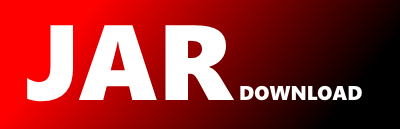
com.alachisoft.ncache.client.ServerInfo Maven / Gradle / Ivy
package com.alachisoft.ncache.client;
// Copyright (c) 2020 Alachisoft
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License
import Alachisoft.NCache.Common.Net.DnsCache;
import java.net.Inet4Address;
import java.net.InetAddress;
import java.net.UnknownHostException;
/**
* Provide connection information for the client to the server node in cache.
*/
public class ServerInfo implements java.lang.Comparable {
private int _port = 9800;
private String _name= null;
private InetAddress _ipAddress;
private short _portRange = 1;
private short _priority = 0;
private boolean _userProvided;
/**
* Initializes a new instance of ServerInfo.
* @param name Specifies name of the server node where cache is running.
*/
public ServerInfo(String name) {
this(name, 9800);
}
/**
* Initializes a new instance of ServerInfo.
* @param name Specifies name of the server node where cache is running.
* @param port Specifies port for client to connect to the server node.
*/
public ServerInfo(String name, int port) {
setName(name);
_port = port;
}
/**
* Initializes new instance of ServerInfo.
* @param ip Specifies {@link InetAddress} of the server node where cache is running.
*/
public ServerInfo(InetAddress ip) {
this(ip, 9800);
}
/**
* Initializes new instance of ServerInfo.
* @param ip Specifies {@link InetAddress} of the server node where cache is running.
* @param port Specifies port for client to connect to the server node.
*/
public ServerInfo(InetAddress ip, int port) {
setIP(ip);
_port = port;
}
public ServerInfo() {
}
/**
* Gets the port for client to connect to the server node.
* @return The port for client to connect to the server node.
*/
public final int getPort() {
return _port;
}
/**
* Sets the port for client to connect to the server node.
* @param value The port for client to connect to the server node.
*/
public final void setPort(int value) {
_port = value;
}
/**
* Gets the name of the server node where cache is running.
* @return The name of the server node where cache is running.
*/
public final String getName() {
if (_name != null) return _name;
if (_ipAddress != null) return _ipAddress.getHostAddress();
return "";
}
/**
* Gets the name of the server node where cache is running.
* @param value The name of the server node where cache is running.
*/
public final void setName(String value) {
if (value.isEmpty()) return;
if (value != null && value.toLowerCase().equals("localhost")) {
try {
_name = InetAddress.getLocalHost().getHostName();
} catch (UnknownHostException e) {
}
} else {
_name = value;
}
}
/**
* Gets the IPAddress of the server node where cache is running.
* @return The IPAddress of the server node where cache is running.
*/
public final InetAddress getIP() {
if (_ipAddress == null) {
try {
if (_name != null) {
try {
_ipAddress = InetAddress.getByName(_name);
return _ipAddress;
} catch (UnknownHostException e) {
_ipAddress = DnsCache.ResolveName(_name);
}
_ipAddress = DnsCache.ResolveName(_name);
}
} catch (UnknownHostException e2) {
}
}
return _ipAddress;
}
/**
* Sets the IPAddress of the server node where cache is running.
* @param value The IPAddress of the server node where cache is running.
*/
public final void setIP(InetAddress value) {
_ipAddress = value;
}
/**
* Sets the IPAddress of the server node where cache is running.
* @param value The IPAddress of the server node where cache is running in form of a string.
*/
public final void setIP(String value) {
try {
_ipAddress= InetAddress.getByName(value);
} catch (UnknownHostException e) {
e.printStackTrace();
}
}
/**
* @hidden
* @return
*/
public final short getPortRangeInternal() {
return _portRange;
}
/**
* @hidden
* @param value
*/
public final void setPortRangeInternal(short value) {
if (_portRange > 0) {
_portRange = value;
}
}
/**
* @hidden
* @return
*/
private final short getPriorityInternal() {
return _priority;
}
/**
* @hidden
* @param value
*/
public final void setPriorityInternal(short value) {
if (value > 0) {
_priority = value;
}
}
/**
* @hidden
* @return
*/
public final boolean getIsUserProvidedInternal() {
return _userProvided;
}
/**
* @hidden
* @param value
*/
public final void setIsUserProvidedInternal(boolean value) {
_userProvided = value;
}
/**
* @hidden
* @return
*/
public final String getIpStringInternal() {
if (_ipAddress == null) {
return "";
} else {
return _ipAddress.toString();
}
}
/**
* Compares two ServerInfo instances.
* @param obj The server info object to compare.
* @return True if specified object is equal to this object otherwise false.
*/
@Override
public boolean equals(Object obj) {
if (obj == null) {
return false;
}
return compareReal(obj) == 0;
}
/**
* Converts the value of this instance to its equivalent string representation.
* @return The string representation of ServerInfo.
*/
@Override
public String toString() {
String ip="";
if(_ipAddress !=null)
{
ip= _ipAddress.toString();
}else{
ip= _name;
}
return ip + ":" + _port;
}
///#region IComparable Members
/**
* @hidden
* @param o
* @return
*/
public final int compareReal(Object o) {
int h1 = 0, h2 = 0, rc;
if ((o == null)) {
return 1;
}
ServerInfo other = o instanceof ServerInfo ? (ServerInfo) o : null;
if (other == null) {
return 1;
}
if (_ipAddress != null) {
h1 = _ipAddress.hashCode();
if (other._ipAddress != null) {
h2 = other._ipAddress.hashCode();
}
rc = h1 < h2 ? -1 : (h1 > h2 ? 1 : 0);
return rc != 0 ? rc : (_port < other.getPort() ? -1 : (_port > other.getPort() ? 1 : 0));
}
else if (_name != null) {
try {
InetAddress nameAddress= InetAddress.getByName(_name);
h1 = nameAddress.hashCode();
if (other._name != null) {
InetAddress otherAddress= InetAddress.getByName(other._name);
h2 = otherAddress.hashCode();
}
rc = h1 < h2 ? -1 : (h1 > h2 ? 1 : 0);
return rc != 0 ? rc : (_port < other.getPort() ? -1 : (_port > other.getPort() ? 1 : 0));
} catch (UnknownHostException e) {
return -1;
}
}
return _port < other.getPort() ? -1 : (_port > other.getPort() ? 1 : 0);
}
/**
* Compares the ServerInfo on the basis of priority
* @param obj The server info object to compare.
* @return 0 if equals, -1 if lesser and 1 if greater than the comparing serverInfo
*/
public final int compareTo(Object obj) {
int result = 0;
if (obj != null && obj instanceof ServerInfo) {
ServerInfo other = (ServerInfo) obj;
if (other.getPriorityInternal() > _priority) {
result = -1;
} else if (other.getPriorityInternal() < _priority) {
result = 1;
}
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy