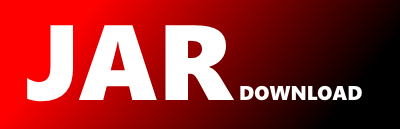
com.alachisoft.ncache.client.CacheStream Maven / Gradle / Ivy
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
package com.alachisoft.ncache.client;
import com.alachisoft.ncache.runtime.exceptions.NotSupportedException;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
/**
* CacheStream is derived from java.io.BufferedInputStream and java.io.BufferedOutputStream.It is designed to put/fetch BLOB using standard CacheStream interface.
*/
public interface CacheStream extends AutoCloseable{
/**
* Gets the length of the stream.
* @return The length of the stream.
* @throws Exception
*/
long length() throws Exception;
/**
* Gets a value indicating whether the current stream supports reading.
* @return True if stream supports reading otherwise false.
*/
boolean canRead();
/**
* Gets a value indicating whether the current stream supports seeking.
* @return True if stream supports seeking otherwise false.
*/
boolean canSeek();
/**
* Gets a value indicating whether the current stream supports writing.
* @return True if stream supports writing otherwise false.
*/
boolean canWrite();
/**
* Gets a value indicating whether stream is closed or not.
* @return True if stream is closed otherwise false.
*/
boolean closed();
/**
* When overridden in a derived class, clears all buffers for this stream and causes any
* buffered data to be written to the underlying device.
*/
void flush();
/**
* Gets the position within current stream.
* @return The position within the current stream.
* @throws Exception
*/
public long position() throws Exception;
/**
* Sets the position within the current stream.
* @param offset A byte offset relative to the origin parameter.
* @return The current position within the stream.
* @throws NotSupportedException If stream does not support seeking.
*/
public long seek(long offset) throws NotSupportedException;
/**
* Sets the length of the stream.
* @param value The desired length of the current stream in bytes.
* @throws NotSupportedException If stream does not support both writing and seeking.
*/
public void setLength(long value) throws NotSupportedException;
/**
* Gets the {@link BufferedInputStream} of given buffer size.
* @param bufferSize Buffer size in bytes.
* @return An instance of java.io.BufferedInputStream.
* @throws Exception
*/
public BufferedInputStream getBufferedInputStream(int bufferSize) throws Exception;
/**
* Gets the {@link BufferedInputStream} instance.
* @return An instance of java.io.BufferedInputStream.
* @throws Exception
*/
public BufferedInputStream getBufferedInputStream() throws Exception;
/**
* Gets the {@link BufferedOutputStream} of given buffer size.
* @param bufferSize Buffer size in bytes.
* @return An instance of java.io.BufferedOutputStream.
* @throws Exception
*/
public BufferedOutputStream getBufferedOutputStream(int bufferSize) throws Exception;
/**
* Gets the {@link BufferedOutputStream} of given buffer size.
* @return An instance of java.io.BufferedOutputStream.
* @throws Exception
*/
public BufferedOutputStream getBufferedOutputStream() throws Exception;
/**
* Reads a sequence of bytes from the current stream and advances the position within the stream by the number of bytes read.
* @param buffer An array of bytes. When this method returns, the buffer contains the specified
* byte array with the values between offset and (offset + count - 1) replaced
* by the bytes read from the current source.
* @param offset The zero-based byte offset in buffer at which to begin storing the data read
* from the current stream.
* @param count The maximum number of bytes to be read from the current stream.
* @return The total number of bytes read into the buffer. This can be less than the
* number of bytes requested if that many bytes are not currently available,
* or zero (0) if the end of the stream has been reached.
* @throws Exception
*/
public int read(byte[] buffer, int offset, int count) throws Exception;
/**
* Writes a sequence of bytes to the current stream and
* advances the current position within this stream by the number of bytes written.
* @param buffer An array of bytes. This method copies count bytes from buffer to the current stream.
* @param offset The zero-based byte offset in buffer at which to begin copying bytes to the current stream.
* @param count The number of bytes to be written to the current stream.
* @throws Exception
*/
public void write(byte[] buffer, int offset, int count) throws Exception;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy