JsonInclude(Include.NON_ABSENT)
@JsonProperty("true_values")
private Optional extends java.util.List> trueValues;
@JsonCreator
public SourceSftpBulkCSVFormat(
@JsonProperty("delimiter") Optional extends String> delimiter,
@JsonProperty("double_quote") Optional extends Boolean> doubleQuote,
@JsonProperty("encoding") Optional extends String> encoding,
@JsonProperty("escape_char") Optional extends String> escapeChar,
@JsonProperty("false_values") Optional extends java.util.List> falseValues,
@JsonProperty("header_definition") Optional extends SourceSftpBulkCSVHeaderDefinition> headerDefinition,
@JsonProperty("ignore_errors_on_fields_mismatch") Optional extends Boolean> ignoreErrorsOnFieldsMismatch,
@JsonProperty("inference_type") Optional extends SourceSftpBulkInferenceType> inferenceType,
@JsonProperty("null_values") Optional extends java.util.List> nullValues,
@JsonProperty("quote_char") Optional extends String> quoteChar,
@JsonProperty("skip_rows_after_header") Optional extends Long> skipRowsAfterHeader,
@JsonProperty("skip_rows_before_header") Optional extends Long> skipRowsBeforeHeader,
@JsonProperty("strings_can_be_null") Optional extends Boolean> stringsCanBeNull,
@JsonProperty("true_values") Optional extends java.util.List> trueValues) {
Utils.checkNotNull(delimiter, "delimiter");
Utils.checkNotNull(doubleQuote, "doubleQuote");
Utils.checkNotNull(encoding, "encoding");
Utils.checkNotNull(escapeChar, "escapeChar");
Utils.checkNotNull(falseValues, "falseValues");
Utils.checkNotNull(headerDefinition, "headerDefinition");
Utils.checkNotNull(ignoreErrorsOnFieldsMismatch, "ignoreErrorsOnFieldsMismatch");
Utils.checkNotNull(inferenceType, "inferenceType");
Utils.checkNotNull(nullValues, "nullValues");
Utils.checkNotNull(quoteChar, "quoteChar");
Utils.checkNotNull(skipRowsAfterHeader, "skipRowsAfterHeader");
Utils.checkNotNull(skipRowsBeforeHeader, "skipRowsBeforeHeader");
Utils.checkNotNull(stringsCanBeNull, "stringsCanBeNull");
Utils.checkNotNull(trueValues, "trueValues");
this.delimiter = delimiter;
this.doubleQuote = doubleQuote;
this.encoding = encoding;
this.escapeChar = escapeChar;
this.falseValues = falseValues;
this.filetype = Builder._SINGLETON_VALUE_Filetype.value();
this.headerDefinition = headerDefinition;
this.ignoreErrorsOnFieldsMismatch = ignoreErrorsOnFieldsMismatch;
this.inferenceType = inferenceType;
this.nullValues = nullValues;
this.quoteChar = quoteChar;
this.skipRowsAfterHeader = skipRowsAfterHeader;
this.skipRowsBeforeHeader = skipRowsBeforeHeader;
this.stringsCanBeNull = stringsCanBeNull;
this.trueValues = trueValues;
}
public SourceSftpBulkCSVFormat() {
this(Optional.empty(), Optional.empty(), Optional.empty(), Optional.empty(), Optional.empty(), Optional.empty(), Optional.empty(), Optional.empty(), Optional.empty(), Optional.empty(), Optional.empty(), Optional.empty(), Optional.empty(), Optional.empty());
}
/**
* The character delimiting individual cells in the CSV data. This may only be a 1-character string. For tab-delimited data enter '\t'.
*/
@SuppressWarnings("unchecked")
@JsonIgnore
public Optional delimiter() {
return (Optional) delimiter;
}
/**
* Whether two quotes in a quoted CSV value denote a single quote in the data.
*/
@SuppressWarnings("unchecked")
@JsonIgnore
public Optional doubleQuote() {
return (Optional) doubleQuote;
}
/**
* The character encoding of the CSV data. Leave blank to default to <strong>UTF8</strong>. See <a href="https://docs.python.org/3/library/codecs.html#standard-encodings" target="_blank">list of python encodings</a> for allowable options.
*/
@SuppressWarnings("unchecked")
@JsonIgnore
public Optional encoding() {
return (Optional) encoding;
}
/**
* The character used for escaping special characters. To disallow escaping, leave this field blank.
*/
@SuppressWarnings("unchecked")
@JsonIgnore
public Optional escapeChar() {
return (Optional) escapeChar;
}
/**
* A set of case-sensitive strings that should be interpreted as false values.
*/
@SuppressWarnings("unchecked")
@JsonIgnore
public Optional> falseValues() {
return (Optional>) falseValues;
}
@SuppressWarnings("unchecked")
@JsonIgnore
public Optional filetype() {
return (Optional) filetype;
}
/**
* How headers will be defined. `User Provided` assumes the CSV does not have a header row and uses the headers provided and `Autogenerated` assumes the CSV does not have a header row and the CDK will generate headers using for `f{i}` where `i` is the index starting from 0. Else, the default behavior is to use the header from the CSV file. If a user wants to autogenerate or provide column names for a CSV having headers, they can skip rows.
*/
@SuppressWarnings("unchecked")
@JsonIgnore
public Optional headerDefinition() {
return (Optional) headerDefinition;
}
/**
* Whether to ignore errors that occur when the number of fields in the CSV does not match the number of columns in the schema.
*/
@SuppressWarnings("unchecked")
@JsonIgnore
public Optional ignoreErrorsOnFieldsMismatch() {
return (Optional) ignoreErrorsOnFieldsMismatch;
}
/**
* How to infer the types of the columns. If none, inference default to strings.
*/
@SuppressWarnings("unchecked")
@JsonIgnore
public Optional inferenceType() {
return (Optional) inferenceType;
}
/**
* A set of case-sensitive strings that should be interpreted as null values. For example, if the value 'NA' should be interpreted as null, enter 'NA' in this field.
*/
@SuppressWarnings("unchecked")
@JsonIgnore
public Optional> nullValues() {
return (Optional>) nullValues;
}
/**
* The character used for quoting CSV values. To disallow quoting, make this field blank.
*/
@SuppressWarnings("unchecked")
@JsonIgnore
public Optional quoteChar() {
return (Optional) quoteChar;
}
/**
* The number of rows to skip after the header row.
*/
@SuppressWarnings("unchecked")
@JsonIgnore
public Optional skipRowsAfterHeader() {
return (Optional) skipRowsAfterHeader;
}
/**
* The number of rows to skip before the header row. For example, if the header row is on the 3rd row, enter 2 in this field.
*/
@SuppressWarnings("unchecked")
@JsonIgnore
public Optional skipRowsBeforeHeader() {
return (Optional) skipRowsBeforeHeader;
}
/**
* Whether strings can be interpreted as null values. If true, strings that match the null_values set will be interpreted as null. If false, strings that match the null_values set will be interpreted as the string itself.
*/
@SuppressWarnings("unchecked")
@JsonIgnore
public Optional stringsCanBeNull() {
return (Optional) stringsCanBeNull;
}
/**
* A set of case-sensitive strings that should be interpreted as true values.
*/
@SuppressWarnings("unchecked")
@JsonIgnore
public Optional> trueValues() {
return (Optional>) trueValues;
}
public final static Builder builder() {
return new Builder();
}
/**
* The character delimiting individual cells in the CSV data. This may only be a 1-character string. For tab-delimited data enter '\t'.
*/
public SourceSftpBulkCSVFormat withDelimiter(String delimiter) {
Utils.checkNotNull(delimiter, "delimiter");
this.delimiter = Optional.ofNullable(delimiter);
return this;
}
/**
* The character delimiting individual cells in the CSV data. This may only be a 1-character string. For tab-delimited data enter '\t'.
*/
public SourceSftpBulkCSVFormat withDelimiter(Optional extends String> delimiter) {
Utils.checkNotNull(delimiter, "delimiter");
this.delimiter = delimiter;
return this;
}
/**
* Whether two quotes in a quoted CSV value denote a single quote in the data.
*/
public SourceSftpBulkCSVFormat withDoubleQuote(boolean doubleQuote) {
Utils.checkNotNull(doubleQuote, "doubleQuote");
this.doubleQuote = Optional.ofNullable(doubleQuote);
return this;
}
/**
* Whether two quotes in a quoted CSV value denote a single quote in the data.
*/
public SourceSftpBulkCSVFormat withDoubleQuote(Optional extends Boolean> doubleQuote) {
Utils.checkNotNull(doubleQuote, "doubleQuote");
this.doubleQuote = doubleQuote;
return this;
}
/**
* The character encoding of the CSV data. Leave blank to default to <strong>UTF8</strong>. See <a href="https://docs.python.org/3/library/codecs.html#standard-encodings" target="_blank">list of python encodings</a> for allowable options.
*/
public SourceSftpBulkCSVFormat withEncoding(String encoding) {
Utils.checkNotNull(encoding, "encoding");
this.encoding = Optional.ofNullable(encoding);
return this;
}
/**
* The character encoding of the CSV data. Leave blank to default to <strong>UTF8</strong>. See <a href="https://docs.python.org/3/library/codecs.html#standard-encodings" target="_blank">list of python encodings</a> for allowable options.
*/
public SourceSftpBulkCSVFormat withEncoding(Optional extends String> encoding) {
Utils.checkNotNull(encoding, "encoding");
this.encoding = encoding;
return this;
}
/**
* The character used for escaping special characters. To disallow escaping, leave this field blank.
*/
public SourceSftpBulkCSVFormat withEscapeChar(String escapeChar) {
Utils.checkNotNull(escapeChar, "escapeChar");
this.escapeChar = Optional.ofNullable(escapeChar);
return this;
}
/**
* The character used for escaping special characters. To disallow escaping, leave this field blank.
*/
public SourceSftpBulkCSVFormat withEscapeChar(Optional extends String> escapeChar) {
Utils.checkNotNull(escapeChar, "escapeChar");
this.escapeChar = escapeChar;
return this;
}
/**
* A set of case-sensitive strings that should be interpreted as false values.
*/
public SourceSftpBulkCSVFormat withFalseValues(java.util.List falseValues) {
Utils.checkNotNull(falseValues, "falseValues");
this.falseValues = Optional.ofNullable(falseValues);
return this;
}
/**
* A set of case-sensitive strings that should be interpreted as false values.
*/
public SourceSftpBulkCSVFormat withFalseValues(Optional extends java.util.List> falseValues) {
Utils.checkNotNull(falseValues, "falseValues");
this.falseValues = falseValues;
return this;
}
/**
* How headers will be defined. `User Provided` assumes the CSV does not have a header row and uses the headers provided and `Autogenerated` assumes the CSV does not have a header row and the CDK will generate headers using for `f{i}` where `i` is the index starting from 0. Else, the default behavior is to use the header from the CSV file. If a user wants to autogenerate or provide column names for a CSV having headers, they can skip rows.
*/
public SourceSftpBulkCSVFormat withHeaderDefinition(SourceSftpBulkCSVHeaderDefinition headerDefinition) {
Utils.checkNotNull(headerDefinition, "headerDefinition");
this.headerDefinition = Optional.ofNullable(headerDefinition);
return this;
}
/**
* How headers will be defined. `User Provided` assumes the CSV does not have a header row and uses the headers provided and `Autogenerated` assumes the CSV does not have a header row and the CDK will generate headers using for `f{i}` where `i` is the index starting from 0. Else, the default behavior is to use the header from the CSV file. If a user wants to autogenerate or provide column names for a CSV having headers, they can skip rows.
*/
public SourceSftpBulkCSVFormat withHeaderDefinition(Optional extends SourceSftpBulkCSVHeaderDefinition> headerDefinition) {
Utils.checkNotNull(headerDefinition, "headerDefinition");
this.headerDefinition = headerDefinition;
return this;
}
/**
* Whether to ignore errors that occur when the number of fields in the CSV does not match the number of columns in the schema.
*/
public SourceSftpBulkCSVFormat withIgnoreErrorsOnFieldsMismatch(boolean ignoreErrorsOnFieldsMismatch) {
Utils.checkNotNull(ignoreErrorsOnFieldsMismatch, "ignoreErrorsOnFieldsMismatch");
this.ignoreErrorsOnFieldsMismatch = Optional.ofNullable(ignoreErrorsOnFieldsMismatch);
return this;
}
/**
* Whether to ignore errors that occur when the number of fields in the CSV does not match the number of columns in the schema.
*/
public SourceSftpBulkCSVFormat withIgnoreErrorsOnFieldsMismatch(Optional extends Boolean> ignoreErrorsOnFieldsMismatch) {
Utils.checkNotNull(ignoreErrorsOnFieldsMismatch, "ignoreErrorsOnFieldsMismatch");
this.ignoreErrorsOnFieldsMismatch = ignoreErrorsOnFieldsMismatch;
return this;
}
/**
* How to infer the types of the columns. If none, inference default to strings.
*/
public SourceSftpBulkCSVFormat withInferenceType(SourceSftpBulkInferenceType inferenceType) {
Utils.checkNotNull(inferenceType, "inferenceType");
this.inferenceType = Optional.ofNullable(inferenceType);
return this;
}
/**
* How to infer the types of the columns. If none, inference default to strings.
*/
public SourceSftpBulkCSVFormat withInferenceType(Optional extends SourceSftpBulkInferenceType> inferenceType) {
Utils.checkNotNull(inferenceType, "inferenceType");
this.inferenceType = inferenceType;
return this;
}
/**
* A set of case-sensitive strings that should be interpreted as null values. For example, if the value 'NA' should be interpreted as null, enter 'NA' in this field.
*/
public SourceSftpBulkCSVFormat withNullValues(java.util.List nullValues) {
Utils.checkNotNull(nullValues, "nullValues");
this.nullValues = Optional.ofNullable(nullValues);
return this;
}
/**
* A set of case-sensitive strings that should be interpreted as null values. For example, if the value 'NA' should be interpreted as null, enter 'NA' in this field.
*/
public SourceSftpBulkCSVFormat withNullValues(Optional extends java.util.List> nullValues) {
Utils.checkNotNull(nullValues, "nullValues");
this.nullValues = nullValues;
return this;
}
/**
* The character used for quoting CSV values. To disallow quoting, make this field blank.
*/
public SourceSftpBulkCSVFormat withQuoteChar(String quoteChar) {
Utils.checkNotNull(quoteChar, "quoteChar");
this.quoteChar = Optional.ofNullable(quoteChar);
return this;
}
/**
* The character used for quoting CSV values. To disallow quoting, make this field blank.
*/
public SourceSftpBulkCSVFormat withQuoteChar(Optional extends String> quoteChar) {
Utils.checkNotNull(quoteChar, "quoteChar");
this.quoteChar = quoteChar;
return this;
}
/**
* The number of rows to skip after the header row.
*/
public SourceSftpBulkCSVFormat withSkipRowsAfterHeader(long skipRowsAfterHeader) {
Utils.checkNotNull(skipRowsAfterHeader, "skipRowsAfterHeader");
this.skipRowsAfterHeader = Optional.ofNullable(skipRowsAfterHeader);
return this;
}
/**
* The number of rows to skip after the header row.
*/
public SourceSftpBulkCSVFormat withSkipRowsAfterHeader(Optional extends Long> skipRowsAfterHeader) {
Utils.checkNotNull(skipRowsAfterHeader, "skipRowsAfterHeader");
this.skipRowsAfterHeader = skipRowsAfterHeader;
return this;
}
/**
* The number of rows to skip before the header row. For example, if the header row is on the 3rd row, enter 2 in this field.
*/
public SourceSftpBulkCSVFormat withSkipRowsBeforeHeader(long skipRowsBeforeHeader) {
Utils.checkNotNull(skipRowsBeforeHeader, "skipRowsBeforeHeader");
this.skipRowsBeforeHeader = Optional.ofNullable(skipRowsBeforeHeader);
return this;
}
/**
* The number of rows to skip before the header row. For example, if the header row is on the 3rd row, enter 2 in this field.
*/
public SourceSftpBulkCSVFormat withSkipRowsBeforeHeader(Optional extends Long> skipRowsBeforeHeader) {
Utils.checkNotNull(skipRowsBeforeHeader, "skipRowsBeforeHeader");
this.skipRowsBeforeHeader = skipRowsBeforeHeader;
return this;
}
/**
* Whether strings can be interpreted as null values. If true, strings that match the null_values set will be interpreted as null. If false, strings that match the null_values set will be interpreted as the string itself.
*/
public SourceSftpBulkCSVFormat withStringsCanBeNull(boolean stringsCanBeNull) {
Utils.checkNotNull(stringsCanBeNull, "stringsCanBeNull");
this.stringsCanBeNull = Optional.ofNullable(stringsCanBeNull);
return this;
}
/**
* Whether strings can be interpreted as null values. If true, strings that match the null_values set will be interpreted as null. If false, strings that match the null_values set will be interpreted as the string itself.
*/
public SourceSftpBulkCSVFormat withStringsCanBeNull(Optional extends Boolean> stringsCanBeNull) {
Utils.checkNotNull(stringsCanBeNull, "stringsCanBeNull");
this.stringsCanBeNull = stringsCanBeNull;
return this;
}
/**
* A set of case-sensitive strings that should be interpreted as true values.
*/
public SourceSftpBulkCSVFormat withTrueValues(java.util.List trueValues) {
Utils.checkNotNull(trueValues, "trueValues");
this.trueValues = Optional.ofNullable(trueValues);
return this;
}
/**
* A set of case-sensitive strings that should be interpreted as true values.
*/
public SourceSftpBulkCSVFormat withTrueValues(Optional extends java.util.List> trueValues) {
Utils.checkNotNull(trueValues, "trueValues");
this.trueValues = trueValues;
return this;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SourceSftpBulkCSVFormat other = (SourceSftpBulkCSVFormat) o;
return
java.util.Objects.deepEquals(this.delimiter, other.delimiter) &&
java.util.Objects.deepEquals(this.doubleQuote, other.doubleQuote) &&
java.util.Objects.deepEquals(this.encoding, other.encoding) &&
java.util.Objects.deepEquals(this.escapeChar, other.escapeChar) &&
java.util.Objects.deepEquals(this.falseValues, other.falseValues) &&
java.util.Objects.deepEquals(this.filetype, other.filetype) &&
java.util.Objects.deepEquals(this.headerDefinition, other.headerDefinition) &&
java.util.Objects.deepEquals(this.ignoreErrorsOnFieldsMismatch, other.ignoreErrorsOnFieldsMismatch) &&
java.util.Objects.deepEquals(this.inferenceType, other.inferenceType) &&
java.util.Objects.deepEquals(this.nullValues, other.nullValues) &&
java.util.Objects.deepEquals(this.quoteChar, other.quoteChar) &&
java.util.Objects.deepEquals(this.skipRowsAfterHeader, other.skipRowsAfterHeader) &&
java.util.Objects.deepEquals(this.skipRowsBeforeHeader, other.skipRowsBeforeHeader) &&
java.util.Objects.deepEquals(this.stringsCanBeNull, other.stringsCanBeNull) &&
java.util.Objects.deepEquals(this.trueValues, other.trueValues);
}
@Override
public int hashCode() {
return java.util.Objects.hash(
delimiter,
doubleQuote,
encoding,
escapeChar,
falseValues,
filetype,
headerDefinition,
ignoreErrorsOnFieldsMismatch,
inferenceType,
nullValues,
quoteChar,
skipRowsAfterHeader,
skipRowsBeforeHeader,
stringsCanBeNull,
trueValues);
}
@Override
public String toString() {
return Utils.toString(SourceSftpBulkCSVFormat.class,
"delimiter", delimiter,
"doubleQuote", doubleQuote,
"encoding", encoding,
"escapeChar", escapeChar,
"falseValues", falseValues,
"filetype", filetype,
"headerDefinition", headerDefinition,
"ignoreErrorsOnFieldsMismatch", ignoreErrorsOnFieldsMismatch,
"inferenceType", inferenceType,
"nullValues", nullValues,
"quoteChar", quoteChar,
"skipRowsAfterHeader", skipRowsAfterHeader,
"skipRowsBeforeHeader", skipRowsBeforeHeader,
"stringsCanBeNull", stringsCanBeNull,
"trueValues", trueValues);
}
public final static class Builder {
private Optional extends String> delimiter;
private Optional extends Boolean> doubleQuote;
private Optional extends String> encoding;
private Optional extends String> escapeChar = Optional.empty();
private Optional extends java.util.List> falseValues = Optional.empty();
private Optional extends SourceSftpBulkCSVHeaderDefinition> headerDefinition = Optional.empty();
private Optional extends Boolean> ignoreErrorsOnFieldsMismatch;
private Optional extends SourceSftpBulkInferenceType> inferenceType;
private Optional extends java.util.List> nullValues = Optional.empty();
private Optional extends String> quoteChar;
private Optional extends Long> skipRowsAfterHeader;
private Optional extends Long> skipRowsBeforeHeader;
private Optional extends Boolean> stringsCanBeNull;
private Optional extends java.util.List> trueValues = Optional.empty();
private Builder() {
// force use of static builder() method
}
/**
* The character delimiting individual cells in the CSV data. This may only be a 1-character string. For tab-delimited data enter '\t'.
*/
public Builder delimiter(String delimiter) {
Utils.checkNotNull(delimiter, "delimiter");
this.delimiter = Optional.ofNullable(delimiter);
return this;
}
/**
* The character delimiting individual cells in the CSV data. This may only be a 1-character string. For tab-delimited data enter '\t'.
*/
public Builder delimiter(Optional extends String> delimiter) {
Utils.checkNotNull(delimiter, "delimiter");
this.delimiter = delimiter;
return this;
}
/**
* Whether two quotes in a quoted CSV value denote a single quote in the data.
*/
public Builder doubleQuote(boolean doubleQuote) {
Utils.checkNotNull(doubleQuote, "doubleQuote");
this.doubleQuote = Optional.ofNullable(doubleQuote);
return this;
}
/**
* Whether two quotes in a quoted CSV value denote a single quote in the data.
*/
public Builder doubleQuote(Optional extends Boolean> doubleQuote) {
Utils.checkNotNull(doubleQuote, "doubleQuote");
this.doubleQuote = doubleQuote;
return this;
}
/**
* The character encoding of the CSV data. Leave blank to default to <strong>UTF8</strong>. See <a href="https://docs.python.org/3/library/codecs.html#standard-encodings" target="_blank">list of python encodings</a> for allowable options.
*/
public Builder encoding(String encoding) {
Utils.checkNotNull(encoding, "encoding");
this.encoding = Optional.ofNullable(encoding);
return this;
}
/**
* The character encoding of the CSV data. Leave blank to default to <strong>UTF8</strong>. See <a href="https://docs.python.org/3/library/codecs.html#standard-encodings" target="_blank">list of python encodings</a> for allowable options.
*/
public Builder encoding(Optional extends String> encoding) {
Utils.checkNotNull(encoding, "encoding");
this.encoding = encoding;
return this;
}
/**
* The character used for escaping special characters. To disallow escaping, leave this field blank.
*/
public Builder escapeChar(String escapeChar) {
Utils.checkNotNull(escapeChar, "escapeChar");
this.escapeChar = Optional.ofNullable(escapeChar);
return this;
}
/**
* The character used for escaping special characters. To disallow escaping, leave this field blank.
*/
public Builder escapeChar(Optional extends String> escapeChar) {
Utils.checkNotNull(escapeChar, "escapeChar");
this.escapeChar = escapeChar;
return this;
}
/**
* A set of case-sensitive strings that should be interpreted as false values.
*/
public Builder falseValues(java.util.List falseValues) {
Utils.checkNotNull(falseValues, "falseValues");
this.falseValues = Optional.ofNullable(falseValues);
return this;
}
/**
* A set of case-sensitive strings that should be interpreted as false values.
*/
public Builder falseValues(Optional extends java.util.List> falseValues) {
Utils.checkNotNull(falseValues, "falseValues");
this.falseValues = falseValues;
return this;
}
/**
* How headers will be defined. `User Provided` assumes the CSV does not have a header row and uses the headers provided and `Autogenerated` assumes the CSV does not have a header row and the CDK will generate headers using for `f{i}` where `i` is the index starting from 0. Else, the default behavior is to use the header from the CSV file. If a user wants to autogenerate or provide column names for a CSV having headers, they can skip rows.
*/
public Builder headerDefinition(SourceSftpBulkCSVHeaderDefinition headerDefinition) {
Utils.checkNotNull(headerDefinition, "headerDefinition");
this.headerDefinition = Optional.ofNullable(headerDefinition);
return this;
}
/**
* How headers will be defined. `User Provided` assumes the CSV does not have a header row and uses the headers provided and `Autogenerated` assumes the CSV does not have a header row and the CDK will generate headers using for `f{i}` where `i` is the index starting from 0. Else, the default behavior is to use the header from the CSV file. If a user wants to autogenerate or provide column names for a CSV having headers, they can skip rows.
*/
public Builder headerDefinition(Optional extends SourceSftpBulkCSVHeaderDefinition> headerDefinition) {
Utils.checkNotNull(headerDefinition, "headerDefinition");
this.headerDefinition = headerDefinition;
return this;
}
/**
* Whether to ignore errors that occur when the number of fields in the CSV does not match the number of columns in the schema.
*/
public Builder ignoreErrorsOnFieldsMismatch(boolean ignoreErrorsOnFieldsMismatch) {
Utils.checkNotNull(ignoreErrorsOnFieldsMismatch, "ignoreErrorsOnFieldsMismatch");
this.ignoreErrorsOnFieldsMismatch = Optional.ofNullable(ignoreErrorsOnFieldsMismatch);
return this;
}
/**
* Whether to ignore errors that occur when the number of fields in the CSV does not match the number of columns in the schema.
*/
public Builder ignoreErrorsOnFieldsMismatch(Optional extends Boolean> ignoreErrorsOnFieldsMismatch) {
Utils.checkNotNull(ignoreErrorsOnFieldsMismatch, "ignoreErrorsOnFieldsMismatch");
this.ignoreErrorsOnFieldsMismatch = ignoreErrorsOnFieldsMismatch;
return this;
}
/**
* How to infer the types of the columns. If none, inference default to strings.
*/
public Builder inferenceType(SourceSftpBulkInferenceType inferenceType) {
Utils.checkNotNull(inferenceType, "inferenceType");
this.inferenceType = Optional.ofNullable(inferenceType);
return this;
}
/**
* How to infer the types of the columns. If none, inference default to strings.
*/
public Builder inferenceType(Optional extends SourceSftpBulkInferenceType> inferenceType) {
Utils.checkNotNull(inferenceType, "inferenceType");
this.inferenceType = inferenceType;
return this;
}
/**
* A set of case-sensitive strings that should be interpreted as null values. For example, if the value 'NA' should be interpreted as null, enter 'NA' in this field.
*/
public Builder nullValues(java.util.List nullValues) {
Utils.checkNotNull(nullValues, "nullValues");
this.nullValues = Optional.ofNullable(nullValues);
return this;
}
/**
* A set of case-sensitive strings that should be interpreted as null values. For example, if the value 'NA' should be interpreted as null, enter 'NA' in this field.
*/
public Builder nullValues(Optional extends java.util.List> nullValues) {
Utils.checkNotNull(nullValues, "nullValues");
this.nullValues = nullValues;
return this;
}
/**
* The character used for quoting CSV values. To disallow quoting, make this field blank.
*/
public Builder quoteChar(String quoteChar) {
Utils.checkNotNull(quoteChar, "quoteChar");
this.quoteChar = Optional.ofNullable(quoteChar);
return this;
}
/**
* The character used for quoting CSV values. To disallow quoting, make this field blank.
*/
public Builder quoteChar(Optional extends String> quoteChar) {
Utils.checkNotNull(quoteChar, "quoteChar");
this.quoteChar = quoteChar;
return this;
}
/**
* The number of rows to skip after the header row.
*/
public Builder skipRowsAfterHeader(long skipRowsAfterHeader) {
Utils.checkNotNull(skipRowsAfterHeader, "skipRowsAfterHeader");
this.skipRowsAfterHeader = Optional.ofNullable(skipRowsAfterHeader);
return this;
}
/**
* The number of rows to skip after the header row.
*/
public Builder skipRowsAfterHeader(Optional extends Long> skipRowsAfterHeader) {
Utils.checkNotNull(skipRowsAfterHeader, "skipRowsAfterHeader");
this.skipRowsAfterHeader = skipRowsAfterHeader;
return this;
}
/**
* The number of rows to skip before the header row. For example, if the header row is on the 3rd row, enter 2 in this field.
*/
public Builder skipRowsBeforeHeader(long skipRowsBeforeHeader) {
Utils.checkNotNull(skipRowsBeforeHeader, "skipRowsBeforeHeader");
this.skipRowsBeforeHeader = Optional.ofNullable(skipRowsBeforeHeader);
return this;
}
/**
* The number of rows to skip before the header row. For example, if the header row is on the 3rd row, enter 2 in this field.
*/
public Builder skipRowsBeforeHeader(Optional extends Long> skipRowsBeforeHeader) {
Utils.checkNotNull(skipRowsBeforeHeader, "skipRowsBeforeHeader");
this.skipRowsBeforeHeader = skipRowsBeforeHeader;
return this;
}
/**
* Whether strings can be interpreted as null values. If true, strings that match the null_values set will be interpreted as null. If false, strings that match the null_values set will be interpreted as the string itself.
*/
public Builder stringsCanBeNull(boolean stringsCanBeNull) {
Utils.checkNotNull(stringsCanBeNull, "stringsCanBeNull");
this.stringsCanBeNull = Optional.ofNullable(stringsCanBeNull);
return this;
}
/**
* Whether strings can be interpreted as null values. If true, strings that match the null_values set will be interpreted as null. If false, strings that match the null_values set will be interpreted as the string itself.
*/
public Builder stringsCanBeNull(Optional extends Boolean> stringsCanBeNull) {
Utils.checkNotNull(stringsCanBeNull, "stringsCanBeNull");
this.stringsCanBeNull = stringsCanBeNull;
return this;
}
/**
* A set of case-sensitive strings that should be interpreted as true values.
*/
public Builder trueValues(java.util.List trueValues) {
Utils.checkNotNull(trueValues, "trueValues");
this.trueValues = Optional.ofNullable(trueValues);
return this;
}
/**
* A set of case-sensitive strings that should be interpreted as true values.
*/
public Builder trueValues(Optional extends java.util.List> trueValues) {
Utils.checkNotNull(trueValues, "trueValues");
this.trueValues = trueValues;
return this;
}
public SourceSftpBulkCSVFormat build() {
if (delimiter == null) {
delimiter = _SINGLETON_VALUE_Delimiter.value();
}
if (doubleQuote == null) {
doubleQuote = _SINGLETON_VALUE_DoubleQuote.value();
}
if (encoding == null) {
encoding = _SINGLETON_VALUE_Encoding.value();
}
if (ignoreErrorsOnFieldsMismatch == null) {
ignoreErrorsOnFieldsMismatch = _SINGLETON_VALUE_IgnoreErrorsOnFieldsMismatch.value();
}
if (inferenceType == null) {
inferenceType = _SINGLETON_VALUE_InferenceType.value();
}
if (quoteChar == null) {
quoteChar = _SINGLETON_VALUE_QuoteChar.value();
}
if (skipRowsAfterHeader == null) {
skipRowsAfterHeader = _SINGLETON_VALUE_SkipRowsAfterHeader.value();
}
if (skipRowsBeforeHeader == null) {
skipRowsBeforeHeader = _SINGLETON_VALUE_SkipRowsBeforeHeader.value();
}
if (stringsCanBeNull == null) {
stringsCanBeNull = _SINGLETON_VALUE_StringsCanBeNull.value();
}
return new SourceSftpBulkCSVFormat(
delimiter,
doubleQuote,
encoding,
escapeChar,
falseValues,
headerDefinition,
ignoreErrorsOnFieldsMismatch,
inferenceType,
nullValues,
quoteChar,
skipRowsAfterHeader,
skipRowsBeforeHeader,
stringsCanBeNull,
trueValues);
}
private static final LazySingletonValue> _SINGLETON_VALUE_Delimiter =
new LazySingletonValue<>(
"delimiter",
"\",\"",
new TypeReference>() {});
private static final LazySingletonValue> _SINGLETON_VALUE_DoubleQuote =
new LazySingletonValue<>(
"double_quote",
"true",
new TypeReference>() {});
private static final LazySingletonValue> _SINGLETON_VALUE_Encoding =
new LazySingletonValue<>(
"encoding",
"\"utf8\"",
new TypeReference>() {});
private static final LazySingletonValue> _SINGLETON_VALUE_Filetype =
new LazySingletonValue<>(
"filetype",
"\"csv\"",
new TypeReference>() {});
private static final LazySingletonValue> _SINGLETON_VALUE_IgnoreErrorsOnFieldsMismatch =
new LazySingletonValue<>(
"ignore_errors_on_fields_mismatch",
"false",
new TypeReference>() {});
private static final LazySingletonValue> _SINGLETON_VALUE_InferenceType =
new LazySingletonValue<>(
"inference_type",
"\"None\"",
new TypeReference>() {});
private static final LazySingletonValue> _SINGLETON_VALUE_QuoteChar =
new LazySingletonValue<>(
"quote_char",
"\"\\\"\"",
new TypeReference>() {});
private static final LazySingletonValue> _SINGLETON_VALUE_SkipRowsAfterHeader =
new LazySingletonValue<>(
"skip_rows_after_header",
"0",
new TypeReference>() {});
private static final LazySingletonValue> _SINGLETON_VALUE_SkipRowsBeforeHeader =
new LazySingletonValue<>(
"skip_rows_before_header",
"0",
new TypeReference>() {});
private static final LazySingletonValue> _SINGLETON_VALUE_StringsCanBeNull =
new LazySingletonValue<>(
"strings_can_be_null",
"true",
new TypeReference>() {});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy