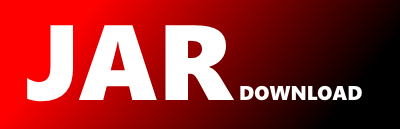
com.alachisoft.ncache.client.NCacheErrorCodes Maven / Gradle / Ivy
package com.alachisoft.ncache.client;
import Alachisoft.NCache.Common.ErrorHandling.ErrorCodes;
/**
* @hidden
*/
public class NCacheErrorCodes
{
//init
public static final int SERVER_INFO_NOT_FOUND = ErrorCodes.CacheInit.SERVER_INFO_NOT_FOUND; //"'client.ncconf' not found or does not contain server information"
public static final int CACHE_ID_EMPTY_STRING = ErrorCodes.CacheInit.CACHE_ID_EMPTY_STRING; //cacheId cannot be an empty string
public static final int CACHE_NOT_REGISTERED_ON_NODE = ErrorCodes.CacheInit.CACHE_NOT_REGISTERED_ON_NODE; //"cache with name '{0}' not registered on specified node"
public static final int CACHE_ID_NOT_REGISTERED = ErrorCodes.CacheInit.CACHE_ID_NOT_REGISTERED; //"Specified cacheId is not registered."
public static final int CACHE_ALREADY_RUNNING = ErrorCodes.CacheInit.CACHE_ALREADY_RUNNING; //"Specified cacheId is already running"
public static final int CACHE_NOT_INIT = ErrorCodes.CacheInit.CACHE_NOT_INIT; //"Cache is not initialized"
public static final int L1_CACHE_NOT_INIT = ErrorCodes.CacheInit.L1_CACHE_NOT_INIT; //"level1Cache not initialized"
public static final int L2_CACHE_NOT_INIT = ErrorCodes.CacheInit.L2_CACHE_NOT_INIT; //"level2Cache not initialized"
public static final int CACHE_KEY_NULL = ErrorCodes.CacheInit.CACHE_KEY_NULL; //"cache key null"
public static final int CACHE_ID_NULL = ErrorCodes.CacheInit.CACHE_ID_NULL; //"Cache id can not be null"
public static final int SAME_NAME_FOR_BOTH_CACHES = ErrorCodes.CacheInit.SAME_NAME_FOR_BOTH_CACHES; //"Same cache-name cannot be specified for both Primary and Client caches."
public static final int CLUSTER_INIT_IN_INPROC = ErrorCodes.CacheInit.CLUSTER_INIT_IN_INPROC; //"Cluster cache cannot be initialized in In-Proc mode."
public static final int CANT_START_AS_INPROC = ErrorCodes.CacheInit.CANT_START_AS_INPROC; //"Cannot start cache of type '{0}' as in-proc cache."
//common
public static final int NOT_ENOUGH_ITEMS_EVICTED = ErrorCodes.Common.NOT_ENOUGH_ITEMS_EVICTED; //"The cache is full and not enough items could be evicted."
public static final int DEPENDENCY_KEY_NOT_FOUND = ErrorCodes.Common.DEPENDENCY_KEY_NOT_FOUND; //"One of the dependency keys does not exist."
public static final int REQUEST_TIMEOUT = ErrorCodes.Common.REQUEST_TIMEOUT; //"Request timeout due to node down"
public static final int OPERATION_INTERRUPTED = ErrorCodes.Common.OPERATION_INTERRUPTED; //"Operation has been interrupted due to loss of connectivity between server and client. "
public static final int ENUMERATION_MODIFIED = ErrorCodes.Common.ENUMERATION_MODIFIED; //"Enumeration has been modified"
public static final int NO_SERVER_AVAILABLE = ErrorCodes.Common.NO_SERVER_AVAILABLE_FOR_CACHE;
//pubsub
public static final int SUBSCRIPTION_EXISTS = ErrorCodes.PubSub.SUBSCRIPTION_EXISTS; //Active subscription with this name already exists
public static final int TOPIC_NOT_FOUND = ErrorCodes.PubSub.TOPIC_NOT_FOUND; //"Topic '{0}' does not exists."
public static final int MESSAGE_ID_ALREADY_EXISTS = ErrorCodes.PubSub.MESSAGE_ID_ALREADY_EXISTS; //"The specified message id already exists."
public static final int TOPIC_DISPOSED = ErrorCodes.PubSub.TOPIC_DISPOSED; //"Topic '{0}' is disposed."
public static final int DEFAULT_TOPICS = ErrorCodes.PubSub.DEFAULT_TOPICS; //"Operation cannot be performed on default topics."
public static final int PATTERN_BASED_PUBLISHING_NOT_ALLOWED = ErrorCodes.PubSub.PATTERN_BASED_PUBLISHING_NOT_ALLOWED; //"Message publishing on pattern based topic is not allowed"
//mapreduce
public static final int TASK_STARTING_FAILED = ErrorCodes.MapReduce.TASK_STARTING_FAILED; //"Task failed while starting."
public static final int ENUMERATION_ERROR = ErrorCodes.MapReduce.ENUMERATION_ERROR; //"Enumeration has either not started or already finished."
public static final int CALLBACK_ALREADY_REGISTERED = ErrorCodes.MapReduce.CALLBACK_REGISTERED; //"You have already registered a callback (async method for Get Result)"
public static final int TASK_FAILED = ErrorCodes.MapReduce.TASK_FAILED; //"Task was failed. Reason: '{0}'. Please refer to logs for detailed information."
public static final int TASK_CANCELLED_REASON = ErrorCodes.MapReduce.TASK_CANCELLED_REASON; //"Task was canceled. Reason: '{0}'"
public static final int GET_REQUEST_TIMEOUT = ErrorCodes.MapReduce.GET_TIMEOUT; //"GetResult request timeout."
public static final int TASK_SUBMISSION_FAILED = ErrorCodes.MapReduce.TASK_SUBMISSION_FAILED; //"Task Submission Failed"
public static final int TASK_IN_SUBMISSION_FAILED = ErrorCodes.MapReduce.TASK_IN_SUBMISSION_FAILED_NODE; //"Task failed during submission on Node : '{0}'"
public static final int TASK_FAILED_WHILE_STARTING = ErrorCodes.MapReduce.TASK_FAILED_WHILE_STARTING; //"Task failed while starting on Node : '{0}'"
public static final int TASK_ENUMERATOR_INVALID = ErrorCodes.MapReduce.TASK_ENUMERATOR_INVALID; //"Task Enumerator is Invalid"
public static final int TASK_CANCELLED = ErrorCodes.MapReduce.TASK_CANCELLED; //"Task with Specified TaskId was cancelled."
public static final int TASK_CANCELLED_1 = ErrorCodes.MapReduce.TASK_CANCELLED_1; //"Task with specified Task ID was cancelled"
public static final int TASK_CANCELLED_2 = ErrorCodes.MapReduce.TASK_CANCELLED_2; //"Task with specified Task ID was Cancelled."
public static final int TASK_WITH_KEY_NOT_FOUND = ErrorCodes.MapReduce.TASK_WITH_KEY_DONT_EXIST; //"Task with specified key does not exist."
public static final int TASK_CANT_BE_SUBMITTED = ErrorCodes.MapReduce.TASK_CANT_BE_SUBMITTED; //"No more task can be submitted"
public static final int NULL_OR_EMPTY_TASK = ErrorCodes.MapReduce.NULL_OR_EMPTY_TASK; //"Task can not be null or empty"
//expiration
public static final int ERROR_LOADING_ORACLE_DATA_ACCESS = ErrorCodes.Expiration.ERROR_LOADING_ORACLE_DATA_ACCESS; //"Could not load assembly 'Oracle.DataAccess.dll'. Please make sure Oracle Data Provider for .NET is installed"
public static final int ABSOLUTE_DEFAULT_EXPIRATION = ErrorCodes.Expiration.ABSOLUTE_DEFAULT_EXPIRATION; //"Absolute Default expiration value is less than 5 seconds."
public static final int ABSOLUTE_LONGER_EXPIRATION = ErrorCodes.Expiration.ABSOLUTE_LONGER_EXPIRATION; //"Absolute Longer expiration value is less than 5 seconds."
public static final int SLIDING_DEFAULT_EXPIRATION = ErrorCodes.Expiration.SLIDING_DEFAULT_EXPIRATION; //"Sliding Default expiration value is less than 5 seconds."
public static final int SLIDING_LONGER_EXPIRATION = ErrorCodes.Expiration.SLIDING_LONGER_EXPIRATION; // "Sliding Longer expiration value is less than 5 seconds."
//basic operations
public static final int KEY_ALREADY_EXISTS = ErrorCodes.BasicCacheOperations.KEY_ALREADY_EXISTS; //"The specified key already exists."
public static final int BACKING_SOURCE_NOT_AVAILABLE = ErrorCodes.BackingSource.BACKING_SOURCE_NOT_AVAILABLE; //"Backing source not available. Verify backing source settings"
public static final int INSERTED_ITEM_DATAGROUP_MISMATCH = ErrorCodes.BasicCacheOperations.INSERTED_ITEM_DATAGROUP_MISMATCH; //"Data group of the inserted item does not match the existing item's data group."
public static final int ITEM_LOCKED = ErrorCodes.BasicCacheOperations.ITEM_LOCKED; //"Item is locked."
public static final int ITEM_WITH_VERSION_DOESNT_EXIST = ErrorCodes.BasicCacheOperations.ITEM_WITH_VERSION_DOESNT_EXIST; //"An item with specified version doesn't exist."
//streaming
public static final int GROUP_SUBGROUP_STREAM_MISMATCH = ErrorCodes.Streaming.GROUP_SUBGROUP_STREAM_MISMATCH; //"Data group/subgroup of the stream does not match the existing stream's data group/subgroup"
public static final int GROUP_STREAM_MISMATCH = ErrorCodes.Streaming.GROUP_STREAM_MISMATCH; //"Data group of the stream does not match the existing stream's data group"
//datatypes
public static final int IMPROPER_DATATYPE_SPECIFIED = ErrorCodes.DataTypes.DATATYPE_SPECIFIED; //"DataType must be properly specified"
public static final int NO_ITEM_IN_HASHSET = ErrorCodes.DataTypes.NO_ITEM_IN_HASHSET; //"No item present in HashSet"
public static final int NOT_A_LIST = ErrorCodes.DataTypes.NOT_A_LIST; //"Type mismatch: Corresponding item in cache is not a List."
public static final int NOT_A_QUEUE = ErrorCodes.DataTypes.NOT_A_QUEUE; //"Type mismatch: Corresponding item in cache is not a Queue."
public static final int NOT_A_SET = ErrorCodes.DataTypes.NOT_A_SET; //"Type mismatch: Corresponding item in cache is not a Set."
public static final int NOT_A_HASHSET = ErrorCodes.DataTypes.NOT_A_HASHSET; // "Type mismatch: Corresponding item in cache is not a HashSet."
public static final int NOT_A_COUNTER = ErrorCodes.DataTypes.NOT_A_COUNTER; //"Type mismatch: Corresponding item in cache is not a Counter."
public static final int TYPE_NOT_ALLOWED = ErrorCodes.DataTypes.TYPE_NOT_ALLOWED; //"Type is not allowed"
public static final int COUNT_NON_POSITIVE = ErrorCodes.DataTypes.COUNT_NON_POSITIVE; //"Count is non positive integer"
public static final int CACHEITEM_IN_DATA_STRUCTURES = ErrorCodes.DataTypes.CACHEITEM_IN_DATA_STRUCTURES; // "CacheItem cannot be used in Data structures"
public static final int INVALID_COLLECTION_RESPONSE = ErrorCodes.DataTypes.INVALID_COLLECTION_RESP; //"Invalid collection response type!"
public static final int ARRAY_FAILED_TO_HOLD_DS_ITEMS = ErrorCodes.DataTypes.ARRAY_FAILED_TO_HOLD_DS_ITEMS; //"Target array starting from the index specified is not big enough to hold data structure's items."
public static final int NOT_A_DICTIONARY = ErrorCodes.DataTypes.NOT_A_DICTIONARY; //Type mismatch: Corresponding item in cache is not a Dictionary.
//queries
public static final int SAME_VALUE_SELECTED_TWICE = ErrorCodes.Queries.SAME_VALUE_SELECTED_TWICE; //"Invalid query. Same value cannot be selected twice."
public static final int ATTRIBUTE_MISSING_IN_GROUP_BY = ErrorCodes.Queries.ATTRIBUTE_MISSING_IN_GROUP_BY; // "Invalid query. '{0}' must be specified in GROUP BY clause."
public static final int VALUES_NOT_SPECIFIED = ErrorCodes.Queries.VALUES_NOT_SPECIFIED; //"Value(s) not specified for indexed attribute '{0}'."
public static final int EXTRA_VALUES_SPECIFIED = ErrorCodes.Queries.EXTRA_VALUES_SPECIFIED; //"Extra value(s) are specified for indexed attribute '{0}'."
public static final int LESS_VALUES_SPECIFIED = ErrorCodes.Queries.LESS_VALUES_SPECIFIED; // "Less value(s) are specified for indexed attribute '{0}'."
public static final int INDEX_NOT_DEFINED = ErrorCodes.Queries.INDEX_NOT_DEFINED; //"Index is not defined for attribute '{0}'"
public static final int MAX_APPLIED_ON_BOOL = ErrorCodes.Queries.MAX_APPLIED_ON_BOOL; //"MAX cannot be applied to Boolean data type."
public static final int MIN_APPLIED_ON_BOOL = ErrorCodes.Queries.MIN_APPLIED_ON_BOOL; //"MIN cannot be applied to Boolean data type."
public static final int CURRENT_STATE_NOT_SUPPORTED = ErrorCodes.Queries.CURRENT_STATE_NOT_SUPPORTED; //"Operation is not valid due to the current state of the object"
public static final int UNABLE_EXTRACTING_QUERY_INFO = ErrorCodes.Queries.UNABLE_EXTRACTING_QUERY_INFO; //"Unable extracting query information from user object."
public static final int AVERAGE_FOR_INTEGRAL_DATATYPES_ONLY = ErrorCodes.Queries.AVERAGE_FOR_INTEGRAL_DATATYPES_ONLY; //"AVG can only be applied to integral data types."
public static final int DUPLICATE_COLUMNS = ErrorCodes.Queries.DUPLICATE_COLUMNS; // "Invalid query. Duplicate columns found in orderby clause."
public static final int SUM_FOR_INTEGRAL_DATATYPES = ErrorCodes.Queries.SUM_FOR_INTEGRAL_DATATYPES; //"SUM can only be applied to integral data types."
public static final int SYNTAX_NOT_SUPPORTED = ErrorCodes.Queries.SYNTAX_NOT_SUPPORTED; //"This syntax is currently not supported."
public static final int INCORRECT_FORMAT_SPECIFIED = ErrorCodes.Queries.INCORRECT_FORMAT_SPEC; //"Incorrect query format. '{0}' not supported."
public static final int INCORRECT_FORMAT_GIVEN = ErrorCodes.Queries.INCORRECT_FORMAT_GIVEN; //"Incorrect query format \'*\'."
public static final int PROJECTION_TYPE_NOT_SUPPORTED = ErrorCodes.Queries.PROJECTION_TYPE_NOT_SUPPORTED; //"The {0} projection type is not supported in the ProjectionPredicate."
public static final int SPECIFIED_FEILD_PROPERTY_NOT_FOUND = ErrorCodes.Queries.SPECIFIED_FEILD_PROPERTY_NOT_FOUND; //"Provided data contains no field or property named {0}"
public static final int ORDER_BY_ATTRIBUTES_MISSING = ErrorCodes.Queries.ORDER_BY_ATTRIBUTES_MISSING; //"Invalid query. All ORDER BY attributes must be included in GROUP BY clause."
public static final int INCORRECT_FORMAT = ErrorCodes.Queries.INCORRECT_FORMAT; //"Incorrect query format"
public static final int GROUP_BY_SECTION_NOT_SUPPORTED = ErrorCodes.Queries.GROUP_BY_SECTION_NOT_SUPPORTED; //"Search query doesn't support Group By Section"
public static final int ONLY_SELECT_SUPPORTED = ErrorCodes.Queries.ONLY_SELECT_SUPPORTED; //"Only select query is supported."
public static final int TEXT_BASED_SEARCHING_NOT_SUPPORTED = ErrorCodes.Queries.TEXT_BASED_SEARCHING_NOT_SUPPORTED; //"Text based searching is not supported in Continous Query"
public static final int ORDER_BY_SECTION_NOT_SUPPORTED = ErrorCodes.Queries.ORDER_BY_SECTION_NOT_SUPPORTED; //"Search query doesn't support Order By Section"
public static final int INDEX_NOT_DEFINED_ON_ATTRIBUE = ErrorCodes.Queries.INDEX_NOT_DEFINED_ON_ATTRIBUE; //"The queried attribute does not have an index defined on it."
//bridge
public static final int SHUTDOWN_STARTED = ErrorCodes.Bridge.SHUTDOWN_STARTED; //"Shutdown is started"
public static final int NO_SERVER_AVAILABLE_FOR_CACHE = ErrorCodes.Bridge.NO_SERVER_AVAILABLE; //"No server is available to process the request"
public static final int OPERATION_TIMED_OUT = ErrorCodes.Bridge.OPERATION_TIMED_OUT; //"Operation timed out. No response from the server ['{0}'] for '{1}'"
public static final int RESOLVE_FAILED = ErrorCodes.Bridge.RESOLVE_FAILED; //"IBridgeConflictResolver.Resolve failed '{0}'"
public static final int BRIDGE_CONFLICT_RESOLUTION_MGR = ErrorCodes.Bridge.BRIDGE_CONFLICT_RESOLUTION_MGR; //"Unable to instantiate BridgeConflictResolutionMgr."
public static final int ACTIVE_PASSIVE_OPERATION_FAILED = ErrorCodes.Bridge.ACTIVE_PASSIVE_OPERATION_FAILED; // "MakeCacheActivePassive operation failed. Error: {0}"
//backing source
public static final int SYNCHRONIZATION_WITH_DATASOURCE_FAILED = ErrorCodes.BackingSource.SYNCHRONIZATION_WITH_DATASOURCE; //"Error occurred while synchronization with data source: '{0}'"
public static final int INVALID_IWRITETHRU_PROVIDER_NAME = ErrorCodes.BackingSource.INVALID_IWRITE_THRU_PROVIDER;
public static final int INVALID_IREADTHRU_PROVIDER_NAME = ErrorCodes.BackingSource.INVALID_IREAD_THRU_PROVIDER;
//notifications
public static final int COLLECTION_TYPE_MISMATCH = ErrorCodes.Notifications.COLLECTION_TYPE_MISMATCH; // "Collection type mismatch. Notifications cannot be registered via the handle at hand."
public static final int COLLECTION_EVENTS_ON_NONCOLLECTION = ErrorCodes.Notifications.COLLECTION_EVENTS_ON_NONCOLLECTION; //"Cannot register collection events on non-collection item."
//json
public static final int ATTRIBUTE_ALREADY_EXISTS = com.alachisoft.ncache.runtime.errorhandling.ErrorCodes.Json.ATTRIBUTE_ALREADY_EXISTS; // "An attribute with the same name aleady exists."
public static final int REFERENCE_TO_PARENT = com.alachisoft.ncache.runtime.errorhandling.ErrorCodes.Json.REFERENCE_TO_PARENT; //"Reference to parent at nested level detected."
public static final int REFERENCE_TO_SELF = com.alachisoft.ncache.runtime.errorhandling.ErrorCodes.Json.REFERENCE_TO_SELF; //"'{0}' cannot contain an attribute that is a reference to self."
//search operations
public static final int KEY_CONFLICTS_WITH_INDEXED_ATTRIBUTE = ErrorCodes.SearchOperations.KEY_CONFLICTS_WITH_INDEXED_ATTRIBUTE; // "Key in named tags conflicts with the indexed attribute name of the specified object."
public static final int GROUP_TAGS_ON_SAME_ITEM = ErrorCodes.SearchOperations.GROUP_TAGS_ON_SAME_ITEM; //"You cannot set both groups and tags on the same cache item."
//EntryProcessor
public static final int NOT_SUPPORTED_FOR_COLLECTIONS = ErrorCodes.EntryProcessor.NOT_SUPPORTED_FOR_COLLECTIONS; //EntryProcessor is not supported for Collections.
// SQL dependency
public static final int SQL_DEPENDENCY_SYNTAX_ERROR = ErrorCodes.SQLDependency.INCORRECT_SYNTAX;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy