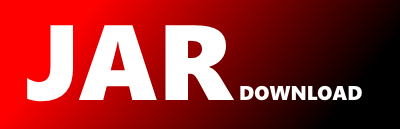
com.airlenet.integration.webapp.mvc.ArgumentResolverResorter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of play-integration-webapp Show documentation
Show all versions of play-integration-webapp Show documentation
The Webapp of Play Intergation.
The newest version!
package com.airlenet.integration.webapp.mvc;
import java.util.ArrayList;
import java.util.List;
import org.springframework.beans.BeansException;
import org.springframework.beans.factory.InitializingBean;
import org.springframework.beans.factory.ObjectFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.context.ApplicationContext;
import org.springframework.context.ApplicationContextAware;
import org.springframework.core.convert.ConversionService;
import org.springframework.stereotype.Component;
import org.springframework.web.method.annotation.ModelAttributeMethodProcessor;
import org.springframework.web.method.support.HandlerMethodArgumentResolver;
import org.springframework.web.servlet.mvc.method.annotation.RequestMappingHandlerAdapter;
import com.airlenet.web.ServletSupport;
@ServletSupport
@Component
public class ArgumentResolverResorter implements InitializingBean, ApplicationContextAware {
@Autowired
@Qualifier("mvcConversionService")
private ObjectFactory conversionService;
@Autowired
private RequestMappingHandlerAdapter handlerAdapter;
private ApplicationContext applicationContext;
@Override
public void afterPropertiesSet() throws Exception {
List argumentResolvers = handlerAdapter.getArgumentResolvers();
List newArgumentResolvers = new ArrayList<>(argumentResolvers);
int indexArg = findIndexOfModelAttributeMethodProcessor(argumentResolvers);
newArgumentResolvers.set(indexArg,
new EntityModelAttributeMethodProcessor(conversionService.getObject(), false, this.applicationContext));
handlerAdapter.setArgumentResolvers(newArgumentResolvers);
List initBinderArgumentResolvers = handlerAdapter
.getInitBinderArgumentResolvers();
List newInitBinderArgumentResolvers = new ArrayList<>(
initBinderArgumentResolvers);
int indexBinder = findIndexOfModelAttributeMethodProcessor(handlerAdapter.getInitBinderArgumentResolvers());
newInitBinderArgumentResolvers.set(indexBinder,
new EntityModelAttributeMethodProcessor(conversionService.getObject(), false, this.applicationContext));
handlerAdapter.setInitBinderArgumentResolvers(newInitBinderArgumentResolvers);
}
private int findIndexOfModelAttributeMethodProcessor(List resolvers) {
for (int i = 0; i < resolvers.size(); i++) {
HandlerMethodArgumentResolver resolver = resolvers.get(i);
if (resolver instanceof ModelAttributeMethodProcessor) {
return i;
}
}
return -1;
}
@Override
public void setApplicationContext(ApplicationContext applicationContext) throws BeansException {
this.applicationContext = applicationContext;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy