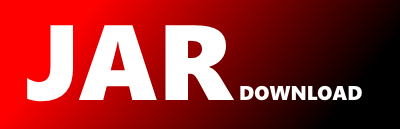
com.alachisoft.ncache.client.CacheSyncDependency Maven / Gradle / Ivy
/*
* CacheSyncDependency.java
*
* Created on December 14, 2006, 12:21 PM
*
* Copyright 2005 Alachisoft, Inc. All rights reserved.
* ALACHISOFT PROPRIETARY/CONFIDENTIAL. Use is subject to license terms.
*/
package com.alachisoft.ncache.client;
/**
* A client application can have more than one cache instances initialized.
* CacheSyncDependency keeps the items present in one cache synchronized with
* the items present in another cache.
*/
public class CacheSyncDependency {
private String _cacheId;
private String _key;
private String _server;
private String _userId;
private byte[] _password;
private int _port;
/**
* Initializes a new instance of the CacheSyncDependency with the
* specified parameters. Internally it tries to initialize the remote cache.
* If the cache can not be initialized, it throws the exception describing the cause of
* failure. The remote cache must be running as outproc even if it is on the same machine.
* The information to connect to the remote cache instance (like server-name and server-port)
* are picked from the 'client.conf'.
* @param remoteCacheId The unique id of the remote cache.
* @param key The key of the item in the remote cache with which the local cache item will be kept synchronized.
*/
public CacheSyncDependency(String remoteCacheId, String key) {
_cacheId = remoteCacheId;
_key = key;
}
/**
* Initializes a new instance of the CacheSyncDependency with the
* specified parameters. Internally it tries to initialize the remote cache.
* If the cache can not be initialized, it throws the exception describing the cause of
* failure. The remote cache must be running as outproc even if it is on the same machine.
* @param remoteCacheId The unique id of the remote cache.
* @param key The key of the item in the remote cache with which the local cache item will be kept synchronized.
* @param server The name of the server where the remote cache is running.
* @param port The port used by the client to connect to the server.
*/
public CacheSyncDependency(String remoteCacheId, String key, String server, int port) {
this(remoteCacheId, key);
_server = server;
_port = port;
}
/**
* Initializes a new instance of the CacheSyncDependency with the
* specified parameters. Internally it tries to initialize the remote cache.
* If the cache can not be initialized, it throws the exception describing the cause of
* failure. The remote cache must be running as outproc even if it is on the same machine.
* @param remoteCacheId The unique id of the remote cache.
* @param key The key of the item in the remote cache with which the local cache item will be kept synchronized.
* @param userId The user id of the remote cache.
* @param password The Security password of the remote cache.
*/
public CacheSyncDependency(String remoteCacheId, String key, String userId, byte[] password) {
this(remoteCacheId, key);
_userId = userId;
_password = password;
}
/**
* The unique Id of the remote cache
* @return The string cacheid.
*/
public String getCacheId() {
return _cacheId;
}
/**
* The key of the item in the remote cache with which the local cache item needs to be synchronized.
* @return The string key of the item in remote cache.
*/
public String getKey() {
return _key;
}
/**
* The name of the server where the remote cache is running.
* @return The name of the server. or the IP.
*/
public String getServer() {
return _server;
}
/**
* Specifies the User Id for user authorization.
* @return The user id.
*/
public String getUserId() {
return _userId;
}
/**
* Specifies the user authorization password.
* @return The password required for user authorization.
*/
public byte[] getPassword() {
return _password;
}
/**
* The server port that is used by the clients to connect to the server.
* @return The integer value of the port.
*/
public int getPort() {
return _port;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy