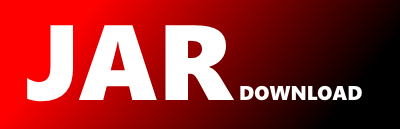
com.ajaxjs.util.resource.ScanClass Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ajaxjs-base Show documentation
Show all versions of ajaxjs-base Show documentation
A pure Java library that provides many tools, utils, and functions.
/**
* Copyright 2015 Sp42 [email protected]
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.ajaxjs.util.resource;
import java.io.File;
import java.io.FileFilter;
import java.util.Set;
import com.ajaxjs.util.ReflectUtil;
/**
* Speical for Java Class Scanning
*
* @author sp42 [email protected]
*
*/
public class ScanClass extends AbstractScanner> {
/**
* 用于查找 class 文件的过滤器
*/
public final static FileFilter fileFilter = file -> file.isDirectory() || file.getName().endsWith(".class");
@Override
public FileFilter getFileFilter() {
return fileFilter;
}
@SuppressWarnings("unchecked")
@Override
public void onFileAdding(Set> target, File resource, String packageName) {
Class clazz = (Class) ReflectUtil.getClassByName(getClassName(resource, packageName));
target.add(clazz);
}
@SuppressWarnings("unchecked")
@Override
public void onJarAdding(Set> target, String resource) {
Class clazz = (Class) ReflectUtil.getClassByName(resource);
target.add(clazz);
}
/**
* 输入包名,获取所有的 classs
*
* @param packageName 包名
* @return 结果
*/
public static Set> scanClass(String packageName) {
return new ScanClass
© 2015 - 2025 Weber Informatics LLC | Privacy Policy