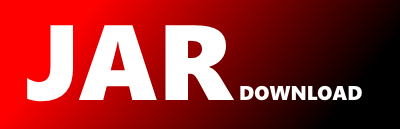
com.alachisoft.ncache.client.EventCacheItem Maven / Gradle / Ivy
package com.alachisoft.ncache.client;
import Alachisoft.NCache.Common.BitSet;
import com.alachisoft.ncache.common.caching.EntryType;
import com.alachisoft.ncache.runtime.CacheItemPriority;
import com.alachisoft.ncache.runtime.JSON.JsonUtil;
import com.alachisoft.ncache.runtime.caching.datasource.ResyncOptions;
/**
* This is a stripped down version of {@link CacheItem}.
* Contains basic information of an item present in the cache
*/
public class EventCacheItem implements Cloneable {
/**
* The actual object provided by the client application
*/
private Object _value;
private CacheItemPriority _cacheItemPriority;
//Callbacks
private boolean _resyncExpiredItems;
private String _group;
//private Hashtable _queryInfo;
private CacheItemVersion _version;
private ResyncOptions _resyncOptions;
private BitSet _flagMap;
private EntryType _entryType;
//private Tag[] _tags;
//Expensive to evaluate and convert to client understanding
//private Expiration
//Expensive to extract
//private NamedTagsDictionary
//Expensive to extract
//private Dependencies
EventCacheItem() {
}
/**
* Will contain the value present in the cache but only if the event was registered against
* EventDataFilter.Metadata or EventDataFilter.DataWithMetadata otherwise it will be null.
* @param cls Specifies the class of value obtained from the EventCacheItem.
* @param Specifies the type of value obtained from the EventCacheItem.
* @return The value stored in EventCacheItem.
*/
public final T getValue(Class> cls) {
if(cls==null)
cls=Object.class;
return JsonUtil.getValueAs(_value,cls);
}
/**
* @hidden
* @param value
*/
final void setValue(Object value) {
_value = value;
}
/**
* Specifies the CacheItemPriority of the item present in the cache
* @return CacheItemPriority of the EventCacheItem.
*/
public final CacheItemPriority getCacheItemPriority() {
return _cacheItemPriority;
}
/**
* @hidden
* @param value
*/
public final void setCacheItemPriority(CacheItemPriority value) {
_cacheItemPriority = value;
}
/**
* ResyncOptions contain information if items are to be resynced at expiry and
* readthrough provider name when item will be resynced at expiry.
* @return The ResyncOptions specific to the EventCacheItem.
*/
public ResyncOptions getResyncOptions() {
return _resyncOptions;
}
/**
* @hidden
* @param value
*/
public void setResyncOptions(ResyncOptions value) {
_resyncOptions = value;
}
/**
* Specifies whether item is to be resynced on expiration or not.
* @return True if item is to be resynced otherwise false.
*/
public final boolean getResyncExpiredItems() {
return _resyncExpiredItems;
}
/**
* @hidden
* @param value
*/
public final void setReSyncExpiredItems(boolean value) {
_resyncExpiredItems = value;
}
/**
* Gets the group associated with the EventCacheItem.
* @return The group associated with the EventCacheItem.
*/
public final String getGroup() {
return _group;
}
/**
* @hidden
* @param value
*/
public final void setGroup(String value) {
_group = value;
}
/**
* Item version of the item
*/
public final CacheItemVersion getCacheItemVersion() {
return _version;
}
/**
* @hidden
* @param value
*/
public final void setCacheItemVersion(CacheItemVersion value) {
_version = value;
}
BitSet getFlagmap() {
return _flagMap;
}
void setFlagmap(BitSet value) {
_flagMap = value;
}
/**
* Gets the entry type associated with the EventCacheItem.
* @return The entry type associated with the EventCacheItem.
*/
public EntryType getEntryType() {
return _entryType;
}
//public Tag[] Tags
//{
// get { return _tags; }
// internal set { _tags = value; }
//}
/**
* @hidden
* @param value
*/
public void setEntryType(EntryType value) {
_entryType = value;
}
/**
* Creates and returns a copy of this object. The precise meaning
* of "copy" may depend on the class of the object. The general
* intent is that, for any object {@code x}, the expression:
*
*
* x.clone() != x
* will be true, and that the expression:
*
*
* x.clone().getClass() == x.getClass()
* will be {@code true}, but these are not absolute requirements.
* While it is typically the case that:
*
*
* x.clone().equals(x)
* will be {@code true}, this is not an absolute requirement.
*
* By convention, the returned object should be obtained by calling
* {@code super.clone}. If a class and all of its superclasses (except
* {@code Object}) obey this convention, it will be the case that
* {@code x.clone().getClass() == x.getClass()}.
*
* By convention, the object returned by this method should be independent
* of this object (which is being cloned). To achieve this independence,
* it may be necessary to modify one or more fields of the object returned
* by {@code super.clone} before returning it. Typically, this means
* copying any mutable objects that comprise the internal "deep structure"
* of the object being cloned and replacing the references to these
* objects with references to the copies. If a class contains only
* primitive fields or references to immutable objects, then it is usually
* the case that no fields in the object returned by {@code super.clone}
* need to be modified.
*
* The method {@code clone} for class {@code Object} performs a
* specific cloning operation. First, if the class of this object does
* not implement the interface {@code Cloneable}, then a
* {@code CloneNotSupportedException} is thrown. Note that all arrays
* are considered to implement the interface {@code Cloneable} and that
* the return type of the {@code clone} method of an array type {@code T[]}
* is {@code T[]} where T is any reference or primitive type.
* Otherwise, this method creates a new instance of the class of this
* object and initializes all its fields with exactly the contents of
* the corresponding fields of this object, as if by assignment; the
* contents of the fields are not themselves cloned. Thus, this method
* performs a "shallow copy" of this object, not a "deep copy" operation.
*
* The class {@code Object} does not itself implement the interface
* {@code Cloneable}, so calling the {@code clone} method on an object
* whose class is {@code Object} will result in throwing an
* exception at run time.
*
* @return a clone of this instance
* @see java.lang.Cloneable
*/
public final Object clone() {
EventCacheItem clone = new EventCacheItem();
clone._group = _group;
clone._version = _version;
clone._resyncOptions = _resyncOptions;
clone._cacheItemPriority = _cacheItemPriority;
clone._value = _value;
clone._entryType = _entryType;
clone._flagMap = _flagMap;
return clone;
}
}