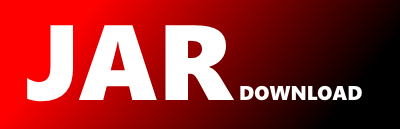
com.aiwiown.face.ApiResponse Maven / Gradle / Ivy
package com.aiwiown.face;
import com.aiwiown.face.internal.mapping.ApiField;
import com.aiwiown.face.internal.util.StringUtils;
import java.io.Serializable;
import java.util.Map;
/**
* API基础响应信息。
*
* @author fengsheng
*/
public abstract class ApiResponse implements Serializable {
private static final long serialVersionUID = 5014379068811962022L;
@ApiField("request_id")
private String requestId;
@ApiField("time_used")
private Integer timeUsed;
@ApiField("error_message")
private String errorMessage;
@ApiField("error")
private String error;
/**
* 当error_message,error值不为空时才会有值
* faceid不同产品返回的错误信息会返回到不同的字段上(error_message,error)
* 统一处理错误code到当前字段
*/
private String code;
/**
* message code对应的各个国家的语言信息
*/
private String message;
private String body;
private Map params;
private Map paramsByteArr;
public boolean isSuccess() {
if (StringUtils.isEmpty(this.errorMessage) && StringUtils.isEmpty(this.error)) {
return true;
}
return false;
}
public String getRequestId() {
return requestId;
}
public void setRequestId(String requestId) {
this.requestId = requestId;
}
public Integer getTimeUsed() {
return timeUsed;
}
public void setTimeUsed(Integer timeUsed) {
this.timeUsed = timeUsed;
}
public String getErrorMessage() {
return errorMessage;
}
public void setErrorMessage(String errorMessage) {
this.errorMessage = errorMessage;
}
public String getBody() {
return body;
}
public void setBody(String body) {
this.body = body;
}
public Map getParams() {
return params;
}
public void setParams(Map params) {
this.params = params;
}
public Map getParamsByteArr() {
return paramsByteArr;
}
public void setParamsByteArr(Map paramsByteArr) {
this.paramsByteArr = paramsByteArr;
}
public String getError() {
return error;
}
public void setError(String error) {
this.error = error;
}
public String getCode() {
return code;
}
public void setCode(String code) {
this.code = code;
}
public String getMessage() {
return message;
}
public void setMessage(String message) {
this.message = message;
}
void build() {
if (isSuccess()) {
return;
}
if (!StringUtils.isEmpty(error)) {
this.code = error;
}
if (!StringUtils.isEmpty(errorMessage)) {
this.code = errorMessage;
}
this.message = ApiConstants.getCodeMessage(this.code);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy