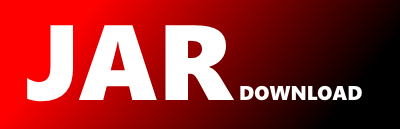
com.alachisoft.ncache.client.CacheConnectionOptions Maven / Gradle / Ivy
package com.alachisoft.ncache.client;
import com.alachisoft.ncache.client.internal.caching.CacheHelper;
import com.alachisoft.ncache.client.internal.util.ClientConfiguration;
import com.alachisoft.ncache.client.internal.util.ConnectionStrings;
import com.alachisoft.ncache.runtime.exceptions.ConfigurationException;
import com.alachisoft.ncache.runtime.util.TimeSpan;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import static tangible.DotNetToJavaStringHelper.isNullOrEmpty;
/**
* Instance of this class can be used to define the parameters at the time of client connection with the cache.
*/
public class CacheConnectionOptions {
//region Fields
private Boolean _loadBalance = true;
private Boolean _enabeKeepAlive = false;
private Boolean _enableClientLogs = false;
private int _port = 9800;
private Integer _commandRetries = 3;
private Integer _connectionRetries = 5;
private TimeSpan _commandRetryInterval = TimeSpan.Zero;
/**
* @hidden
*/
public TimeSpan _clientRequestTimeout = TimeSpan.FromSeconds(90);
private TimeSpan _keepAliveInterval = TimeSpan.FromSeconds(30);
private TimeSpan _retryInterval = TimeSpan.FromSeconds(1); //1 sec
private TimeSpan _connectionTimeout = TimeSpan.FromSeconds(5); //5 sec
private TimeSpan _retryConnectionDelay = TimeSpan.FromMinutes(10); //600 sec or 10 mins
private String _appName;
private String _serverName;
private String _clientBindIP = "";
private String _defaultReadThruProvider = "";
private String _defaultWriteThruProvider = "";
private LogLevel _logLevel = LogLevel.Info;
private IsolationLevel _mode = IsolationLevel.Default;
private ClientCacheSyncMode _clientCacheSyncMode = ClientCacheSyncMode.Optimistic;
private List _serverList = new ArrayList();
private HashMap _dirtyFlags = new HashMap();
private Credentials userCredentials;
private Credentials secondaryUserCredentials;
private String serverName;
private boolean haveBridgeCacheAlias;
private String bridgeCacheAlias;
private boolean fromClientCache;
private boolean isBridgeClient;
private boolean _skipUnAvailableClientCache= true;
private int _retryL1ConnectionInterval= 10;
/**
* @hidden
* @return
*/
public boolean isSkipUnAvailableClientCache() {
return _skipUnAvailableClientCache;
}
/**
* @hidden
* @param skipUnAvailableClientCache
*/
public void setSkipUnAvailableClientCache(boolean skipUnAvailableClientCache) {
_dirtyFlags.put(ConnectionStrings.SKIPUNAVAILABLECLIENTCACHE, true);
this._skipUnAvailableClientCache = skipUnAvailableClientCache;
}
/**
* @hidden
* @return
*/
public int getRetryL1ConnectionInterval() {
return _retryL1ConnectionInterval;
}
/**
* @hidden
* @param retryL1ConnectionInterval
*/
public void setRetryL1ConnectionInterval(int retryL1ConnectionInterval) {
_dirtyFlags.put(ConnectionStrings.RETRYL1CONNECTIONINTERVAL, true);
this._retryL1ConnectionInterval = _retryL1ConnectionInterval;
}
//endregion
//region Public Properties
/**
* Gets the list of {@link ServerInfo} in the cache.
* @return The list of Server info's.
*/
public List getServerList() {
return _serverList;
}
/**
* Sets the list of {@link ServerInfo} in the cache.
* @param value The list of Server info's.
*/
public void setServerList(List value) {
if (value == null) {
return;
}
if (_serverList.size() > 0) {
_serverList.clear();
}
for (ServerInfo temp : value) {
if (!_serverList.contains(temp)) {
_serverList.add(temp);
}
}
_dirtyFlags.put(ConnectionStrings.SERVERLIST, true);
}
/**
* Gets the Isolation level of the cache.
* @return The isolation level of the cache.
*/
public IsolationLevel getIsolationMode() { return _mode; }
/**
* Sets the isolation level of the cache.
* @param value The isolation level of the cache.
*/
public void setIsolationLevel(IsolationLevel value) {
_mode = value;
}
/**
* Gets the name of the default ReadThruProvider.
* @return The name of the default ReadThruProvider.
*/
public String getDefaultReadThruProvider() {
return _defaultReadThruProvider;
}
/**
* Sets the name of the default ReadThruProvider.
* @param value The name of the default ReadThruProvider.
*/
public void setDefaultReadThruProvider(String value) {
_dirtyFlags.put(ConnectionStrings.DEFAULTREADTHRUPROVIDER, true);
_defaultReadThruProvider = value;
}
/**
* Gets the name of default WriteThruProvider.
* @return The name of default WriteThruProvider.
*/
public String getDefaultWriteThruProvider() {
return _defaultWriteThruProvider;
}
/**
* Sets the name of default WriteThruProvider.
* @param value The name of default WriteThruProvider.
*/
public void setDefaultWriteThruProvider(String value) {
_dirtyFlags.put(ConnectionStrings.DEFAULTWRITETHRUPROVIDER, true);
_defaultWriteThruProvider = value;
}
/**
* Gets the IP for the client to be binded with.
* @return The IP for the client to be binded with.
*/
public String getClientBindIP() {
return _clientBindIP;
}
/**
* Sets the IP for the client to be binded with.
* @param value The IP for the client to be binded with.
*/
public void setClientBindIP(String value) {
if (!isNullOrEmpty(value)) {
_dirtyFlags.put(ConnectionStrings.BINDIP, true);
_clientBindIP = value;
}
}
/**
* Gets the appication name.If different client applications are connected to server and because of any issue which results
* in connection failure with server, after the client again establishes connection AppName is used
* to identify these different client applications.
* @return The application name.
*/
public String getAppName() {
return _appName;
}
/**
* Sets the appication name.If different client applications are connected to server and because of any issue which results
* in connection failure with server, after the client again establishes connection AppName is used
* to identify these different client applications.
* @param value The application name.
*/
public void setAppName(String value) {
_appName = value;
}
/**
* When this flag is set, client tries to connect to the optimum server in terms of number of connected clients.
* @return True if loadBalancing enabled otherwise false.
*/
public Boolean getLoadBalance() {
return _loadBalance;
}
/**
* When this flag is set, client tries to connect to the optimum server in terms of number of connected clients.
* @param value The boolean value indicating whether to set LoadBalancing true or false.
*/
public void setLoadBalance(Boolean value) {
_dirtyFlags.put(ConnectionStrings.LOADBALANCE, true);
_loadBalance = value;
}
/**
* Clients operation timeout specified in seconds.
* Clients wait for the response from the server for this time.
* If the response is not received within this time, the operation is not successful.
* @return The request timeout associated with connection options.
*/
public TimeSpan getClientRequestTimeOut() {
return _clientRequestTimeout;
}
/**
* Clients operation timeout specified in seconds.
* Clients wait for the response from the server for this time.
* If the response is not received within this time, the operation is not successful.
* @param value The request timeout to be associated with connection options.
*/
public void setClientRequestTimeOut(TimeSpan value) {
if (value.compareTo(TimeSpan.Zero) >= 0) {
_dirtyFlags.put(ConnectionStrings.CLIENTREQUESTOPTIME, true);
_clientRequestTimeout = value;
}
}
/**
* Client's connection timeout specified in seconds.
* @return The client connecton timeout in form of a {@link TimeSpan}.
*/
public TimeSpan getConnectionTimeout() {
return _connectionTimeout;
}
/**
Client's connection timeout specified in seconds.
* @param value The client connecton timeout in form of a {@link TimeSpan}.
*/
public void setConnectionTimeout(TimeSpan value) {
if (value.compareTo(TimeSpan.Zero) >= 0) {
_dirtyFlags.put(ConnectionStrings.CONNECTIONTIMEOUT, true);
_connectionTimeout = value;
}
}
/**
* Number of tries to re-establish a broken connection between client and server.
* @return An integer indicating the number of retries.
*/
public Integer getConnectionRetries() {
return _connectionRetries;
}
/**
* Number of tries to re-establish a broken connection between client and server.
* @param value An integer indicating the number of retries.
*/
public void setConnectionRetries(Integer value) {
if (value > 0) {
_dirtyFlags.put(ConnectionStrings.CONNECTIONRETRIES, true);
_connectionRetries = value;
}
}
/**
* Time in seconds to wait between two connection retries.
* @return The retry interval in form of {@link TimeSpan}.
*/
public TimeSpan getRetryInterval() {
return _retryInterval;
}
/**
* Time in seconds to wait between two connection retries.
* @param value The retry interval in form of {@link TimeSpan}.
*/
public void setRetryInterval(TimeSpan value) {
_dirtyFlags.put(ConnectionStrings.RETRYINTERVAL, true);
_retryInterval = value;
}
/**
* The time after which client will try to reconnect to the server.
* @return The retry connection delay in form of {@link TimeSpan}.
*/
public TimeSpan getRetryConnectionDelay() {
return _retryConnectionDelay;
}
/**
* The time after which client will try to reconnect to the server.
* @param value The retry connection delay in form of {@link TimeSpan}.
*/
public void setRetryConnectionDelay(TimeSpan value) {
if (value.compareTo(TimeSpan.Zero) >= 0) {
_dirtyFlags.put(ConnectionStrings.RETRYCONNECTIONDELAY, true);
_retryConnectionDelay = value;
}
}
/**
* Gets {@link ClientCacheSyncMode} to specify how the Client cache is synchronized with the cluster caches through events.
* @return The client cache sync mode associated with connection options.
*/
public ClientCacheSyncMode getClientCacheMode() {
return _clientCacheSyncMode;
}
/**
* Sets {@link ClientCacheSyncMode} to specify how the Client cache is synchronized with the cluster caches through events.
* @param value The client cache sync mode to be associated with connection options.
*/
public void setClientCacheMode(ClientCacheSyncMode value) {
_dirtyFlags.put(ConnectionStrings.CACHESYNCMODE, true);
_clientCacheSyncMode = value;
}
/**
* If client application sends request to server for any operation and a response is not received,
* then the number of retries it will make until it gets response is defined here.
* @return An integer indicating the number of command retries.
*/
public Integer getCommandRetries() {
return _commandRetries;
}
/**
* If client application sends request to server for any operation and a response is not received,
* then the number of retries it will make until it gets response is defined here.
* @param value An integer indicating the number of command retries.
*/
public void setCommandRetries(Integer value) {
if (value > 0) {
_dirtyFlags.put(ConnectionStrings.COMMANDRETRIES, true);
_commandRetries = value;
}
}
/**
* In case if client app doesn’t get response against some operation call on server, the command retry
* interval defines the waiting period before the next attempt to send the operation the server is made.
* Type integer which defines seconds.
* @return The command retry interval in form of {@link TimeSpan}.
*/
public TimeSpan getCommandRetryInterval() {
return _commandRetryInterval;
}
/**
* In case if client app doesn’t get response against some operation call on server, the command retry
* interval defines the waiting period before the next attempt to send the operation the server is made.
* Type integer which defines seconds.
* @param value The command retry interval in form of {@link TimeSpan}.
*/
public void setCommandRetryInterval(TimeSpan value) {
if (value.compareTo(TimeSpan.Zero) > 0) {
_dirtyFlags.put(ConnectionStrings.COMMANDRETRYINTERVAL, true);
_commandRetryInterval = value;
}
}
/**
* Gets the keep alive flag.
* @return True if keep alive enabled otherwise false.
*/
public Boolean getEnableKeepAlive() {
return _enabeKeepAlive;
}
/**
* Sets the keep alive flag.
* @param value A boolean value that specifies whether to enable keep alive or not.
*/
public void setEnableKeepAlive(Boolean value) {
_dirtyFlags.put(ConnectionStrings.ENABLEKEEPALIVE, true);
_enabeKeepAlive = value;
}
/**
* Gets the KeepAliveInterval, which will be in effect if the EnabledKeepAlive is set 'true'
* or is specified 'true' from the client configuration.
* @return The keep alive interval in form of {@link TimeSpan}.
*/
public TimeSpan getKeepAliveInterval() {
return _keepAliveInterval;
}
/**
* Sets the KeepAliveInterval, which will be in effect if the EnabledKeepAlive is set 'true'
* or is specified 'true' from the client configuration.
* @param value The keep alive interval in form of {@link TimeSpan}.
*/
public void setKeepAliveInterval(TimeSpan value) {
if (value.compareTo(TimeSpan.FromSeconds(1)) ==-1 || value.compareTo(TimeSpan.FromSeconds(2 * 60 * 60)) ==1) {
_keepAliveInterval = TimeSpan.FromSeconds(30);
} else {
_keepAliveInterval = value;
}
_dirtyFlags.put(ConnectionStrings.KEEPALIVEINTERVAL, true);
}
/**
* @hidden
* @hidden
* @param paramId
* @return
*/
public boolean IsSet(String paramId) {
if (_dirtyFlags == null || _dirtyFlags.isEmpty()) {
return false;
}
if (_dirtyFlags.containsKey(paramId)) {
return (boolean) (_dirtyFlags.get(paramId));
}
return false;
}
/**
* Gets {@link Credentials} for the authentication of connection with the cache.
* This information is required when the security is enabled.
* @return The credentials for the authentication of cache connection.
*/
public Credentials getUserCredentials() {
return userCredentials;
}
/**
* Gets {@link Credentials} for the authentication of connection with the cache.
* This information is required when the security is enabled.
* @param value The credentials for the authentication of cache connection.
*/
public void setUserCredentials(Credentials value) {
userCredentials = value;
}
/**
* Gets the {@link LogLevel} either as Info, Error or Debug.
* @return The log level associated with connection options.
*/
public LogLevel getLogLevel() {
return _logLevel;
}
/**
* Sets the {@link LogLevel} either as Info, Error or Debug.
* @param value The log level to be associated with connection options.
*/
public void setLogLevel(LogLevel value) {
_logLevel = value;
_dirtyFlags.put(ConnectionStrings.LOGLEVEL, true);
}
//endregion
//region Internal Properties
/**
* Gets the flag that specifies whether to create client logs or not.
* @return True if client logs are enabled otherwise false.
*/
public Boolean getEnableClientLogs() {
return _enableClientLogs;
}
/**
* Sets the flag that specifies whether to create client logs or not.
* @param value The boolean value that specifies whether client logs are enabled or not.
*/
public void setEnableClientLogs(Boolean value) {
_enableClientLogs = value;
_dirtyFlags.put(ConnectionStrings.ENABLECLIENTLOGS, true);
}
/**
* @hidden
* @hidden
* @return
*/
public Credentials getSecondaryUserCredentials() {
return secondaryUserCredentials;
}
/**
* @hidden
* @hidden
* @param value
*/
public void setSecondaryUserCredentials(Credentials value) {
secondaryUserCredentials = value;
}
/**
* @hidden
* @hidden
* @return
*/
public Integer getPort() {
if (_serverList.size() > 0) {
return _serverList.get(0).getPort();
}
return _port;
}
void setPort(Integer value) {
_dirtyFlags.put(ConnectionStrings.PORT, true);
_port = value;
}
/**
* @hidden
* @hidden
* @return
*/
public String getServerName() {
return serverName;
}
/**
* @hidden
* @hidden
* @return
*/
public boolean getHaveBridgeCacheAlias() {
return haveBridgeCacheAlias;
}
/**
* @hidden
* @hidden
* @param value
*/
public void setHaveBridgeCacheAlias(boolean value) {
haveBridgeCacheAlias = value;
}
/**
* @hidden
* @hidden
* @return
*/
public String getBridgeCacheAlias() {
return bridgeCacheAlias;
}
/**
* @hidden
* @hidden
* @param value
*/
public void setBridgeCacheAlias(String value) {
bridgeCacheAlias = value;
}
/**
* @hidden
* @hidden
* @return
*/
public boolean getFromClientCache() {
return fromClientCache;
}
/**
* @hidden
* @hidden
* @param value
*/
public void setFromClientCache(boolean value) {
fromClientCache = value;
}
/**
* @hidden
* @hidden
* @return
*/
public boolean getIsBridgeClient() {
return isBridgeClient;
}
/**
* @hidden
* @hidden
* @param value
*/
public void setIsBridgeClient(boolean value) {
isBridgeClient = value;
}
//endregion
//region Clonable
/**
* Creates a new object that is a copy of the current instance.
* @return The cloned object.
*/
public Object clone() {
CacheConnectionOptions _cloneParam = new CacheConnectionOptions();
synchronized (this) {
_cloneParam.setAppName(getAppName());
_cloneParam.setClientBindIP(getClientBindIP());
_cloneParam.setHaveBridgeCacheAlias(getHaveBridgeCacheAlias());
_cloneParam.setClientCacheMode(getClientCacheMode());
_cloneParam.setClientRequestTimeOut(getClientRequestTimeOut());
_cloneParam.setCommandRetries(getCommandRetries());
_cloneParam.setCommandRetryInterval(getCommandRetryInterval());
_cloneParam.setConnectionRetries(getConnectionRetries());
_cloneParam.setConnectionTimeout(getConnectionTimeout());
_cloneParam.setDefaultReadThruProvider(getDefaultReadThruProvider());
_cloneParam.setDefaultWriteThruProvider(getDefaultWriteThruProvider());
_cloneParam.setEnableKeepAlive(getEnableKeepAlive());
_cloneParam.setFromClientCache(getFromClientCache());
_cloneParam.setHaveBridgeCacheAlias(getHaveBridgeCacheAlias());
_cloneParam.setIsBridgeClient(getIsBridgeClient());
_cloneParam.setKeepAliveInterval(getKeepAliveInterval());
_cloneParam.setLoadBalance(getLoadBalance());
_cloneParam.setIsolationLevel(getIsolationMode());
_cloneParam.setPort(getPort());
_cloneParam.setRetryConnectionDelay(getRetryConnectionDelay());
_cloneParam.setRetryInterval(getRetryInterval());
_cloneParam.setSecondaryUserCredentials(getSecondaryUserCredentials());
_cloneParam.setServerList(getServerList());
_cloneParam.serverName = getServerName();
_cloneParam.setUserCredentials(getUserCredentials());
_cloneParam.setEnableClientLogs(getEnableClientLogs());
_cloneParam.setLogLevel(getLogLevel());
_cloneParam.setSkipUnAvailableClientCache(isSkipUnAvailableClientCache());
_cloneParam.setRetryL1ConnectionInterval(getRetryL1ConnectionInterval());
}
return _cloneParam;
}
//endregion
//region Internal Methods
void initialize(String cacheId) throws ConfigurationException {
boolean useDefault = false;
ClientConfiguration config = new ClientConfiguration(cacheId);
int retries = 3;
while (true) {
try {
config.loadConfiguration();
break;
} catch (Exception ie) {
if (--retries == 0) {
useDefault = true;
break;
}
try {
Thread.sleep(500);
} catch (InterruptedException ignored) {
}
}
}
if (!useDefault) {
if (!IsSet(ConnectionStrings.CLIENTREQUESTOPTIME)) {
_clientRequestTimeout = TimeSpan.FromMilliseconds(config.getClientRequestTimeout());
}
if (!IsSet(ConnectionStrings.CONNECTIONTIMEOUT)) {
this._connectionTimeout = TimeSpan.FromMilliseconds(config.getConnectionTimeout());
}
if (!IsSet(ConnectionStrings.CONNECTIONRETRIES)) {
this._connectionRetries = config.getConnectionRetries();
}
if (!IsSet(ConnectionStrings.RETRYINTERVAL)) {
this._retryInterval = TimeSpan.FromMilliseconds(config.getRetryInterval());
}
if (!IsSet(ConnectionStrings.RETRYCONNECTIONDELAY)) {
this._retryConnectionDelay = TimeSpan.FromMinutes(config.getRetryConnectionDelay());
}
if (!IsSet(ConnectionStrings.BINDIP)) {
this._clientBindIP = config.getBindIP();
}
if (!IsSet(ConnectionStrings.DEFAULTREADTHRUPROVIDER)) {
this._defaultReadThruProvider = config.getDefaultReadThru();
}
if (!IsSet(ConnectionStrings.DEFAULTWRITETHRUPROVIDER)) {
this._defaultWriteThruProvider = config.getDefaultWriteThru();
}
if (!IsSet(ConnectionStrings.PORT)) {
this._port = config.getServerPort();
}
if (!IsSet(ConnectionStrings.CACHESYNCMODE)) {
this._clientCacheSyncMode = config.getCacheSyncMode();
}
if (!IsSet(ConnectionStrings.ENABLECLIENTLOGS)) {
this._enableClientLogs = config.getEnableClientLogs();
}
if (!IsSet(ConnectionStrings.LOGLEVEL)) {
this._logLevel = config.getLogLevels();
}
if (!IsSet(ConnectionStrings.LOADBALANCE)) {
this._loadBalance = config.getBalanceNodes();
}
if (!IsSet(ConnectionStrings.ENABLEKEEPALIVE)) {
this._enabeKeepAlive = config.getEnableKeepAlive();
}
if (!IsSet(ConnectionStrings.KEEPALIVEINTERVAL)) {
this._keepAliveInterval = new TimeSpan(0,0,config.getKeepAliveInterval());
}
if (!IsSet(ConnectionStrings.SKIPUNAVAILABLECLIENTCACHE)) {
this._skipUnAvailableClientCache = config.isSkipUnAvailableClientCache();
}
if (!IsSet(ConnectionStrings.RETRYL1CONNECTIONINTERVAL)) {
this._retryL1ConnectionInterval = config.getRetryL1ConnectionInterval();
}
if (getUserCredentials() == null) {
ArrayList list = CacheHelper.LoadCredentialsFromConfig(cacheId);
if (getUserCredentials() == null) {
setUserCredentials(list.get(0));
}
}
}
}
//endregion
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy