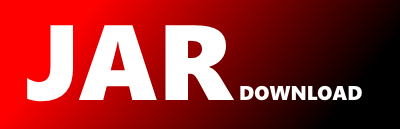
com.aiwiown.face.domain.beautify.BeautifyModel Maven / Gradle / Ivy
package com.aiwiown.face.domain.beautify;
import com.aiwiown.face.ApiObject;
import com.aiwiown.face.internal.mapping.ApiField;
import java.io.File;
/**
* @ClassName : BeautifyModel
* @Description :
* @Author : dbin0123
* @Date: 2020-03-15 15:42
*/
public class BeautifyModel extends ApiObject {
private static final long serialVersionUID = -271577525039536020L;
/**
* 图片的 URL
*/
@ApiField("image_url")
private String imageUrl;
/**
* 一个图片,二进制文件,需要用post multipart/form-data的方式上传
*/
@ApiField(value = "image_file", isFile = true)
private File imageFile;
/**
* base64 编码的二进制图片数据
* 如果同时传入了 image_url、image_file 和 image_base64 参数,本API使用顺序为 image_file 优先,image_url 最低。
*/
@ApiField("image_base64")
private String imageBase64;
/**
* 美白程度,取值范围[0,100] 0不美白,100代表最高程度 本参数默认值为 100
*/
@ApiField("whitening")
private Integer whitening;
/**
* 磨皮程度,取值范围 [0,100] 0不磨皮,100代表最高程度 本参数默认值为 100
*/
@ApiField("smoothing")
private Integer smoothing;
/**
* 瘦脸程度,取值范围 [0,100] 0无瘦脸效果,100代表最高程度
* 本参数默认值为50
*/
@ApiField("thinface")
private Integer thinface;
/**
* 小脸程度,取值范围 [0,100] 0无小脸效果,100代表最高程度
* 本参数默认值为50
*/
@ApiField("shrink_face")
private Integer shrinkFace;
/**
* 大眼程度,取值范围 [0,100] 0无大眼效果,100代表最高程度
* 本参数默认值为50
*/
@ApiField("enlarge_eye")
private Integer enlargeEye;
/**
* 去眉毛程度,取值范围 [0,100] 0无去眉毛效果,100代表最高程度
* 本参数默认值为50
*/
@ApiField("remove_eyebrow")
private Integer removeEyebrow;
/**
* 滤镜名称,滤镜名称列表见下方 默认无滤镜效果
* FilterType.class
*/
@ApiField("filter_type")
private String filterType;
public String getImageUrl() {
return imageUrl;
}
public void setImageUrl(String imageUrl) {
this.imageUrl = imageUrl;
}
public File getImageFile() {
return imageFile;
}
public void setImageFile(File imageFile) {
this.imageFile = imageFile;
}
public String getImageBase64() {
return imageBase64;
}
public void setImageBase64(String imageBase64) {
this.imageBase64 = imageBase64;
}
public Integer getWhitening() {
return whitening;
}
public void setWhitening(Integer whitening) {
this.whitening = whitening;
}
public Integer getSmoothing() {
return smoothing;
}
public void setSmoothing(Integer smoothing) {
this.smoothing = smoothing;
}
public Integer getThinface() {
return thinface;
}
public void setThinface(Integer thinface) {
this.thinface = thinface;
}
public Integer getShrinkFace() {
return shrinkFace;
}
public void setShrinkFace(Integer shrinkFace) {
this.shrinkFace = shrinkFace;
}
public Integer getEnlargeEye() {
return enlargeEye;
}
public void setEnlargeEye(Integer enlargeEye) {
this.enlargeEye = enlargeEye;
}
public Integer getRemoveEyebrow() {
return removeEyebrow;
}
public void setRemoveEyebrow(Integer removeEyebrow) {
this.removeEyebrow = removeEyebrow;
}
public String getFilterType() {
return filterType;
}
public void setFilterType(String filterType) {
this.filterType = filterType;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy