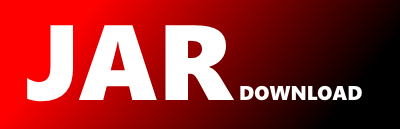
com.alachisoft.ncache.client.CacheReader Maven / Gradle / Ivy
package com.alachisoft.ncache.client;
import com.alachisoft.ncache.runtime.exceptions.CacheException;
import com.alachisoft.ncache.runtime.exceptions.OperationFailedException;
import java.io.IOException;
/**
* Reads one or more than forward-only stream of result sets by executing OQ commands on cache source.
*/
public interface CacheReader extends AutoCloseable
{
/**
* Gets number of columns.
*/
int getFieldCount();
/**
* True, if reader is closed else false.
*/
boolean getIsClosed();
/**
* Advances ICacheReader to next record.
* @return true if there are more rows; else false.
*/
boolean read() throws CacheException;
/**
* Gets value of specified index as bool.
* @param index Index of column.
* @return bool value on specified index.
*/
boolean getBoolean(int index) throws OperationFailedException;
/**
* Gets value of specified index as string.
* @param index Index of column.
* @return string value on specified column.
*/
String getString(int index) throws OperationFailedException;
/**
* Gets value of specified index as decimal.
* @param index Index of column.
* @return decimal value on specified index.
*/
java.math.BigDecimal getBigDecimal(int index) throws OperationFailedException;
/**
* Gets value of specified index as double.
* @param index Index of column.
* @return double value on specified index.
*/
double getDouble(int index) throws OperationFailedException;
/**
* Gets value of specified index as short.
* @param index Index of column.
* @return short value on specified index.
*/
short getShort(int index) throws OperationFailedException;
/**
* Gets value of specified index as integer.
* @param index Index of column.
* @return integer value on specified index.
*/
int getInt(int index) throws OperationFailedException;
/**
* Gets value of specified index as long.
* @param index Index of column.
* @return long value on specified index.
*/
long getLong(int index) throws OperationFailedException;
/**
* Gets value at specified column index.
* @param get generic type value at specified column index.
* @param index Index of column.
* @param cls Specifies the class of value obtained from the cache.
* @return Object value on specified index.
* @throws IOException
* @throws ClassNotFoundException
*/
T getValue(int index, Class> cls) throws IOException, ClassNotFoundException, OperationFailedException;
/**
* Gets value of specified column name.
* @param columnName Name of column.
* @param cls Specifies the class of value obtained from the cache.
* @return Object value of specified column.
*/
T getValue(String columnName, Class> cls) throws IOException, ClassNotFoundException, OperationFailedException;
/**
* Populates array of objects with values in current row.
* @param objects array of objects to be populated.
* @param cls Specifies the class of values obtained from the array.
* @return No of objects copied in specified array.
*/
int getValues(Object[] objects, Class> cls) throws IOException, ClassNotFoundException, OperationFailedException;
/**
* Returns name of specified column index.
* @param index Index of column.
* @return Name of column.
*/
String getName(int index);
/**
* Returns index of specified column name.
* @param columnName Name of column.
* @return Index of column.
*/
int getOrdinal(String columnName);
/**
* Returns Date at specified column index
* @param index Index of Column.
* @return Date at the specified index.
*/
java.util.Date getDate(int index) throws OperationFailedException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy