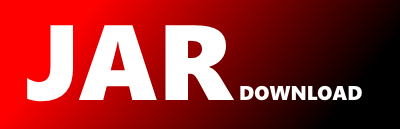
com.alachisoft.ncache.client.datastructures.CollectionManager Maven / Gradle / Ivy
package com.alachisoft.ncache.client.datastructures;
import com.alachisoft.ncache.runtime.caching.ReadThruOptions;
import com.alachisoft.ncache.runtime.caching.WriteThruOptions;
import com.alachisoft.ncache.runtime.exceptions.CacheException;
/**
* This interface contains Create and Get operations for all collection data types
*/
public interface CollectionManager {
/**
* Creates distributed list against the provided collection name.
* @param key Name of collection to be created.
* @param cls Class of the list items.
* @param Type of list items.
* @return Interface for using list.
* @throws CacheException
*/
DistributedList createList(String key,Class> cls) throws CacheException;
/**
* Creates distributed list against the provided collection name and configures it according to the provided user configuration as attributes of collection.
* @param key Name of collection to be created.
* @param attributes {@link DataStructureAttributes} for providing user configuration for this collection.
* @param options {@link WriteThruOptions} regarding updating the data source. This can be either WriteThru, WriteBehind or None.
* @param cls Class of the list items.
* @param Type of list items.
* @return Interface for using list.
* @throws CacheException
*/
DistributedList createList(String key, DataStructureAttributes attributes, WriteThruOptions options,Class> cls) throws CacheException;
/**
* Creates distributed queue against the provided collection name.
* @param key Name of collection to be created.
* @param cls Class of queue items.
* @param Type of queue items.
* @return Interface for using queue.
* @throws CacheException
*/
DistributedQueue createQueue(String key,Class> cls) throws CacheException;
/**
* Creates distributed queue against the provided collection name and configures it according to the provided user configuration as attributes of collection.
* @param key Name of collection to be created.
* @param attributes {@link DataStructureAttributes} for providing user configuration for this collection.
* @param options {@link WriteThruOptions} regarding updating the data source. This can be either WriteThru, WriteBehind or None.
* @param cls Class of queue items.
* @param Type of queue items.
* @return Interface for using queue.
* @throws CacheException
*/
DistributedQueue createQueue(String key, DataStructureAttributes attributes, WriteThruOptions options,Class> cls) throws CacheException;
/**
* Creates distributed Map against the provided collection name.
* @param key Name of collection to be created.
* @param cls Class of Map values.
* @param Type of Map values.
* @return Interface for using dictionary.
* @throws CacheException
*/
DistributedMap createMap(String key,Class> cls) throws CacheException;
/**
* Creates distributed Map against the provided collection name and configures it according to the provided user configuration as attributes of collection.
* @param key Name of collection to be created.
* @param attributes {@link DataStructureAttributes} for providing user configuration for this collection.
* @param options {@link WriteThruOptions} regarding updating the data source. This can be either WriteThru, WriteBehind or None.
* @param cls Class of Map values.
* @param Type of Map values.
* @return Interface for using dictionary.
* @throws CacheException
*/
DistributedMap createMap(String key, DataStructureAttributes attributes, WriteThruOptions options,Class> cls) throws CacheException;
/**
* Creates distributed set against the provided collection name.
* @param key Name of collection to be created.
* @param cls Class of set items.
* @param Type of set items.
* @return Interface for using set.
* @throws CacheException
*/
DistributedHashSet createHashSet(String key,Class> cls) throws CacheException;
/**
* Creates distributed set against the provided collection name and configures it according to the provided user configuration as attributes of collection.
* @param key Name of collection to be created.
* @param attributes {@link DataStructureAttributes} for providing user configuration for this collection.
* @param options {@link WriteThruOptions} regarding updating the data source. This can be either WriteThru, WriteBehind or None.
* @param cls Class of set items.
* @param Type of set items.
* @return Interface for using set.
* @throws CacheException
*/
DistributedHashSet createHashSet(String key, DataStructureAttributes attributes, WriteThruOptions options,Class> cls) throws CacheException;
/**
* Gets distributed list interface against the provided collection name.
* @param key Name of collection.
* @param cls Class of the list items.
* @param Type of list items.
* @return Interface for using list.
* @throws CacheException
*/
DistributedList getList(String key,Class> cls) throws CacheException;
/**
* Gets distributed list interface against the provided collection name.
* @param key Name of collection.
* @param options {@link ReadThruOptions} to read from data source. These can be either ReadThru, ReadThruForced or None.
* @param cls Class of the list items.
* @param Type of list items.
* @return Interface for using list.
* @throws CacheException
*/
DistributedList getList(String key, ReadThruOptions options,Class> cls) throws CacheException;
/**
* Gets distributed queue interface against the provided collection name.
* @param key Name of collection to be created.
* @param cls Class of queue items.
* @param Type of queue items.
* @return Interface for using queue.
* @throws CacheException
*/
DistributedQueue getQueue(String key,Class> cls) throws CacheException;
/**
* Gets distributed queue interface against the provided collection name.
* @param key Name of collection to be created.
* @param options {@link ReadThruOptions} to read from data source. These can be either ReadThru, ReadThruForced or None.
* @param cls Class of queue items.
* @param Type of queue items.
* @return Interface for using queue.
* @throws CacheException
*/
DistributedQueue getQueue(String key, ReadThruOptions options,Class> cls) throws CacheException;
/**
* Gets distributed Map interface against the provided collection name.
* @param key Name of collection.
* @param cls Class of Map values.
* @param Type of Map values.
* @return Interface for using Map.
* @throws CacheException
*/
DistributedMap getMap(String key,Class> cls) throws CacheException;
/**
* Gets distributed Map interface against the provided collection name.
* @param key Name of collection.
* @param options {@link ReadThruOptions} to read from data source. These can be either ReadThru, ReadThruForced or None.
* @param cls Class of Map values.
* @param Type of Map values.
* @return Interface for using Map.
* @throws CacheException
*/
DistributedMap getMap(String key, ReadThruOptions options,Class> cls) throws CacheException;
/**
* Gets distributed set interface against the provided collection name.
* @param key Name of collection.
* @param cls Class of set items.
* @param Type of set items.
* @return Interface for using set.
* @throws CacheException
*/
DistributedHashSet getHashSet(String key,Class> cls) throws CacheException;
/**
* Gets distributed set interface against the provided collection name.
* @param key Name of collection.
* @param options {@link ReadThruOptions} to read from data source. These can be either ReadThru, ReadThruForced or None.
* @param cls Class of set items.
* @param Type of set items.
* @return Interface for using set.
* @throws CacheException
*/
DistributedHashSet getHashSet(String key, ReadThruOptions options,Class> cls) throws CacheException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy