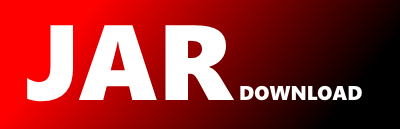
com.airlenet.repo.DatabaseConfigBean Maven / Gradle / Ivy
package com.airlenet.repo;
import com.jolbox.bonecp.BoneCPDataSource;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.jdbc.datasource.LazyConnectionDataSourceProxy;
import javax.sql.DataSource;
import java.util.HashMap;
import java.util.Map;
import java.util.concurrent.TimeUnit;
/**
* Created by lig on 17/2/4.
*/
@Configuration
public class DatabaseConfigBean {
@Value("${jdbc.driver_class?:com.mysql.jdbc.Driver}")
private String jdbcDriverClass;
@Value("${jdbc.url?:jdbc:mysql://localhost:3306/mdm?useUnicode=true&characterEncoding=utf-8&useSSL=false}")
private String jdbcUrl;
@Value("${jdbc.username?:root}")
private String jdbcUsername;
@Value("${jdbc.password?:123456}")
private String jdbcPassword;
@Value("${jdbc.multiple?:false}")
private Boolean multipleDataSource;
@Bean
public DataSource dataSource() {
DataSource mainDataSource = mainDataSource();
if (multipleDataSource) {
Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy