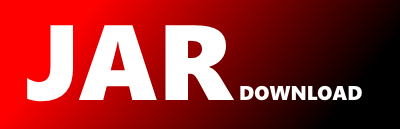
com.ajjpj.afoundation.concurrent.AThreadPoolImpl Maven / Gradle / Ivy
package com.ajjpj.afoundation.concurrent;
import com.ajjpj.afoundation.collection.ACollectionHelper;
import com.ajjpj.afoundation.function.AFunction1;
import com.ajjpj.afoundation.function.AFunction1NoThrow;
import com.ajjpj.afoundation.function.AStatement2NoThrow;
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.*;
/**
* @author arno
*/
class AThreadPoolImpl extends ThreadPoolExecutor implements AThreadPool {
private final boolean shouldInterruptOnTimeout;
final ScheduledThreadPoolExecutor timeoutChecker;
AThreadPoolImpl (int corePoolSize, int maximumPoolSize, long keepAliveTime, TimeUnit unit, BlockingQueue workQueue, ThreadFactory threadFactory, RejectedExecutionHandler handler, boolean shouldInterruptOnTimeout) {
super (corePoolSize, maximumPoolSize, keepAliveTime, unit, workQueue, threadFactory, handler);
this.shouldInterruptOnTimeout = shouldInterruptOnTimeout;
timeoutChecker = new ScheduledThreadPoolExecutor (1, AThreadFactory.createWithRunningPoolNumber ("timeoutChecker", true));
timeoutChecker.setRemoveOnCancelPolicy (true);
}
@Override public void shutdown () {
super.shutdown ();
timeoutChecker.shutdown ();
}
@Override public List shutdownNow () {
timeoutChecker.shutdownNow ();
return super.shutdownNow ();
}
@Override protected RunnableFuture newTaskFor (Runnable runnable, T value) {
return new AFutureImpl<> (this, runnable, value);
}
@Override protected RunnableFuture newTaskFor (Callable callable) {
return new AFutureImpl<> (this, callable);
}
@Override public AFuture submit (Callable task, long timeout, TimeUnit timeoutUnit) {
return register ((AFutureImpl) submit (task), timeout, timeoutUnit);
}
@Override public AFuture submit (Runnable task, T result, long timeout, TimeUnit timeoutUnit) {
return register ((AFutureImpl) submit (task, result), timeout, timeoutUnit);
}
@Override public List> submitAll (List params, final AFunction1 taskFunction, long timeout, TimeUnit timeoutUnit) {
// first transform all tasks to callables to submit either all or none, even if an exception occurs during transformation
final List> callables = new ArrayList<> ();
for (final T param: params) {
callables.add (new Callable () {
@Override public R call () throws Exception {
return taskFunction.apply (param);
}
});
}
final List> result = new ArrayList<> ();
for (Callable c: callables) {
result.add (submit (c, timeout, timeoutUnit));
}
return result;
}
@Override public List> submitAllWithDefaultValue (List params, AFunction1 taskFunction, long timeout, TimeUnit timeoutUnit, final R defaultValue) {
final List> raw = submitAll (params, taskFunction, timeout, timeoutUnit);
return ACollectionHelper.map (raw, new AFunction1NoThrow, AFuture> () {
@Override public AFuture apply (AFuture param) {
return param.withDefaultValue (defaultValue);
}
});
}
private AFutureImpl register (final AFutureImpl f, long timeout, TimeUnit timeoutUnit) {
final Runnable timeoutCanceler = new Runnable () {
@Override public void run () {
f.setTimedOut ();
f.cancel (shouldInterruptOnTimeout);
}
};
final ScheduledFuture> timeoutFuture = timeoutChecker.schedule (timeoutCanceler, timeout, timeoutUnit);
f.onFinished (new AStatement2NoThrow () {
@Override public void apply (T param1, Throwable param2) {
timeoutFuture.cancel (false);
}
});
return f;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy