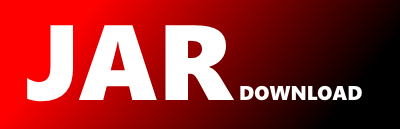
com.alachisoft.ncache.client.CacheItem Maven / Gradle / Ivy
package com.alachisoft.ncache.client;
// Copyright (c) 2020 Alachisoft
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License
import Alachisoft.NCache.Common.BitSet;
import Alachisoft.NCache.Common.Enum.UserObjectType;
import Alachisoft.NCache.Common.Extensibility.ModuleInfo;
import com.alachisoft.ncache.client.internal.caching.*;
import com.alachisoft.ncache.client.internal.datastructure.DataTypeCreator;
import com.alachisoft.ncache.common.caching.EntryType;
import com.alachisoft.ncache.runtime.CacheItemPriority;
import com.alachisoft.ncache.runtime.caching.NamedTagsDictionary;
import com.alachisoft.ncache.runtime.caching.datasource.ResyncOptions;
import com.alachisoft.ncache.runtime.caching.Tag;
import com.alachisoft.ncache.runtime.caching.expiration.Expiration;
import com.alachisoft.ncache.runtime.dependencies.CacheDependency;
import com.alachisoft.ncache.runtime.events.EventDataFilter;
import com.alachisoft.ncache.runtime.events.EventType;
import com.alachisoft.ncache.runtime.util.NCDateTime;
import com.alachisoft.ncache.runtime.exceptions.OperationFailedException;
import java.util.EnumSet;
import java.util.List;
/**
* NCache uses a "key" and "value" structure for storing objects in cache.
* When an object is added in cache it is stored as value and metadata against the specified key.
* This combination of value and metadata is defined as CacheItem in NCache.
* The value of the objects stored in the cache can range from being simple string types to complex objects.
* CacheItem class in NCache has properties that enable you to set metadata for the item to be added in cache
* in an organized way. In scenarios where multiple attributes have to be set while adding an item in cache
* using CacheItem is preferred.Using CacheItem class object removes the problem of using multiple API overloads
* on adding/updating data in cache.You can easily use the basic API overload and add/update data easily using CacheItem.
*/
public class CacheItem implements Cloneable {
///#region Fields
private Object _value;
private Object _currentValue;
// private java.util.Date _absoluteTime = NCDateTime.MaxValue;
private Expiration _expiration = new Expiration();
///#endregion
private CacheItemPriority priority;
private CacheItemVersion version;
///#endregion
///#region Public Properties
private java.util.Date creationTime = null;
private java.util.Date lastModifiedTime = null;
private List tags;
private NamedTagsDictionary namedTags;
private ResyncOptions resyncOptions;
private String group;
private CacheSyncDependency syncDependency;
private CacheDependency dependency;
private int size;
private BitSet flagMap;
private java.util.HashMap queryInfo;
private CacheDataModificationListener cacheItemRemovedListener;
private CacheDataModificationListener cacheItemUpdatedListener;
private EventDataFilter itemUpdatedDataFilter;
private EventDataFilter itemRemovedDataFilter;
private String typeName;
private CacheImpl cacheInstance;
private EntryType entryType;
private boolean isModuleData;
private ModuleInfo moduleInfo;
///#region Constructor
/**
* Initialize new instance of cache item.
*/
public CacheItem() {
setCacheItemPriority(CacheItemPriority.Default);
}
/**
* Initialize new instance of cache item.
* @param value Actual object to be stored in cache.
*/
public CacheItem(Object value) {
_value = value;
setCacheItemPriority(CacheItemPriority.Default);
}
//#endregion
///#region Properties
/**
* Gets the expiration mechanism for cache item.
* @return {@link Expiration} instance that contains info about cache item expiration mechanism.
*/
public final Expiration getExpiration() {
return _expiration;
}
/**
* Sets the expiration mechanism for cache item.
* @param value Expiration object that specifies the expiration mechanism for cache item.
*/
public final void setExpiration(Expiration value) {
_expiration = value;
}
/**
* Gets the relative priority for cache item which is kept under consideration whenever cache starts to free up space and evicts items.
* @return CacheItemPriority associated with cache item.
*/
public final CacheItemPriority getCacheItemPriority() {
return priority;
}
/**
* Sets the relative priority for cache item which is kept under consideration whenever cache starts to free up space and evicts items.
* @param value CacheItemPriority to be associated with cache item.
*/
public final void setCacheItemPriority(CacheItemPriority value) {
priority = value;
}
/**
* Gets the version associated with the cache item.Version is basically a numeric value that is used to represent the version of the cached item which changes
* with every update to an item.
* @return The version associated with the cache item.
*/
public final CacheItemVersion getCacheItemVersion() {
return version;
}
/**
* Sets the version associated with the cache item.Version is basically a numeric value that is used to represent the version of the cached item which changes
* with every update to an item.
* @param value The version to be associated with the cache item
*/
public final void setCacheItemVersion(CacheItemVersion value) {
version = value;
}
/**
* Gets the time when the item was added in cache for the first time.
* @return The date of creation of the cache item.
*/
public final java.util.Date getCreationTime() {
return creationTime;
}
/**
* Gets the lastModifiedTime of the cache item.CacheItem stores the last modified time of the cache item. If an item is
* updated in cache its last modified time is updated as well. Last modified time is checked
* when Least Recently Used based eviction is triggered.
* @return The last modification time of the cache item.
*/
public final java.util.Date getLastModifiedTime() {
return lastModifiedTime;
}
/**
* Gets the tags information associated with the cache item, it can be queried on the basis of Tags provided.
* @return List of Tags associated with cache item.
*/
public final List getTags() {
return tags;
}
/**
* Sets the tags information associated with the cache item, it can be queried on the basis of Tags provided.
* @param value List of Tags to be associated with cache item.
*/
public final void setTags(List value) {
tags = value;
}
/**
* Gets the NamedTags information associated with the cache item, it can be queried on the basis of NamedTags provided.
* @return NamedTags associated with cache item.
*/
public final NamedTagsDictionary getNamedTags() {
return namedTags;
}
/**
* Sets the NamedTags information associated with the cache item, it can be queried on the basis of NamedTags provided.
* @param value NamedTags to be associated with cache item.
*/
public final void setNamedTags(NamedTagsDictionary value) {
namedTags = value;
}
/**
* Gets the ResyncOptions specific to the cache item.
* @return ResyncOptions specific to the cache item.
*/
public final ResyncOptions getResyncOptions() {
return resyncOptions;
}
/**
* Sets the ResyncOptions specific to the cache item.
* @param value ResyncOptions specific to the cache item.
*/
public final void setResyncOptions(ResyncOptions value) {
resyncOptions = value;
}
/**
* Gets the group associated with the cache item. It can be queryed on the basis of Groups.
* @return The group associated with cache item.
*/
public final String getGroup() {
return group;
}
/**
* Sets the group associated with the cache item. It can be queryed on the basis of Groups.
* @param value The group to be associated with cache item.
*/
public final void setGroup(String value) {
group = value;
}
/**
* Synchronizes two separate caches so that an item updated or removed from one cache can
* have the same effect on the synchronized cache.
* For cache sync dependency, an item must exist in cache before another item can be added
* with a dependency on it. This property lets you set Cache sync dependency with a cache item.
* @return CacheSyncDependency associated with cache item.
*/
public final CacheSyncDependency getSyncDependency() {
return syncDependency;
}
/**
* Synchronizes two separate caches so that an item updated or removed from one cache can
* have the same effect on the synchronized cache.
* For cache sync dependency, an item must exist in cache before another item can be added
* with a dependency on it. This property lets you set Cache sync dependency with a cache item.
* @param value CacheSyncDependency to be associated with cache item.
*/
public final void setSyncDependency(CacheSyncDependency value) {
syncDependency = value;
}
/**
* Gets the Cache Dependency instance that contains all dependencies associated with cache item.
* @return The Cache Dependency instance associated with cache item.
*/
public final CacheDependency getDependency() {
return dependency;
}
/**
* Sets the Cache Dependency instance that contains all dependencies associated with cache item.
* @param value The Cache Dependency instance to be associated with cache item.
*/
public final void setDependency(CacheDependency value) {
dependency = value;
}
///#endregion
///#region Internal Properties
void setLastModifiedTime(java.util.Date value) {
lastModifiedTime = value;
}
void setCreationTime(java.util.Date value) {
creationTime = value;
}
/**
* @hidden
* @return
*/
public BitSet getFlagMapInternal() { return flagMap;}
void setFlagMapInternal(BitSet value) {
flagMap = value;
}
java.util.HashMap getQueryInfoInternal() {
return queryInfo;
}
void setQueryInfo(java.util.HashMap value) {
queryInfo = value;
}
CacheDataModificationListener getCacheItemRemovedListener() {
return cacheItemRemovedListener;
}
void setCacheItemRemovedListener(CacheDataModificationListener value) {
cacheItemRemovedListener = value;
}
CacheDataModificationListener getCacheItemUpdatedListener() {
return cacheItemUpdatedListener;
}
void setCacheItemUpdatedListener(CacheDataModificationListener value) {
cacheItemUpdatedListener = value;
}
EventDataFilter getItemUpdatedDataFilter() {
return itemUpdatedDataFilter;
}
void setItemUpdatedDataFilter(EventDataFilter value) {
itemUpdatedDataFilter = value;
}
EventDataFilter getItemRemovedDataFilter() {
return itemRemovedDataFilter;
}
void setItemRemovedDataFilter(EventDataFilter value) {
itemRemovedDataFilter = value;
}
String getTypeName() {
return typeName;
}
/**
* @hidden
* @param value
*/
public void setTypeNameInternal(String value) {
typeName = value;
}
CacheImpl getCacheInstance() {
return cacheInstance;
}
void setCacheInstance(CacheImpl value) {
cacheInstance = value;
}
EntryType getEntryType() {
return entryType;
}
void setEntryType(EntryType value) {
entryType = value;
}
boolean getIsModuleData() {
return isModuleData;
}
void setIsModuleData(boolean value) {
isModuleData = value;
}
ModuleInfo getModuleInfo() {
return moduleInfo;
}
void setModuleInfo(ModuleInfo value) {
moduleInfo = value;
}
///#endregion
///#region Public Methods
/**
* You can use this to notify applications when their objects are updated or removed in the cache.
* Listeners can be registered against event type/types for the key the items is inserted to.
* Listeners are overridden for the same event type/types if called again.
* @param listener Listener to be raised when an item is updated or removed.
* @param eventType Event type/types the listener is registered against.
* @param datafilter Tells whether to receive metadata, data with metadata or none when a notification is triggered.
*/
public final void addCacheDataNotificationListener(CacheDataModificationListener listener, EnumSet eventType, EventDataFilter datafilter) {
if (listener == null) {
return;
}
if ((eventType.contains(EventType.ItemRemoved))) {
setCacheItemRemovedListener(listener);
setItemRemovedDataFilter(datafilter);
}
if (eventType.contains(EventType.ItemUpdated)) {
setCacheItemUpdatedListener(listener);
setItemUpdatedDataFilter(datafilter);
}
}
/**
* Unregisters the user from cache data modification notifications.
* @param listener The listener that is registered for notifications.
* @param eventEnumSet Event type/types the listener is unregistered against.
*/
public void removeCacheDataNotificationListener(CacheDataModificationListener listener, EnumSet eventEnumSet)
{
if( listener == null ) return;
if(eventEnumSet.contains(EventType.ItemRemoved))
{
setCacheItemRemovedListener(listener);
setItemRemovedDataFilter(EventDataFilter.None);
}
if(eventEnumSet.contains(EventType.ItemUpdated))
{
setCacheItemUpdatedListener(listener);
setItemUpdatedDataFilter(EventDataFilter.None);
}
}
/**
* Returns the value stored in the cache item.
* This value must be serializable.
* @param cls Specifies the class of value obtained from the cache item.
* @param Specifies the type of value obtained from the cache item.
* @return Value of the cache item with the class defined.
* @throws OperationFailedException
*/
public final T getValue(Class> cls) throws OperationFailedException {
if (_value instanceof ValueEmissary) {
T instanceofT = null;
if (_currentValue != null)
try {
return (T) _currentValue;
} catch (ClassCastException e) {
//ignore
}
ValueEmissary emissary = (ValueEmissary)((_value instanceof ValueEmissary) ? _value : null);
if (cacheInstance == null)
{
return (T)emissary.getData();
}
switch (emissary.getType())
{
case CacheItem:
_currentValue = cacheInstance.safeDeserialize(emissary.getData(), cacheInstance.getSerializationContext(), flagMap, UserObjectType.CacheItem, cls);
break;
default:
_currentValue = DataTypeCreator.createDataTypeHandle(emissary.getType(), emissary.getKey(), cacheInstance,cls);
break;
}
return (T)_currentValue;
}
return (T)_value;
}
/**
* Sets the value of the cache item.
* @param value object to be stored in cache item.
*/
public void setValue(Object value) {
_value = value;
}
///#endregion
///#region Cloneable Members
/**
* Creates a shallow copy of CacheItem
* @return The cloned object.
*/
public final Object clone() {
CacheItem newItem = new CacheItem();
newItem.setCacheItemRemovedListener(getCacheItemRemovedListener());
newItem.setCacheItemUpdatedListener(getCacheItemUpdatedListener());
newItem.setCreationTime(getCreationTime());
newItem.setDependency(getDependency());
newItem.setExpiration(getExpiration());
newItem.flagMap = flagMap;
newItem.setGroup(getGroup());
newItem.setItemRemovedDataFilter(getItemRemovedDataFilter());
newItem.setItemUpdatedDataFilter(getItemUpdatedDataFilter());
newItem.setTypeNameInternal(getTypeName());
newItem.setLastModifiedTime(getLastModifiedTime());
newItem.setNamedTags(getNamedTags());
newItem.setCacheItemPriority(getCacheItemPriority());
newItem.queryInfo = queryInfo;
newItem.setResyncOptions(getResyncOptions());
newItem.size= size;
newItem.setSyncDependency(getSyncDependency());
newItem.setTags(getTags());
newItem._value = _value;
newItem.setCacheItemVersion(getCacheItemVersion());
newItem.setCacheInstance(getCacheInstance());
newItem.setIsModuleData(getIsModuleData());
return newItem;
}
///#endregion
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy