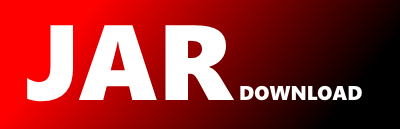
com.aiwiown.face.domain.detect.FaceAttributes Maven / Gradle / Ivy
package com.aiwiown.face.domain.detect;
import com.aiwiown.face.ApiObject;
import com.aiwiown.face.internal.mapping.ApiField;
/**
* 人脸属性特征
*/
public class FaceAttributes extends ApiObject {
private static final long serialVersionUID = -5450451079302547130L;
/**
* 性别分析结果 Male 男性 Female 女性
*/
@ApiField("gender")
private String gender;
/**
* 年龄分析结果
*/
@ApiField("age")
private Integer age;
/**
* 笑容分析结果
*/
@ApiField("smile")
private Threshold smile;
/**
* 是否佩戴眼镜的分析结果 None 不佩戴眼镜 Dark 佩戴墨镜 Normal 佩戴普通眼镜
*/
@ApiField("glass")
private String glass;
/**
* 人脸姿势分析结果
*/
@ApiField("headpose")
private Headpose headpose;
/**
* 人脸模糊分析结果
*/
@ApiField("blur")
private Blur blur;
/**
* 眼睛状态信息
*/
@ApiField("eyestatus")
private EyestatusInfo eyestatus;
/**
* 情绪识别结果
*/
@ApiField("emotion")
private Emotion emotion;
/**
* 人脸质量判断结果
*/
@ApiField("facequality")
private Threshold facequality;
/**
* 人种分析结果
*/
@ApiField("ethnicity")
private String ethnicity;
/**
* 颜值识别结果
*/
@ApiField("beauty")
private Beauty beauty;
/**
* 嘴部状态信息
*/
@ApiField("mouthstatus")
private Mouthstatus mouthstatus;
/**
* 眼球位置与视线方向信息
*/
@ApiField("eyegaze")
private Eyegaze eyegaze;
/**
* 面部特征识别结果
*/
@ApiField("skinstatus")
private Skinstatus skinstatus;
public String getGender() {
return gender;
}
public void setGender(String gender) {
this.gender = gender;
}
public Integer getAge() {
return age;
}
public void setAge(Integer age) {
this.age = age;
}
public Threshold getSmile() {
return smile;
}
public void setSmile(Threshold smile) {
this.smile = smile;
}
public String getGlass() {
return glass;
}
public void setGlass(String glass) {
this.glass = glass;
}
public Headpose getHeadpose() {
return headpose;
}
public void setHeadpose(Headpose headpose) {
this.headpose = headpose;
}
public Blur getBlur() {
return blur;
}
public void setBlur(Blur blur) {
this.blur = blur;
}
public EyestatusInfo getEyestatus() {
return eyestatus;
}
public void setEyestatus(EyestatusInfo eyestatus) {
this.eyestatus = eyestatus;
}
public Emotion getEmotion() {
return emotion;
}
public void setEmotion(Emotion emotion) {
this.emotion = emotion;
}
public Threshold getFacequality() {
return facequality;
}
public void setFacequality(Threshold facequality) {
this.facequality = facequality;
}
public String getEthnicity() {
return ethnicity;
}
public void setEthnicity(String ethnicity) {
this.ethnicity = ethnicity;
}
public Beauty getBeauty() {
return beauty;
}
public void setBeauty(Beauty beauty) {
this.beauty = beauty;
}
public Mouthstatus getMouthstatus() {
return mouthstatus;
}
public void setMouthstatus(Mouthstatus mouthstatus) {
this.mouthstatus = mouthstatus;
}
public Eyegaze getEyegaze() {
return eyegaze;
}
public void setEyegaze(Eyegaze eyegaze) {
this.eyegaze = eyegaze;
}
public Skinstatus getSkinstatus() {
return skinstatus;
}
public void setSkinstatus(Skinstatus skinstatus) {
this.skinstatus = skinstatus;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy