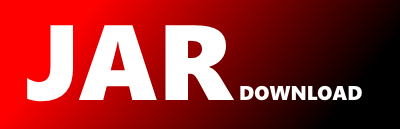
com.aiwiown.face.internal.util.RequestParamUtils Maven / Gradle / Ivy
package com.aiwiown.face.internal.util;
import com.aiwiown.face.ApiObject;
import com.aiwiown.face.internal.mapping.ApiField;
import java.io.File;
import java.lang.reflect.Field;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
/**
* @ClassName : RequestParamUtils
* @Description :
* @Author : dbin0123
* @Date: 2020-03-14 18:26
*/
public class RequestParamUtils {
public static Map getTextParams(ApiObject bizModel) throws IllegalAccessException {
Map param = new HashMap<>();
if (null != bizModel) {
Field[] declaredFields = bizModel.getClass().getDeclaredFields();
if (null != declaredFields && declaredFields.length > 0) {
for (Field field : declaredFields) {
ApiField apiField = field.getAnnotation(ApiField.class);
if (null != apiField && !apiField.isFile()) {
//打开私有访问
field.setAccessible(true);
Object o = field.get(bizModel);
if(Objects.nonNull(o)){
param.put(apiField.value(), String.valueOf(o));
}
}
}
}
}
return param;
}
public static Map getByteArrayParams(ApiObject bizModel) throws IllegalAccessException {
Map param = new HashMap<>();
if (null != bizModel) {
Field[] declaredFields = bizModel.getClass().getDeclaredFields();
if (null != declaredFields && declaredFields.length > 0) {
for (Field field : declaredFields) {
ApiField apiField = field.getAnnotation(ApiField.class);
if (Objects.nonNull(apiField) && apiField.isFile()) {
//打开私有访问
field.setAccessible(true);
Object o = field.get(bizModel);
if (Objects.nonNull(o)) {
if (o instanceof File) {
//文件
param.put(apiField.value(), StreamUtil.file2byte((File) o));
} else if (o instanceof String) {
//base64串(可以使用字符串传递)
String val = (String) o;
if (val.startsWith("http")) {
param.put(apiField.value(), StreamUtil.decode(StreamUtil.imgUrlToBase64Str(val)));
} else {
param.put(apiField.value(), StreamUtil.decode(val));
}
} else if (o instanceof byte[] || o instanceof Byte[]) {
//byte数组
param.put(apiField.value(), (byte[]) o);
}
}
}
}
}
}
return param;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy