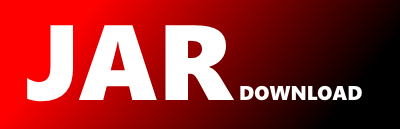
com.alachisoft.ncache.client.CacheStreamAttributes Maven / Gradle / Ivy
package com.alachisoft.ncache.client;
// Copyright (c) 2020 Alachisoft
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License
import com.alachisoft.ncache.runtime.CacheItemPriority;
import com.alachisoft.ncache.runtime.caching.expiration.Expiration;
import com.alachisoft.ncache.runtime.dependencies.CacheDependency;
/**
* CacheStreamAttributes Class contains information about the {@link CacheStream} attributes.
*/
public class CacheStreamAttributes {
private CacheItemPriority _priority = CacheItemPriority.Default;
private String _group = null;
private CacheDependency _dependency = null;
private Expiration _expiration = null;
private StreamMode _streamMode = StreamMode.Read;
/**
* Creates new instance of CacheStreamAttributes.
* @param streamMode Specifies {@link StreamMode} of the cache stream.
*/
public CacheStreamAttributes(StreamMode streamMode) {
setStreamMode(streamMode);
}
/**
* Gets the relative cache item priority of the cache stream.
* @return The relative cache item priority.
*/
public final CacheItemPriority getCacheItemPriority() {
return _priority;
}
/**
* Sets the relative cache item priority of the cache stream.
* @param value The relative cache item priority.
*/
public final void setCacheItemPriority(CacheItemPriority value) {
_priority = value;
}
/**
* Gets the group associated with cache stream.
* @return The group associated with the cache stream.
*/
public final String getGroup() {
return _group;
}
/**
* Sets the group associated with cache stream.
* @param value The group to be associated with the cache stream.
*/
public final void setGroup(String value) {
_group = value;
}
/**
* Gets the Cache Dependency instance that contains all dependencies associated with cache stream.
* @return The Cache Dependency instance associated with cache stream.
*/
public final CacheDependency getCacheDependency() {
return _dependency;
}
/**
* Sets the Cache Dependency instance that contains all dependencies associated with cache stream.
* @param value The Cache Dependency instance to be associated with cache stream.
*/
public final void setCacheDependency(CacheDependency value) {
_dependency = value;
}
/**
* Gets the expiration mechanism for cache stream.
* @return {@link Expiration} instance that contains info about cache stream expiration mechanism.
*/
public final Expiration getExpiration() {
return _expiration;
}
/**
* Sets the expiration mechanism for cache stream.
* @param value Expiration object that specifies the expiration mechanism for cache stream.
*/
public final void setExpiration(Expiration value) {
_expiration = value;
}
/**
* Gets the stream mode associated with the cache stream.
* @return The stream mode associated with the cache stream.
*/
public final StreamMode getStreamMode() {
return _streamMode;
}
/**
* Sets the stream mode associated with the cache stream.
* @param value The stream mode to be associated with the cache stream.
*/
public final void setStreamMode(StreamMode value) {
_streamMode = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy