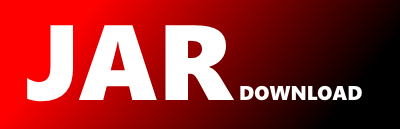
com.ajjpj.afoundation.collection.immutable.MapValueCollection Maven / Gradle / Ivy
package com.ajjpj.afoundation.collection.immutable;
import com.ajjpj.afoundation.collection.ACollectionHelper;
import com.ajjpj.afoundation.function.AFunction1;
import com.ajjpj.afoundation.function.APartialFunction;
import java.util.Collection;
import java.util.Iterator;
import java.util.Objects;
/**
* @author arno
*/
class MapValueCollection extends AbstractACollection> { //TODO other more efficient internal representation?
private final AMap inner;
public MapValueCollection (AMap inner) {
this.inner = inner;
}
@Override protected AList createInternal (Collection elements) {
return AList.create (elements);
}
@Override public ACollection clear () {
return AList.nil();
}
@Override public int size () {
return inner.size ();
}
@Override public boolean contains (V el) {
for (V candidate: this) {
if (Objects.equals (candidate, el)) {
return true;
}
}
return false;
}
@SuppressWarnings ("unchecked")
@Override public ACollection flatten () {
return AList.create ((Iterable) ACollectionHelper.flatten ((Iterable extends Iterable
© 2015 - 2024 Weber Informatics LLC | Privacy Policy