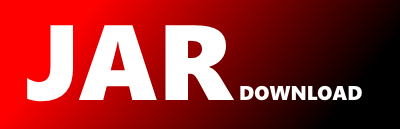
com.alachisoft.ncache.client.CacheManager Maven / Gradle / Ivy
package com.alachisoft.ncache.client;
import Alachisoft.NCache.Caching.CacheFactory;
import Alachisoft.NCache.Common.AppUtil;
import Alachisoft.NCache.Common.Exceptions.ManagementException;
import Alachisoft.NCache.Common.Monitoring.CategoriesConstants;
import Alachisoft.NCache.Common.Monitoring.MetricsPublisher;
import Alachisoft.NCache.Common.Monitoring.MonitoringConfigManager;
import Alachisoft.NCache.Config.Dom.CacheServerConfig;
import Alachisoft.NCache.Management.CacheConfig;
import Alachisoft.NCache.Management.CacheServerModerator;
import Alachisoft.NCache.Management.MetricsTransporterFactoryImpl;
import com.alachisoft.ncache.client.internal.caching.*;
import com.alachisoft.ncache.client.internal.statistics.PerfStatsCollector;
import com.alachisoft.ncache.client.internal.util.ConfigReader;
import com.alachisoft.ncache.client.internal.util.DirectoryUtil;
import Alachisoft.NCache.Common.ErrorHandling.ErrorCodes;
import Alachisoft.NCache.Common.ErrorHandling.ErrorMessages;
import com.alachisoft.ncache.common.monitoring.MetricsTransporterFactory;
import com.alachisoft.ncache.runtime.cachemanagement.CacheContext;
import com.alachisoft.ncache.runtime.cachemanagement.CacheHealth;
import com.alachisoft.ncache.runtime.cachemanagement.ServerNode;
import com.alachisoft.ncache.runtime.caching.ClientInfo;
import com.alachisoft.ncache.runtime.exceptions.CacheException;
import com.alachisoft.ncache.runtime.exceptions.ConfigurationException;
import com.alachisoft.ncache.runtime.exceptions.OperationFailedException;
import com.alachisoft.ncache.runtime.exceptions.OperationNotSupportedException;
import java.net.UnknownHostException;
import java.util.List;
import java.util.Map;
import static tangible.DotNetToJavaStringHelper.isNullOrEmpty;
/**
* Provides and manages the instance of {@link Cache}
*/
public final class CacheManager {
//region Private Fields
private static String _clientCacheID = "";
private static CacheImpl _cache = new CacheImpl();
private static boolean s_exceptions = true;
private static CacheCollection _cacheCollection = new CacheCollection();
private static String _configPath = null;
//endregion
//region Public Properties
/**
* Returns {@link CacheCollection} of the caches initialized within the same application domain.
* @return The collection of caches within the same application domain.
*/
public static CacheCollection getCaches() {
synchronized (_cacheCollection) {
return _cacheCollection;
}
}
/**
* @hidden
* @return
*/
public static boolean getExceptionsEnabled() {
if (_cache != null) {
s_exceptions = _cache.getExceptionEnabled();
}
return s_exceptions;
}
/**
* @hidden
* @param value
*/
public static void setExceptionsEnabled(boolean value) {
s_exceptions = value;
if (_cache != null) {
_cache.setExceptionsEnabled(value);
}
}
/**
* @hidden
* @return
*/
public static String getConfigPath() {
return _configPath;
}
/**
* hidden
* @param path
*/
public static void setConfigPath(String path)
{
_configPath = path;
}
//endregion
//region Public Methods
/**
* Returns an instance of {@link Cache} for this application.
* @param cacheName The identifier for the {@link Cache}.
* @return Instance of {@link Cache}.
* @throws Exception
*/
public static Cache getCache(String cacheName) throws Exception {
if (isNullOrEmpty(cacheName)) throw new IllegalArgumentException("Value cannot be null or empty."+System.lineSeparator()+"Parameter name: cacheName");
return getCache(cacheName, null, null, null);
}
/**
* Returns an instance of {@link Cache} for this application.
* @param cacheName The identifier for the {@link Cache}.
* @param cacheConnectionOptions {@link CacheConnectionOptions} parameters for cache connection.
* @return Instance of {@link Cache}.
* @throws Exception
*/
public static Cache getCache(String cacheName, CacheConnectionOptions cacheConnectionOptions) throws Exception {
if (isNullOrEmpty(cacheName)) throw new IllegalArgumentException("Value cannot be null or empty."+System.lineSeparator()+"Parameter name: cacheName");
if (cacheConnectionOptions == null) throw new IllegalArgumentException("Connection Options");
return getCache(cacheName, cacheConnectionOptions, null, null);
}
/**
* Returns an instance of {@link Cache} for this application.
* @param cacheName The identifier for the {@link Cache}.
* @param cacheConnectionOptions {@link CacheConnectionOptions} parameters for cache connection.
* @param clientCacheName The identifier for the ClientCache.
* @param clientCacheConnectionOptions {@link CacheConnectionOptions} parameters for ClientCache connection.
* @return Instance of {@link Cache}.
* @throws Exception
*/
public static Cache getCache(String cacheName, CacheConnectionOptions cacheConnectionOptions, String clientCacheName, CacheConnectionOptions clientCacheConnectionOptions) throws Exception {
if (isNullOrEmpty(cacheName))
throw new IllegalArgumentException("Value cannot be null or empty."+System.lineSeparator()+"Parameter name: cacheName");
if (clientCacheName != null)
return getCachePrivate(cacheName, cacheConnectionOptions, clientCacheName, clientCacheConnectionOptions);
boolean isPessimistic = false;
tangible.RefObject tempRef_isPessimistic = new tangible.RefObject(isPessimistic);
boolean isClientCache = IsClientCache(cacheName, tempRef_isPessimistic);
isPessimistic = tempRef_isPessimistic.argvalue;
return getCache(cacheName, cacheConnectionOptions, isClientCache, isPessimistic);
}
/**
* Get information of clients connected to each server node in a cache.
* @param cacheName The identifier for the {@link Cache}.
* @param connection {@link CacheConnection} parameters for connection to Cache.
* @return Key value pair of {@link ServerNode} and list of {@link ClientInfo} in a cache.
* @throws ManagementException
*/
private static Map> getCacheClients(String cacheName, CacheConnection connection) throws ManagementException {
if (isNullOrEmpty(cacheName)) {
throw new IllegalArgumentException("cacheName");
}
String serverName = connection != null ? connection.getServer() : "";
int port = connection != null ? connection.getPort() : 0;
return CacheServerModerator.getCacheClients(cacheName.toLowerCase(), serverName, CacheContext.TayzGrid, port);
}
/**
* Get Health status of a cache.
* @param cacheName The identifier for the {@link Cache}.
* @param connection {@link CacheConnection} parameters for connection to Cache.
* @return {@link CacheHealth} containing information cache including {@link com.alachisoft.ncache.runtime.cachemanagement.NodeStatus} for each node in cache.
* @throws ManagementException
*/
private static CacheHealth getCacheHealth(String cacheName, CacheConnection connection) throws ManagementException {
if (isNullOrEmpty(cacheName)) {
throw new IllegalArgumentException("cacheName");
}
String serverName = connection != null ? connection.getServer() : "";
int port = connection != null ? connection.getPort() : 0;
return CacheServerModerator.getCacheHealth(cacheName.toLowerCase(), serverName, CacheContext.TayzGrid, port);
}
/**
* Starts an out-proc cache. The end result is the same as that of starting the
* cache using NCache Manager. It starts the cache on the specified cache server.
* If the Cache Server could not be contacted then it throws a {@link ManagementException} exception.
* It tries to get the Security Parameters from the client.ncconf.
* @param cacheName The identifier for the {@link Cache}.
* @param connection {@link CacheConnection} parameters for connection to Cache.
* @throws ManagementException
* @throws UnknownHostException
* @throws ConfigurationException
*/
public static void startCache(String cacheName, CacheConnection connection) throws ManagementException, UnknownHostException, ConfigurationException {
if (isNullOrEmpty(cacheName)) {
throw new IllegalArgumentException("cacheName");
}
Credentials primaryUserCredentials = null;
Credentials secondaryUserCredentials = null;
if (connection != null) {
if (connection.getUserCredentials() == null) {
List list = CacheHelper.LoadCredentialsFromConfig(cacheName);
primaryUserCredentials = list.get(0);
secondaryUserCredentials = list.get(1);
} else {
primaryUserCredentials = connection.getUserCredentials();
}
}
String serverName = connection != null ? connection.getServer() : "";
int port = connection != null ? connection.getPort() : 8250;
int maxTries = 2;
Credentials credentials = verifyCredentials(primaryUserCredentials, secondaryUserCredentials);
do {
try {
CacheServerModerator.StartCache(cacheName, serverName, port, credentials.getUserID(), credentials.getPassword());
return;
} catch (Exception ex) {
maxTries--;
if (maxTries == 0) {
throw ex;
}
if (secondaryUserCredentials != null) {
credentials = secondaryUserCredentials;
} else {
throw ex;
}
}
} while (maxTries > 0);
}
/**
* Stops an out-proc cache. The end result is the same as that of stoping the
* cache using NCache Manager. It stops the cache only on the same server where the client application is running.
* If the Cache Server could not be contacted then it throws a {@link ManagementException} exception.
* It tries to get the Security Parameters from the client.ncconf.
* @param cacheName The identifier for the {@link Cache}.
* @param connection {@link CacheConnection} parameters for connection to Cache.
* @param gracefullyShutDownNode The flag for graceful node shutdown.
* @throws ManagementException
* @throws UnknownHostException
* @throws ConfigurationException
*/
public static void stopCache(String cacheName, CacheConnection connection, boolean gracefullyShutDownNode) throws ManagementException, UnknownHostException, ConfigurationException {
if (isNullOrEmpty(cacheName)) {
throw new IllegalArgumentException("cacheName");
}
Credentials primaryUserCredentials = null;
Credentials secondaryUserCredentials = null;
if (connection != null) {
if (connection.getUserCredentials() == null) {
java.util.ArrayList list = CacheHelper.LoadCredentialsFromConfig(cacheName);
primaryUserCredentials = list.get(0);
secondaryUserCredentials = list.get(1);
} else {
primaryUserCredentials = connection.getUserCredentials();
}
}
String serverName = connection != null ? connection.getServer() : "";
int port = connection != null ? connection.getPort() : 0;
int maxTries = 2;
Credentials credentials = verifyCredentials(primaryUserCredentials, secondaryUserCredentials);
do {
try {
CacheServerModerator.StopCache(cacheName, serverName, port, credentials.getUserID(), credentials.getPassword(), gracefullyShutDownNode);
return;
} catch (Exception ex) {
maxTries--;
if (maxTries == 0) {
throw ex;
}
if (secondaryUserCredentials != null) {
credentials = secondaryUserCredentials;
} else {
throw ex;
}
}
} while (maxTries > 0);
}
//endregion
//region Internal Methods
/**
* @hidden
* @param cacheName
* @param cacheConnectionOptions
* @param isClientCache
* @param isPessimistic
* @return
* @throws Exception
*/
public static CacheImpl getCache(String cacheName, CacheConnectionOptions cacheConnectionOptions, boolean isClientCache, boolean isPessimistic) throws Exception {
if (cacheConnectionOptions == null) {
cacheConnectionOptions = new CacheConnectionOptions();
}
cacheConnectionOptions.initialize(cacheName);
if (isClientCache) {
CacheConnectionOptions clientCacheConnectionOptions = new CacheConnectionOptions();
clientCacheConnectionOptions.setClientCacheMode(isPessimistic ? ClientCacheSyncMode.Pessimistic : ClientCacheSyncMode.Optimistic);
return getCachePrivate(cacheName, cacheConnectionOptions, _clientCacheID, clientCacheConnectionOptions);
}
CacheImpl cache = getCacheInternal(cacheName, cacheConnectionOptions);
cache.setMessagingServiceCacheImpl(cache.getCacheImpl());
cache.setDataTypeManagerImpl(cache);
return cache;
}
//endregion
//region Private Methods
private static boolean IsClientCache(String clientCacheName, tangible.RefObject isPessimistic) throws Exception {
if (clientCacheName == null) {
throw new IllegalArgumentException("cacheId");
}
String clientCacheID = ConfigReader.ClientCacheID("client.ncconf", clientCacheName, isPessimistic).trim();
if (clientCacheID.length() > 0) {
_clientCacheID = clientCacheID.toLowerCase();
return true;
} else {
return false;
}
}
private static CacheImpl getCachePrivate(String cacheName, CacheConnectionOptions cacheConnectionOptions, String clientCacheName, CacheConnectionOptions clientCacheConnectionOptions) throws Exception {
String userId = null, password = null;
if (cacheName.isEmpty())
throw new IllegalArgumentException("cacheName");
if ((cacheName.toLowerCase()).compareTo(clientCacheName.toLowerCase()) == 0) {
throw new OperationFailedException(ErrorCodes.CacheInit.SAME_NAME_FOR_BOTH_CACHES, ErrorMessages.getErrorMessage(ErrorCodes.CacheInit.SAME_NAME_FOR_BOTH_CACHES));
}
if (cacheConnectionOptions == null)
cacheConnectionOptions = new CacheConnectionOptions();
if (clientCacheConnectionOptions == null)
clientCacheConnectionOptions = new CacheConnectionOptions();
cacheConnectionOptions.setIsolationLevel(IsolationLevel.OutProc);
cacheConnectionOptions.initialize(cacheName);
CacheImpl l2Cache = getCacheInternal(cacheName, cacheConnectionOptions);
l2Cache.setMessagingServiceCacheImpl(l2Cache.getCacheImpl());
l2Cache.setDataTypeManagerImpl(l2Cache);
clientCacheConnectionOptions.initialize(clientCacheName);
if (clientCacheConnectionOptions.getUserCredentials() != null) {
if (clientCacheConnectionOptions.getUserCredentials().getUserID() != null && clientCacheConnectionOptions.getUserCredentials().getPassword() != null) {
userId = clientCacheConnectionOptions.getUserCredentials().getUserID();
password = clientCacheConnectionOptions.getUserCredentials().getPassword();
}
}
if (isNullOrEmpty(userId) && isNullOrEmpty(password)) {
if (cacheConnectionOptions.getUserCredentials() != null) {
if (cacheConnectionOptions.getUserCredentials().getUserID() != null && cacheConnectionOptions.getUserCredentials().getPassword() != null) {
userId = cacheConnectionOptions.getUserCredentials().getUserID();
password = cacheConnectionOptions.getUserCredentials().getPassword();
clientCacheConnectionOptions.setUserCredentials(new Credentials(userId, password));
}
}
}
boolean enableFaultTolerance = cacheConnectionOptions.isSkipUnAvailableClientCache() == true ? true : false;
CacheImpl l1Cache = initializeClientCache(clientCacheName, clientCacheConnectionOptions, l2Cache, !enableFaultTolerance);
boolean isPessim = false;
tangible.RefObject tempRef_isPessim = new tangible.RefObject(isPessim);
if (IsClientCache(cacheName, tempRef_isPessim) && !l1Cache.isInProc()) {
isPessim = tempRef_isPessim.argvalue;
if (_clientCacheID.equals(clientCacheName)) {
if (enableFaultTolerance) {
return new FailSafeClientCache(l2Cache, l1Cache, userId, password, isPessim, clientCacheConnectionOptions, clientCacheName, cacheConnectionOptions.getRetryL1ConnectionInterval());
} else
return new DisconnectedClientCache(l2Cache, l1Cache, userId, password, isPessim);
}
}
return new ClientCache(l2Cache, l1Cache, userId, password, clientCacheConnectionOptions.getClientCacheMode() == ClientCacheSyncMode.Pessimistic);
}
private static CacheImpl getCacheInternal(String cacheName, CacheConnectionOptions cacheConnectionOptions) throws Exception {
if (cacheName == null) {
throw new IllegalArgumentException("Value cannot be null or empty."+System.lineSeparator()+"Parameter name: cacheName");
}
if (cacheName.isEmpty()) {
throw new IllegalArgumentException("cacheId cannot be an empty string");
}
IsolationLevel mode = cacheConnectionOptions.getIsolationMode();
Credentials primaryUserCredentials = cacheConnectionOptions.getUserCredentials() == null ? new Credentials(null, null) : cacheConnectionOptions.getUserCredentials();
Credentials secondaryUserCredentials = cacheConnectionOptions.getSecondaryUserCredentials() == null ? new Credentials(null, null) : cacheConnectionOptions.getSecondaryUserCredentials();
String cacheIdWithAlias = cacheName + (!cacheConnectionOptions.getHaveBridgeCacheAlias() ? "" : "(" + cacheConnectionOptions.getBridgeCacheAlias() + ")");
int maxTries = 2;
Credentials securityParams = primaryUserCredentials;
try {
CacheServerConfig config = null;
if (mode != IsolationLevel.OutProc) {
do {
try {
config = DirectoryUtil.getCacheDom(cacheName, securityParams == null ? null : securityParams.getUserID(), securityParams == null ? null : securityParams.getPassword(), mode == IsolationLevel.InProc);
} catch (SecurityException se) {
maxTries--;
if (maxTries == 0) {
throw se;
}
securityParams = secondaryUserCredentials;
continue;
} catch (Exception ex) {
if (mode == IsolationLevel.Default) {
mode = IsolationLevel.OutProc;
} else {
throw ex;
}
}
if (config != null) {
if (config.getCacheType().toLowerCase().equals("clustered-cache")) {
throw new OperationFailedException(ErrorCodes.CacheInit.CLUSTER_INIT_IN_INPROC, ErrorMessages.getErrorMessage(ErrorCodes.CacheInit.CLUSTER_INIT_IN_INPROC));
}
switch (mode) {
case InProc:
config.setInProc(true);
break;
case OutProc:
config.setInProc(false);
break;
}
}
break;
} while (maxTries > 0);
}
synchronized (CacheManager.class) {
CacheImpl primaryCache = null;
synchronized (_cacheCollection) {
if (!_cacheCollection.contains(cacheIdWithAlias)) {
MetricsTransporterFactory transporterFactory = new MetricsTransporterFactoryImpl();
CacheImplBase cacheImpl = null;
PerfStatsCollector perfStatsCollector = null;
if (config != null && config.getInProc()) {
Alachisoft.NCache.Caching.Cache ncache = null;
CacheImpl cache = null;
maxTries = 2;
securityParams = primaryUserCredentials;
do {
try {
if (config.getCacheType().equals("partitioned-replicas-server") || config.getCacheType().equals("mirror-server")) {
String name = config.getCacheType().equals("mirror-server") ? "Mirror Topology" : "Partitioned Replica Topology";
throw new OperationFailedException(ErrorCodes.CacheInit.CANT_START_AS_INPROC, ErrorMessages.getErrorMessage(ErrorCodes.CacheInit.CANT_START_AS_INPROC, name));
}
if(config.getInProc()&&(!config.getCacheType().equalsIgnoreCase("client-cache")))
{
throw new OperationNotSupportedException(ErrorCodes.ClientCache.InprocCacheNotSuportedInJavaClient, ErrorMessages.getErrorMessage(ErrorCodes.ClientCache.InprocCacheNotSuportedInJavaClient, cacheName));
}
CacheConfig cacheConfig = CacheConfig.FromDom(config);
cache = new CacheImpl(null, cacheConfig);
if (securityParams != null) {
ncache = CacheFactory.CreateFromPropertyString(cacheConfig.getPropertyString(), config, securityParams.getUserID(), securityParams.getPassword(), false, false);
} else {
ncache = CacheFactory.CreateFromPropertyString(cacheConfig.getPropertyString(), config, null, null, false, false);
}
cacheImpl = new InprocCache(ncache, cacheConfig, cache, securityParams.getUserID(), securityParams.getPassword());
cache.setCacheImpl(cacheImpl);
if (primaryCache == null) {
primaryCache = cache;
primaryCache.setSecurityParameters(securityParams);
} else {
primaryCache.addSecondaryInprocInstance(cache);
}
break;
} catch (SecurityException se) {
maxTries--;
if (maxTries == 0) {
throw se;
}
securityParams = secondaryUserCredentials;
}
} while (maxTries > 0);
} else {
maxTries = 2;
securityParams = primaryUserCredentials;
do {
try {
perfStatsCollector = new PerfStatsCollector(cacheName, false);
primaryCache = new CacheImpl(null, cacheName, perfStatsCollector);
cacheImpl = new RemoteCache(cacheName, primaryCache, securityParams, cacheConnectionOptions, perfStatsCollector);
primaryCache.setSecurityParameters(securityParams);
primaryCache.setCacheImpl(cacheImpl);
perfStatsCollector.initializePerfCounters(false);
try {
if (!cacheConnectionOptions.getFromClientCache() && !cacheConnectionOptions.getIsBridgeClient()) {
if (!AppUtil.isMavenOnlyInstallation()) {
MonitoringConfigManager monitoringConfigManager = new MonitoringConfigManager();
com.alachisoft.ncache.client.internal.util.ClientConfiguration clientConfig = new com.alachisoft.ncache.client.internal.util.ClientConfiguration(null);
//dont load the cache related information from client.conf
clientConfig.setLoadCacheConfiguration(false);
clientConfig.loadLocalServerIP();
if (monitoringConfigManager.getCongigFile()) {
MetricsPublisher metricsPublisher = new MetricsPublisher(transporterFactory, cacheImpl);
metricsPublisher.setNCacheLog(cacheImpl.getNCacheLog());
metricsPublisher.setSessionId(cacheImpl.getMonitoringSessionId());
metricsPublisher.setCacheConfigID(cacheImpl.getCacheConfigId());
perfStatsCollector.setCategory(monitoringConfigManager.GetCategory(CategoriesConstants.NCacheClient));
perfStatsCollector.setStatsPublisher(metricsPublisher);
perfStatsCollector.RegisterOnMetricsPublisher();
metricsPublisher.Initialize();
}
perfStatsCollector.startPublishingCounters(clientConfig.getLocalServerIP());
}
}
}
catch (Exception ex)
{
}
break;
} catch (SecurityException se) {
maxTries--;
if (maxTries == 0) {
throw se;
}
securityParams = secondaryUserCredentials;
}
} while (maxTries > 0);
}
if (primaryCache != null) {
primaryCache.initializeCompactFramework();
_cacheCollection.addCache(cacheIdWithAlias, primaryCache);
primaryCache.InitializeEncryption();
}
} else {
synchronized (_cacheCollection.getCache(cacheIdWithAlias, false)) {
Object tempVar = _cacheCollection.getCache(cacheIdWithAlias, false);
primaryCache = (CacheImpl) ((tempVar instanceof CacheImpl) ? tempVar : null);
if (primaryUserCredentials != null && !isNullOrEmpty(primaryUserCredentials.getUserID()) && !isNullOrEmpty(primaryUserCredentials.getPassword())) {
try {
primaryCache.checkUserAuthorization(cacheName.toLowerCase(), primaryUserCredentials.getPassword(), primaryUserCredentials.getUserID());
} catch (Exception ex) {
if (ex instanceof SecurityException) {
try {
primaryCache.checkUserAuthorization(cacheName.toLowerCase(), secondaryUserCredentials.getPassword(), secondaryUserCredentials.getUserID());
} catch (java.lang.Exception e) {
throw e;
}
}
}
}
primaryCache.addRef();
}
}
}
synchronized (_cache) {
// it is first cache instance.
if (_cache.getCacheImpl() == null) {
primaryCache.setExceptionEnabled(getExceptionsEnabled());
_cache = primaryCache;
_cache.setDataTypeManagerImpl(_cache);
}
}
return primaryCache;
}
} catch (Exception e2) {
throw e2;
}
}
private static Credentials verifyCredentials(Credentials primaryCredentials, Credentials secondaryCredentials) {
Credentials credentials = primaryCredentials != null ? primaryCredentials : secondaryCredentials;
return credentials != null ? credentials : new Credentials("", "");
}
/**
* @hidden
* @param clientCacheName
* @param clientCacheConnectionOptions
* @param l2Cache
* @param throwInitializationError
* @return
* @throws Exception
*/
public static CacheImpl initializeClientCache(String clientCacheName, CacheConnectionOptions clientCacheConnectionOptions, CacheImpl l2Cache, boolean throwInitializationError) throws Exception {
try
{
CacheImpl cache = getCacheInternal(clientCacheName, clientCacheConnectionOptions);
cache.setMessagingServiceCacheImpl(l2Cache.getCacheImpl());
cache.setDataTypeManagerImpl(l2Cache);
return cache;
}
catch (RuntimeException | CacheException ex)
{
if (throwInitializationError || !ex.getMessage().contains("No server"))
{
throw ex;
}
else
{
return null;
}
}
}
//endregion
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy