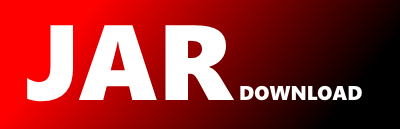
com.ajaxjs.ioc.BeanLoader Maven / Gradle / Ivy
/**
* Copyright sp42 [email protected]
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.ajaxjs.ioc;
import java.io.File;
import java.io.FileFilter;
import java.util.Set;
import com.ajaxjs.ioc.aop.After;
import com.ajaxjs.ioc.aop.Before;
import com.ajaxjs.util.CommonUtil;
import com.ajaxjs.util.ReflectUtil;
import com.ajaxjs.util.resource.AbstractScanner;
import com.ajaxjs.util.resource.ScanClass;
import javassist.CannotCompileException;
import javassist.ClassPool;
import javassist.CtClass;
import javassist.CtField;
import javassist.CtMethod;
import javassist.CtNewMethod;
import javassist.NotFoundException;
/**
* Special for Java Class Scanning
*
* @author sp42 [email protected]
*
*/
public class BeanLoader extends AbstractScanner> {
@Override
public FileFilter getFileFilter() {
return ScanClass.fileFilter;
}
@Override
public void onFileAdding(Set> target, File resource, String packageName) {
String resourcePath = ScanClass.getClassName(resource, packageName);
onJarAdding(target, resourcePath);
}
@SuppressWarnings("unchecked")
@Override
public void onJarAdding(Set> target, String resource) {
ClassPool cp = ClassPool.getDefault();
try {
CtClass cc = cp.get(resource);
doAop(cc);
makeSetter(cc);
// if ("com.ajaxjs.ioc.Hi".equals(resourcePath)) {
// CtField f1 = new CtField(cp.get("com.ajaxjs.ioc.Person"), "person", cc);
// cc.addMethod(CtNewMethod.setter("setPerson", f1));
// }
Class
© 2015 - 2025 Weber Informatics LLC | Privacy Policy