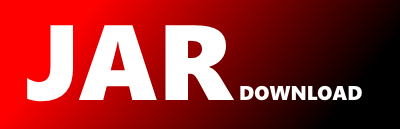
com.aiwiown.face.internal.util.StreamUtil Maven / Gradle / Ivy
/**
* Alipay.com Inc. Copyright (c) 2004-2012 All Rights Reserved.
*/
package com.aiwiown.face.internal.util;
import com.aiwiown.face.internal.util.codec.Base64;
import java.io.*;
import java.net.HttpURLConnection;
import java.net.URL;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
* @author runzhi
*/
public class StreamUtil {
private static final int DEFAULT_BUFFER_SIZE = 8192;
public static void io(InputStream in, OutputStream out) throws IOException {
io(in, out, -1);
}
public static void io(InputStream in, OutputStream out, int bufferSize) throws IOException {
if (bufferSize == -1) {
bufferSize = DEFAULT_BUFFER_SIZE;
}
byte[] buffer = new byte[bufferSize];
int amount;
while ((amount = in.read(buffer)) >= 0) {
out.write(buffer, 0, amount);
}
}
public static void io(Reader in, Writer out) throws IOException {
io(in, out, -1);
}
public static void io(Reader in, Writer out, int bufferSize) throws IOException {
if (bufferSize == -1) {
bufferSize = DEFAULT_BUFFER_SIZE >> 1;
}
char[] buffer = new char[bufferSize];
int amount;
while ((amount = in.read(buffer)) >= 0) {
out.write(buffer, 0, amount);
}
}
public static OutputStream synchronizedOutputStream(OutputStream out) {
return new SynchronizedOutputStream(out);
}
public static OutputStream synchronizedOutputStream(OutputStream out, Object lock) {
return new SynchronizedOutputStream(out, lock);
}
public static String readText(InputStream in) throws IOException {
return readText(in, null, -1);
}
public static String readText(InputStream in, String encoding) throws IOException {
return readText(in, encoding, -1);
}
public static String readText(InputStream in, String encoding, int bufferSize)
throws IOException {
Reader reader = (encoding == null) ? new InputStreamReader(in) : new InputStreamReader(in,
encoding);
return readText(reader, bufferSize);
}
public static String readText(Reader reader) throws IOException {
return readText(reader, -1);
}
public static String readText(Reader reader, int bufferSize) throws IOException {
StringWriter writer = new StringWriter();
io(reader, writer, bufferSize);
return writer.toString();
}
/**
* 将文件转换成byte数组
*
* @param tradeFile
* @return
*/
public static byte[] file2byte(File tradeFile) {
byte[] buffer = null;
try (FileInputStream fis = new FileInputStream(tradeFile);
ByteArrayOutputStream bos = new ByteArrayOutputStream()) {
byte[] b = new byte[1024];
int n;
while ((n = fis.read(b)) != -1) {
bos.write(b, 0, n);
}
fis.close();
bos.close();
buffer = bos.toByteArray();
} catch (Exception e) {
throw new RuntimeException(e);
}
return buffer;
}
public static byte[] decode(String str){
return Base64.decodeBase64String(str);
}
public static String encode(byte[] image) {
return replaceEnter(Base64.encodeBase64String(image));
}
public static String replaceEnter(String str) {
String reg = "[\n-\r]";
Pattern p = Pattern.compile(reg);
Matcher m = p.matcher(str);
return m.replaceAll("");
}
/**
* 图片url转base64字符串
*
* @param imgUrlStr
* @return
*/
public static String imgUrlToBase64Str(String imgUrlStr) {
InputStream inputStream = null;
HttpURLConnection connection = null;
try (ByteArrayOutputStream outputStream = new ByteArrayOutputStream()) {
connection = (HttpURLConnection) new URL(imgUrlStr).openConnection();
connection.setRequestMethod("GET");
connection.setConnectTimeout(5000);
connection.connect();
int responseCode = connection.getResponseCode();
if (301 == responseCode || 302 == responseCode) {
return imgUrlToBase64Str(connection.getHeaderField("Location"));
}
inputStream = connection.getInputStream();
byte[] buffer = new byte[1024];
int len = 0;
while ((len = inputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, len);
}
return encode(outputStream.toByteArray());
} catch (Exception e) {
throw new RuntimeException("图片URL转base64异常!");
} finally {
if (inputStream != null) {
try {
inputStream.close();
} catch (IOException e) {
inputStream = null;
}
}
if (connection != null) {
connection.disconnect();
}
}
}
private static class SynchronizedOutputStream extends OutputStream {
private OutputStream out;
private Object lock;
SynchronizedOutputStream(OutputStream out) {
this(out, out);
}
SynchronizedOutputStream(OutputStream out, Object lock) {
this.out = out;
this.lock = lock;
}
@Override
public void write(int datum) throws IOException {
synchronized (lock) {
out.write(datum);
}
}
@Override
public void write(byte[] data) throws IOException {
synchronized (lock) {
out.write(data);
}
}
@Override
public void write(byte[] data, int offset, int length) throws IOException {
synchronized (lock) {
out.write(data, offset, length);
}
}
@Override
public void flush() throws IOException {
synchronized (lock) {
out.flush();
}
}
@Override
public void close() throws IOException {
synchronized (lock) {
out.close();
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy