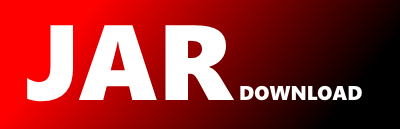
com.aiwiown.face.response.FaceCompareResponse Maven / Gradle / Ivy
package com.aiwiown.face.response;
import com.aiwiown.face.ApiResponse;
import com.aiwiown.face.domain.compare.FaceInfo;
import com.aiwiown.face.domain.compare.Thresholds;
import com.aiwiown.face.internal.mapping.ApiField;
import com.aiwiown.face.internal.mapping.ApiListField;
import java.math.BigDecimal;
import java.util.List;
/**
* @ClassName : FaceCompareResponse
* @Description :
* @Author : dbin0123
* @Date: 2020-03-14 18:49
*/
public class FaceCompareResponse extends ApiResponse {
private static final long serialVersionUID = 5478230332050088392L;
/**
* 比对结果置信度,范围 [0,100],小数点后3位有效数字,数字越大表示两个人脸越可能是同一个人。
* 注:如果传入图片但图片中未检测到人脸,则无法进行比对,本字段不返回。
*/
@ApiField("confidence")
private BigDecimal confidence;
/**
* 一组用于参考的置信度阈值,包含以下三个字段。每个字段的值为一个 [0,100] 的浮点数,小数点后 3 位有效数字。
* 1e-3:误识率为千分之一的置信度阈值;
* 1e-4:误识率为万分之一的置信度阈值;
* 1e-5:误识率为十万分之一的置信度阈值;
* 如果置信值低于“千分之一”阈值则不建议认为是同一个人;如果置信值超过“十万分之一”阈值,则是同一个人的几率非常高。
* 请注意:阈值不是静态的,每次比对返回的阈值不保证相同,所以没有持久化保存阈值的必要,更不要将当前调用返回的 confidence 与之前调用返回的阈值比较。
* 注:如果传入图片但图片中未检测到人脸,则无法进行比对,本字段不返回。
*/
@ApiField("thresholds")
private Thresholds thresholds;
/**
* 通过 image_url1、image_file1 或 image_base64_1 传入的图片在系统中的标识。
*/
@ApiField("image_id1")
private String imageId1;
/**
* 通过 image_url2、image_file2 或 image_base64_2 传入的图片在系统中的标识。
*/
@ApiField("image_id2")
private String imageId2;
/**
* 通过 image_url1、image_file1 或 image_base64_1 传入的图片中检测出的人脸数组
*/
@ApiListField("faces1")
private List faces1;
/**
* 通过 image_url2、image_file2 或 image_base64_2 传入的图片中检测出的人脸数组
*/
@ApiListField("faces2")
private List faces2;
public BigDecimal getConfidence() {
return confidence;
}
public void setConfidence(BigDecimal confidence) {
this.confidence = confidence;
}
public Thresholds getThresholds() {
return thresholds;
}
public void setThresholds(Thresholds thresholds) {
this.thresholds = thresholds;
}
public String getImageId1() {
return imageId1;
}
public void setImageId1(String imageId1) {
this.imageId1 = imageId1;
}
public String getImageId2() {
return imageId2;
}
public void setImageId2(String imageId2) {
this.imageId2 = imageId2;
}
public List getFaces1() {
return faces1;
}
public void setFaces1(List faces1) {
this.faces1 = faces1;
}
public List getFaces2() {
return faces2;
}
public void setFaces2(List faces2) {
this.faces2 = faces2;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy