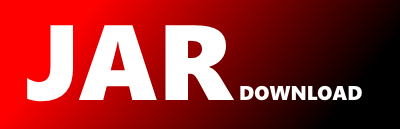
com.airlenet.repo.domain.TreeHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of play-repo Show documentation
Show all versions of play-repo Show documentation
The Repository of Play Framework.
The newest version!
package com.airlenet.repo.domain;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.function.Consumer;
import com.google.common.base.Objects;
import com.google.common.collect.Iterables;
public class TreeHelper {
public static > List listTree(List all) {
return listTree(all, null);
}
public static > List listTree(List all, T parent) {
List result = new ArrayList<>();
if (all != null) {
for (T one : all) {
if ((one.getParent() != null && Objects.equal(one.getParent(), parent))
|| (one.getParent() == null && parent == null)) {
result.add(one);
result.addAll(listTree(all, one));
}
}
}
return result;
}
public static > Tree toTree(T current, List nodes) {
return toTree(current, nodes, null);
}
public static > Tree toSubTree(List nodes) {
Collections.sort(nodes, SortNoComparator.COMPARATOR);
List roots = new ArrayList<>();
if (nodes != null) {
for (T node : nodes) {
if (!Iterables.tryFind(nodes, n -> Objects.equal(n.getParent(), node)).isPresent()) {
roots.add(node);
}
}
}
List> rootNodes = new ArrayList<>();
for (T root : roots) {
rootNodes.add(toNode(root, findDirectSubordinates(root, nodes)));
}
return new TreeImpl<>(rootNodes);
}
public static > Tree toTree(T current, List nodes, Node singleRoot) {
Collections.sort(nodes, SortNoComparator.COMPARATOR);
List> directSubordinates = findDirectSubordinates(current, nodes);
if (current != null) {
Node root = toNode(current, directSubordinates);
directSubordinates = new ArrayList<>();
directSubordinates.add(root);
}
if (singleRoot == null) {
return new TreeImpl<>(directSubordinates);
}
singleRoot.setChildren(directSubordinates);
List> newRoots = new ArrayList<>();
newRoots.add(singleRoot);
Tree tree = new TreeImpl<>(newRoots);
return tree;
}
public static > void visitNodes(List extends Node> nodes,
Consumer> consumer) {
if (nodes != null) {
nodes.forEach((node) -> {
consumer.accept(node);
visitNodes(node.getChildren(), consumer);
});
}
}
public static > List> findDirectSubordinates(T root, List allChildren) {
List> nodes = new ArrayList<>();
for (T entity : allChildren) {
if (Objects.equal(entity.getParent(), root)) {
nodes.add(toNode(entity, findDirectSubordinates(entity, allChildren)));
}
}
return nodes;
}
public static > Node toNode(T entity, List> children) {
Node node = new Node<>();
node.setData(entity);
if (children != null) {
Collections.sort(children, NodeComparator.COMPARATOR);
node.setChildren(children);
}
return node;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy